## Tutorial JQuery : More about Events ### What You Will Learn: • More Events (Bind/Unbind) • Depreciated Events ### Requirements: • Knowledge of HTML, CSS and JS. • Basics syntax of JQuery. • Basics of Events. ### Difficulty: • Basic. ## Tutorial Contents: After understanding mouse, keyboard and form events this tutorial covers bind, unbind and on/off event and which one is batter. Why two events perform same functionality? This question is answered in this tutorial. ``` <!DOCTYPE html> <html lang="en"> <head> <title>Events</title> <script src="jquery-3.2.1.js"></script> <script> $(document).ready(function(){ $('input').bind('select',function(){ $("input").css("background","yellow"); }); }); </script> </head> <body> <input type="text" value="tutorial"> </body> </html> ``` Run this code and after selecting something in the input field, the color of the text field will be yellow. As you can see in the picture below:  Bind event, as the name shows, is for combining the event and actions. You can see the example from the code above. Another alternate of bind is “on”. There is no difference between them. .on is batter because .bind will also convert itself into .on in backend so your site’s performance could be a little batter if you use .on. Another JQuery event is .one. It is for performing the functionality only once. To better understand this see the code below: ``` <!DOCTYPE html> <html lang="en"> <head> <title>Events</title> <script src="jquery-3.2.1.js"></script> <script> $(document).ready(function(){ $('input').bind('select',function(){ $(".para").fadeToggle(1000); }); }); </script> </head> <body> <p class="para">Hello World</p> <input type="text" value="tutorial"> </body> </html> ``` There is an input field and a paragraph in the HTML body. The code in the script means that whenever I will select any character in the input field the function will be triggered. Now Instead of the above given code if the function and events are combined using .one this means that the function will be triggered only once. You should try it yourself. Unbind is opposite of bind. Like bind combines the event and function, unbind is to remove event and function. To understand this lets see an example: ``` <!DOCTYPE html> <html lang="en"> <head> <title>Events</title> <script src="jquery-3.2.1.js"></script> <script> $(document).ready(function(){ $('p').click(function(){ $(this).fadeToggle(1000); }); }); </script> </head> <body> <p>Hello World</p> <p>I am Web Dev</p> </body> </html> ``` Above written code consists of two paragraphs and a simple JQuery click event. Now to unbind the event and the function I have added some more code. ``` <!DOCTYPE html> <html lang="en"> <head> <title>Events</title> <script src="jquery-3.2.1.js"></script> <script> $(document).ready(function(){ $('p').click(function(){ $(this).fadeToggle(1000); }); $("button").click(function(){ $("p").unbind(); }); }); </script> < /head> < body> < p>Hello World</p> < p>I am Web Dev</p> < button>Remove Event</button> < /body> < /html> ``` Run this code in the browser everything will see something like this  Everything will works as expected but because of unbind event, when you click on the function if you click on the paragraph nothing will happen. Another alternative of .unbind is .off. In near future bind/unbind will be depreciated from JQuery and on/off will replace them permanently. #### Curriculum - [Tutorial: selectors](https://utopian.io/utopian-io/@brothermic/tutorial-jquery-use-of-selectors) - [Tutorial: basic synthax](https://utopian.io/utopian-io/@brothermic/tutorial-basic-syntax-of-jquery) - [tutorial include jquery in code](https://utopian.io/utopian-io/@brothermic/tutorial-jquery-how-to-including-jquery-in-your-code) <br /><hr/><em>Posted on <a href="https://utopian.io/utopian-io/@brothermic/tutorial-jquery-more-about-events">Utopian.io - Rewarding Open Source Contributors</a></em><hr/>
post_id | 28,420,773 | ||||||
