You spent a lot of time networking on Steemit, commenting, upvoting and trying to create your own little community. At the end of the day, you got a few hundreds (of thousands) followers. Nice. But how many of your readers are actually supporting you too? In other words, what's the _engagement rate_ between the total number of your followers and the total _votes_ you get on your posts? In today's tutorial we're going to write a short snippet using `php-steem-tools` and a little magic to get exactly that. I will describe the same approach used in the "Social Insights" section of [steem.supply](http://steem.supply), which generates the following chart: ***  *** # Prerequisites You will need basic understanding of PHP and composer. # Step 1: Add `php-steem-tools` to `composer.php` Install composer (this is out of the scope of this tutorial, but if you need a good start, the [Composer website](http://getcomposer.org/) has a very good documentation). Open composer.php and add the following lines: ``` { "minimum-stability" : "dev", "require": { "dragos-roua/php-steem-tools": "v0.0.4-alpha" } } ``` The current version of the package is 0.0.4-alpha, and you need the "minimum-stability" setting to be at the "dev" level. # Step 2: Require the package and instantiate the Steem API Create a file (in the same folder as composer.php) and require the package: ```require __DIR__ . '/vendor/autoload.php';``` Now you'll have access to all the classes you included in the composer.php file. Let's instantiate the API. Add the following lines: ``` $config['webservice_url'] = 'steemd.minnowsupportproject.org'; $api = new DragosRoua\PHPSteemTools\SteemApi($config); ``` Now, the `$api` variable will contain a new API object which will query the data from the `webservice_url` address. If you want to query a different node, just change this address. # Step 3: Pick an author and get the total number of the followers If you read my [previous php-steem-tools tutorial](https://steemit.com/utopian-io/@dragosroua/how-to-get-the-payment-rewards-of-a-steemit-user-in-less-than-50-lines), you noticed that the first two steps are pretty much identical. Here's wehre we change things a bit. We are going to find the total number of followers of the author. This is a recursive call, hence resource intensive, so we're going to use a little folder-based cache. Here's the code: ``` $follower_count = $api->getFollowerCount($account); $returned_follower_count = $follower_count['followers']; $cache_folder = "./"; $file_name = $cache_folder.$author.".txt"; $cache_interval = 86400; // seconds for the cache file to live if(file_exists($file_name)){ // if the file was modified less than $cache_interval, we do nothing $current_time = time(); if($current_time - filemtime($file_name) > $cache_interval){ $follower_count = $api->getFollowerCount($author); // write to the file again $handle = fopen($file_name, 'w+') or die('Cannot open file: '.$file_name); fwrite($handle, "followers|".$follower_count); fclose($handle); $returned_follower_count = $follower_count; } else { // get the data from the cache file $cache_contents = file($file_name); $first_line = $cache_contents[0]; $content = explode("|", $first_line); $returned_follower_count = $content[1]; } } else { $follower_count = $api->getFollowerCount($author); // write the data to cache file $handle = fopen($file_name, 'w+') or die('Cannot open file: '.$file_name); fwrite($handle, "followers|".$follower_count); fclose($handle); $returned_follower_count = $follower_count; } ``` It's pretty much self-explanatory, not much going on here. We make a call to the `getFollowerCount()` of the API and save the result in txt file. The variable `$follower_count` will hold the returned value from the API call. # Step 4: Get the total number of posts and the votes The total number of posts is not really required for our goal, but it's a nice addition. Again, if you looked at my previous tutorial, you'll notice some similarities. What we are going to do is to call the `getDiscussionsByAuthorBeforeDate($params, $transport)` function of the API, which will return the total number of posts during the last 8 days. If we have a result, we then cycle through the returned JSON object and the following: * increment the value of the variable `$total_posts` * add to the array `$votes_number` the voter nickname as key, and total number of his votes as the value, because we are going to use the total number of users who are actually voting * at the end of the loop, we just print the calculated ratio Here's the code: ``` date_default_timezone_set('UTC'); $dateNow = (new \DateTime())->format('Y-m-d\TH:i:s'); $date_8days_ago = date('Y-m-d\TH:i:s', strtotime('-8 days', strtotime($dateNow))); $params = [$author, '', $date_8days_ago, 100]; $remote_content = $api->getDiscussionsByAuthorBeforeDate($params, 'websocket'); //print_r($remote_content); if(isset($remote_content)){ if (array_key_exists('error', $remote_content)){ echo "Something is not ok. You should investigate more.\n"; } else { $votes_number = array(); $total_posts = 0; foreach($remote_content as $rkey => $rvalue){ if($rvalue['pending_payout_value'] !== '0.000 SBD' && $rvalue['max_accepted_payout'] != 0){ foreach($rvalue['active_votes'] as $idx => $voter){ $voter_nickname = $voter['voter']; if($voter_nickname != $author){ if(array_key_exists($voter['voter'], $votes_number)){ $votes_number[$voter['voter']] += 1; } else { $votes_number[$voter['voter']] = 1; } } } } $total_posts++; } echo "\n**************\n"; echo "Total number of posts during the last 7 days: ".$total_posts."\n"; echo "Total number of voters: ".count($votes_number)." \n"; echo "Total followers: ".$returned_follower_count."\n"; echo "**************\n"; echo "Conversion rate: ".number_format((count($votes_number) * 100)/$returned_follower_count, 2)."%\n**************\n"; } } ``` That's pretty much it! Now you can invoke the script (in the same folder) by issuing this command: `php conversionRate.php`. And here's how it looks for the author `utopian-io`: ***  *** `utopian-io` has a 10.69% engagement rate. You can get the full source of this tutorial from the 'examples' folder of the [php-steem-tools repository](https://github.com/dragosroua/php-steem-tools) on GitHub. _Note: this snippet is calculating the ratio between your followers and the number of votes you get, resulting in an overview of your popularity. It doesn't calculate how many of your followers are voting you. I leave this as a homework for you. Hint: there is a function in the api called `getFollowers($account, $transport)`, which returns an array. You may want to intersect that array with the `$votes_number` array created in the script above, and get the percentage of the voting followers._ *** _I'm a serial entrepreneur, blogger and ultrarunner. You can find me mainly on my blog at [Dragos Roua](http://dragosroua.com) where I write about productivity, business, relationships and running. Here on Steemit you may stay updated by following me @dragosroua._ <center> </center> _If you're new to Steemit, you may find these articles relevant (that's also part of my witness activity to support new members of the platform)_: * [Rewards: Were Does The Money Come From?](https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-rewards-were-does-the-money-come-from) * [Netiquette: How To Avoid Being A Steem Douche?](https://steemit.com/steem/@dragosroua/steemit-newbie-faq-netiquette-how-to-avoid-being-a-steem-douche) * [Witnesses: What Are They And Why Should You Care?](https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-witnesses-what-are-they-and-why-should-you-care) * [How Can I Mine Steem And What CPU / GPU Power Do I Need For That?](https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-how-can-i-mine-steem-and-what-cpu-gpu-power-do-i-need-for-that) * [The Beginner Guide To Not Getting Hacked On Steemit](https://steemit.com/steemit/@dragosroua/the-beginner-guide-to-not-getting-hacked-on-steemit) <br /><hr/><em>Posted on <a href="https://utopian.io/utopian-io/@dragosroua/how-to-calculate-your-engagement-rate-using-php-steem-tools">Utopian.io - Rewarding Open Source Contributors</a></em><hr/>
post_id | 23,258,367 | ||||||
---|---|---|---|---|---|---|---|
author | dragosroua | ||||||
permlink | how-to-calculate-your-engagement-rate-using-php-steem-tools | ||||||
category | utopian-io | ||||||
json_metadata | "{"repository": {"id": 104924789, "watchers": 3, "events_url": "https://api.github.com/repos/dragosroua/php-steem-tools/events", "forks": 2, "name": "php-steem-tools", "issues_url": "https://api.github.com/repos/dragosroua/php-steem-tools/issues{/number}", "trees_url": "https://api.github.com/repos/dragosroua/php-steem-tools/git/trees{/sha}", "fork": false, "git_url": "git://github.com/dragosroua/php-steem-tools.git", "assignees_url": "https://api.github.com/repos/dragosroua/php-steem-tools/assignees{/user}", "size": 491, "owner": {"id": 1648447, "following_url": "https://api.github.com/users/dragosroua/following{/other_user}", "starred_url": "https://api.github.com/users/dragosroua/starred{/owner}{/repo}", "subscriptions_url": "https://api.github.com/users/dragosroua/subscriptions", "repos_url": "https://api.github.com/users/dragosroua/repos", "login": "dragosroua", "gists_url": "https://api.github.com/users/dragosroua/gists{/gist_id}", "followers_url": "https://api.github.com/users/dragosroua/followers", "received_events_url": "https://api.github.com/users/dragosroua/received_events", "type": "User", "avatar_url": "https://avatars0.githubusercontent.com/u/1648447?v=4", "site_admin": false, "organizations_url": "https://api.github.com/users/dragosroua/orgs", "gravatar_id": "", "events_url": "https://api.github.com/users/dragosroua/events{/privacy}", "url": "https://api.github.com/users/dragosroua", "html_url": "https://github.com/dragosroua"}, "forks_count": 2, "git_refs_url": "https://api.github.com/repos/dragosroua/php-steem-tools/git/refs{/sha}", "blobs_url": "https://api.github.com/repos/dragosroua/php-steem-tools/git/blobs{/sha}", "pushed_at": "2018-01-02T16:04:00Z", "watchers_count": 