---|---|---|---|---|---|---|---|
author | brothermic | ||||||
permlink | tutorial-jquery-more-about-events | ||||||
category | utopian-io | ||||||
json_metadata | "{"repository": {"owner": {"login": "jquery"}, "id": 167174, "full_name": "jquery/jquery", "fork": false, "name": "jquery", "html_url": "https://github.com/jquery/jquery"}, "moderator": {"pending": false, "account": "amosbastian", "reviewed": true, "flagged": false, "time": "2018-01-27T17:19:55.744Z"}, "format": "markdown", "platform": "github", "tags": ["utopian-io", "jquery", "javascript", "html", "programming"], "community": "utopian", "type": "tutorials", "pullRequests": [], "links": ["https://res.cloudinary.com/hpiynhbhq/image/upload/v1517042355/p4tyyww1zvf8fsjvobju.png", "https://res.cloudinary.com/hpiynhbhq/image/upload/v1517042909/zxwzpgzhx9hcwxn5326i.png", "https://utopian.io/utopian-io/@brothermic/tutorial-jquery-use-of-selectors", "https://utopian.io/utopian-io/@brothermic/tutorial-basic-syntax-of-jquery", "https://utopian.io/utopian-io/@brothermic/tutorial-jquery-how-to-including-jquery-in-your-code"], "app": "utopian/1.0.0", "users": ["brothermic"], "image": ["https://res.cloudinary.com/hpiynhbhq/image/upload/v1517042355/p4tyyww1zvf8fsjvobju.png"]}" | ||||||
created | 2018-01-27 09:07:24 | ||||||
last_update | 2018-01-27 17:19:54 | ||||||
depth | 0 | ||||||
children | 12 | ||||||
net_rshares | 3,734,043,226,821 | ||||||
last_payout | 2018-02-03 09:07:24 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 18.520 SBD | ||||||
curator_payout_value | 7.654 SBD | ||||||
pending_payout_value | 0.000 SBD | ||||||
promoted | 0.000 SBD | ||||||
body_length | 4,475 | ||||||
author_reputation | 70,073,781,649,061 | ||||||
root_title | "Tutorial JQuery : More about Events" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 SBD | ||||||
percent_steem_dollars | 10,000 | ||||||
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
rsauerschnig | 0 | 4,240,141,431 | 100% | ||
droucil | 0 | 18,320,707,052 | 100% | ||
michaelwilshaw | 0 | 3,757,569,242 | 10% | ||
javio | 0 | 245,801,514 | 40% | ||
traderr | 0 | 7,456,338,331 | 100% | ||
simpleawesome | 0 | 614,503,071 | 100% | ||
jonas160 | 0 | 2,426,379,999 | 100% | ||
ricbo | 0 | 944,063,437 | 100% | ||
alanzheng | 0 | 54,092,391 | 2% | ||
utopian-io | 0 | 3,688,434,223,477 | 2.4% | ||
dbddv01 | 0 | 811,305,297 | 100% | ||
yorkchinese | 0 | 351,642,433 | 4.8% | ||
steemitstats | 0 | 2,061,751,003 | 5% | ||
bardionson | 0 | 512,662,900 | 100% | ||
maghrib | 0 | 497,434,152 | 100% | ||
foudmf103 | 0 | 518,901,259 | 100% | ||
claraquarius | 0 | 1,870,939,879 | 5% | ||
jauharialz | 0 | 390,187,257 | 100% | ||
nehaar | 0 | 534,582,696 | 100% |
@brothermic, I like your contribution to open source project, so I upvote to support you.
post_id | 28,421,212 |
---|---|
author | steemitstats |
permlink | 20180127t091023445z-post |
category | utopian-io |
json_metadata | "{"tags": ["utopian-io"]}" |
created | 2018-01-27 09:10:24 |
last_update | 2018-01-27 09:10:24 |
depth | 1 |
children | 1 |
net_rshares | 6,824,616,674 |
last_payout | 2018-02-03 09:10:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.056 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 89 |
author_reputation | 352,100,520,168 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 6,824,616,674 | 1% |
thanks alot!
post_id | 28,422,056 |
---|---|
author | brothermic |
permlink | re-steemitstats-20180127t091023445z-post-20180127t091602632z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-27 09:16:00 |
last_update | 2018-01-27 09:16:00 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-02-03 09:16:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 12 |
author_reputation | 70,073,781,649,061 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Very interesting and important tutorial you share with us.I want to learn javascript, programming etc..you must be good trainer this subject...
post_id | 28,421,583 |
---|---|
author | kawsar |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180127t091242063z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-27 09:12:48 |
last_update | 2018-01-27 09:12:48 |
depth | 1 |
children | 1 |
net_rshares | 7,425,408,850 |
last_payout | 2018-02-03 09:12:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.061 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 144 |
author_reputation | 140,532,825,392,812 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 6,824,616,674 | 1% | ||
rakeshsaha | 0 | 600,792,176 | 100% |
thanks alot!
post_id | 28,422,101 |
---|---|
author | brothermic |
permlink | re-kawsar-re-brothermic-tutorial-jquery-more-about-events-20180127t091623271z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-27 09:16:21 |
last_update | 2018-01-27 09:16:21 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-02-03 09:16:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 12 |
author_reputation | 70,073,781,649,061 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
very good and awesome tutorial
post_id | 28,433,190 |
---|---|
author | jauharialz |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180127t102700160z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-27 10:26:45 |
last_update | 2018-01-27 10:26:45 |
depth | 1 |
children | 0 |
net_rshares | 6,824,616,674 |
last_payout | 2018-02-03 10:26:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.042 SBD |
curator_payout_value | 0.014 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 30 |
author_reputation | 4,309,674,245,249 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 6,824,616,674 | 1% |
Thank you for the contribution. It has been approved. You can contact us on [Discord](https://discord.gg/uTyJkNm). **[[utopian-moderator]](https://utopian.io/moderators)**
post_id | 28,508,110 |
---|---|
author | amosbastian |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180127t171959417z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-27 17:20:00 |
last_update | 2018-01-27 17:20:00 |
depth | 1 |
children | 1 |
net_rshares | 20,473,850,022 |
last_payout | 2018-02-03 17:20:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.132 SBD |
curator_payout_value | 0.042 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 172 |
author_reputation | 174,225,255,912,876 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 20,473,850,022 | 3% |
thanks alot!
post_id | 28,541,052 |
---|---|
author | brothermic |
permlink | re-amosbastian-re-brothermic-tutorial-jquery-more-about-events-20180127t203455260z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-27 20:34:54 |
last_update | 2018-01-27 20:34:54 |
depth | 2 |
children | 0 |
net_rshares | 6,846,161,041 |
last_payout | 2018-02-03 20:34:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.041 SBD |
curator_payout_value | 0.013 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 12 |
author_reputation | 70,073,781,649,061 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 6,846,161,041 | 1% |
Goede tutorial Groetjes @brothermic
post_id | 28,557,236 |
---|---|
author | traderr |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180127t222119233z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["brothermic"], "tags": ["utopian-io"]}" |
created | 2018-01-27 22:21:18 |
last_update | 2018-01-27 22:21:18 |
depth | 1 |
children | 0 |
net_rshares | 7,361,524,652 |
last_payout | 2018-02-03 22:21:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.046 SBD |
curator_payout_value | 0.013 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 35 |
author_reputation | 1,490,504,643,386 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
traderr | 0 | 7,361,524,652 | 100% |
### Hey @brothermic I am @utopian-io. I have just upvoted you! #### Achievements - Seems like you contribute quite often. AMAZING! #### Suggestions - Contribute more often to get higher and higher rewards. I wish to see you often! - Work on your followers to increase the votes/rewards. I follow what humans do and my vote is mainly based on that. Good luck! #### Get Noticed! - Did you know project owners can manually vote with their own voting power or by voting power delegated to their projects? Ask the project owner to review your contributions! #### Community-Driven Witness! I am the first and only Steem Community-Driven Witness. <a href="https://discord.gg/zTrEMqB">Participate on Discord</a>. Lets GROW TOGETHER! - <a href="https://v2.steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1">Vote for my Witness With SteemConnect</a> - <a href="https://v2.steemconnect.com/sign/account-witness-proxy?proxy=utopian-io&approve=1">Proxy vote to Utopian Witness with SteemConnect</a> - Or vote/proxy on <a href="https://steemit.com/~witnesses">Steemit Witnesses</a> [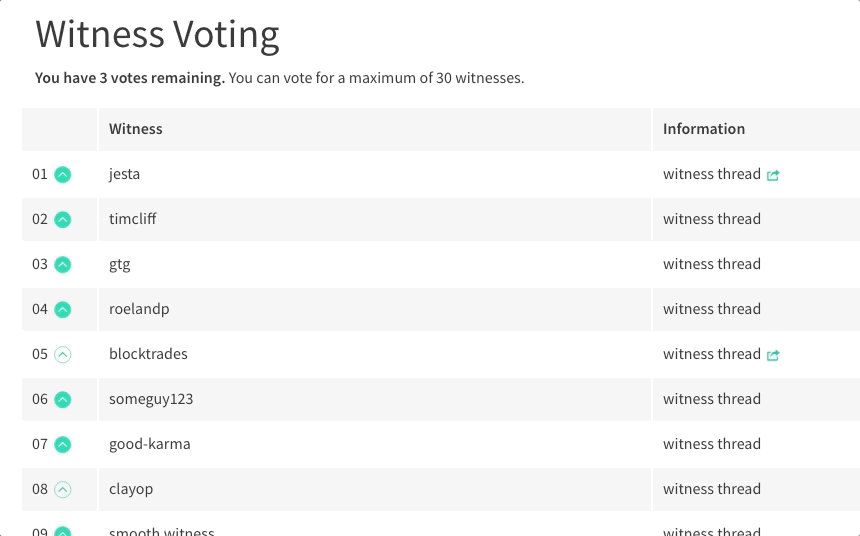](https://steemit.com/~witnesses) **Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x**
post_id | 28,744,042 |
---|---|
author | utopian-io |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180128t174627894z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-28 17:46:27 |
last_update | 2018-01-28 17:46:27 |
depth | 1 |
children | 1 |
net_rshares | 0 |
last_payout | 2018-02-04 17:46:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 1,430 |
author_reputation | 152,913,012,544,965 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
thanks!
post_id | 44,817,728 |
---|---|
author | brothermic |
permlink | re-utopian-io-re-brothermic-tutorial-jquery-more-about-events-20180422t105050498z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-04-22 10:50:51 |
last_update | 2018-04-22 10:50:51 |
depth | 2 |
children | 0 |
net_rshares | 59,288,898,596 |
last_payout | 2018-04-29 10:50:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.394 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 7 |
author_reputation | 70,073,781,649,061 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 59,288,898,596 | 100% |
Thanks for sharing brothermic. This is definatly interesting for a someone like me who just started learning the art of jquerry. Thanks to you
post_id | 28,976,331 |
---|---|
author | zoef |
permlink | re-brothermic-tutorial-jquery-more-about-events-20180129t163243118z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-29 16:32:42 |
last_update | 2018-01-29 16:32:42 |
depth | 1 |
children | 1 |
net_rshares | 610,420,582,827 |
last_payout | 2018-02-05 16:32:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 3.434 SBD |
curator_payout_value | 1.142 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 142 |
author_reputation | 23,263,050,671,536 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
zoef | 0 | 610,420,582,827 | 100% |
I love hearing that I helping you out :) thats why I do it for! :)
post_id | 29,039,299 |
---|---|
author | brothermic |
permlink | re-zoef-re-brothermic-tutorial-jquery-more-about-events-20180129t230231620z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-29 23:02:30 |
last_update | 2018-01-29 23:02:30 |
depth | 2 |
children | 0 |
net_rshares | 637,370,953,253 |
last_payout | 2018-02-05 23:02:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 3.516 SBD |
curator_payout_value | 1.169 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 66 |
author_reputation | 70,073,781,649,061 |
root_title | "Tutorial JQuery : More about Events" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
brothermic | 0 | 637,370,953,253 | 100% |