3, "teams_url": "https://api.github.com/repos/dragosroua/php-steem-tools/teams", "comments_url": "https://api.github.com/repos/dragosroua/php-steem-tools/comments{/number}", "archived": false, "svn_url": "https://github.com/dragosroua/php-steem-tools", "merges_url": "https://api.github.com/repos/dragosroua/php-steem-tools/merges", "subscribers_url": "https://api.github.com/repos/dragosroua/php-steem-tools/subscribers", "issue_events_url": "https://api.github.com/repos/dragosroua/php-steem-tools/issues/events{/number}", "stargazers_url": "https://api.github.com/repos/dragosroua/php-steem-tools/stargazers", "mirror_url": null, "statuses_url": "https://api.github.com/repos/dragosroua/php-steem-tools/statuses/{sha}", "has_projects": true, "milestones_url": "https://api.github.com/repos/dragosroua/php-steem-tools/milestones{/number}", "description": "A collection of PHP tools to interact with the Steem blockchain.", "keys_url": "https://api.github.com/repos/dragosroua/php-steem-tools/keys{/key_id}", "open_issues": 0, "compare_url": "https://api.github.com/repos/dragosroua/php-steem-tools/compare/{base}...{head}", "ssh_url": "git@github.com:dragosroua/php-steem-tools.git", "license": {"name": "Other", "key": "other", "url": null, "spdx_id": null}, "html_url": "https://github.com/dragosroua/php-steem-tools", "commits_url": "https://api.github.com/repos/dragosroua/php-steem-tools/commits{/sha}", "open_issues_count": 0, "stargazers_count": 3, "branches_url": "https://api.github.com/repos/dragosroua/php-steem-tools/branches{/branch}", "full_name": "dragosroua/php-steem-tools", "forks_url": "https://api.github.com/repos/dragosroua/php-steem-tools/forks", "score": 23.845394, "deployments_url": "https://api.github.com/repos/dragosroua/php-steem-tools/deployments", "contributors_url": "https://api.github.com/repos/dragosroua/php-steem-tools/contributors", "homepage": null, "contents_url": "https://api.github.com/repos/dragosroua/php-steem-tools/contents/{+path}", "has_downloads": true, "collaborators_url": "https://api.github.com/repos/dragosroua/php-steem-tools/collaborators{/collaborator}", "created_at": "2017-09-26T18:47:42Z", "git_commits_url": "https://api.github.com/repos/dragosroua/php-steem-tools/git/commits{/sha}", "releases_url": "https://api.github.com/repos/dragosroua/php-steem-tools/releases{/id}", "private": false, "pulls_url": "https://api.github.com/repos/dragosroua/php-steem-tools/pulls{/number}", "git_tags_url": "https://api.github.com/repos/dragosroua/php-steem-tools/git/tags{/sha}", "notifications_url": "https://api.github.com/repos/dragosroua/php-steem-tools/notifications{?since,all,participating}", "language": "PHP", "updated_at": "2017-10-30T22:31:43Z", "has_wiki": true, "downloads_url": "https://api.github.com/repos/dragosroua/php-steem-tools/downloads", "hooks_url": "https://api.github.com/repos/dragosroua/php-steem-tools/hooks", "languages_url": "https://api.github.com/repos/dragosroua/php-steem-tools/languages", "default_branch": "master", "labels_url": "https://api.github.com/repos/dragosroua/php-steem-tools/labels{/name}", "url": "https://api.github.com/repos/dragosroua/php-steem-tools", "has_pages": false, "tags_url": "https://api.github.com/repos/dragosroua/php-steem-tools/tags", "clone_url": "https://github.com/dragosroua/php-steem-tools.git", "archive_url": "https://api.github.com/repos/dragosroua/php-steem-tools/{archive_format}{/ref}", "has_issues": true, "issue_comment_url": "https://api.github.com/repos/dragosroua/php-steem-tools/issues/comments{/number}", "subscription_url": "https://api.github.com/repos/dragosroua/php-steem-tools/subscription"}, "moderator": {"flagged": false, "account": "damla", "reviewed": true, "pending": false}, "format": "markdown", "platform": "github", "tags": ["utopian-io", "steemit", "php", "tutorial", "programming"], "community": "utopian", "type": "tutorials", "pullRequests": [], "links": ["http://steem.supply", "http://getcomposer.org/", "https://steemit.com/utopian-io/@dragosroua/how-to-get-the-payment-rewards-of-a-steemit-user-in-less-than-50-lines", "https://github.com/dragosroua/php-steem-tools", "http://dragosroua.com", "https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-rewards-were-does-the-money-come-from", "https://steemit.com/steem/@dragosroua/steemit-newbie-faq-netiquette-how-to-avoid-being-a-steem-douche", "https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-witnesses-what-are-they-and-why-should-you-care", "https://steemit.com/steemit/@dragosroua/steemit-newbie-faq-how-can-i-mine-steem-and-what-cpu-gpu-power-do-i-need-for-that", "https://steemit.com/steemit/@dragosroua/the-beginner-guide-to-not-getting-hacked-on-steemit", "https://utopian.io/utopian-io/@dragosroua/how-to-calculate-your-engagement-rate-using-php-steem-tools"], "app": "utopian/1.0.0", "users": ["dragosroua"], "image": ["https://res.cloudinary.com/hpiynhbhq/image/upload/v1514963595/ef0tgknljun1rjhsi2zc.png"]}" | ||||||
created | 2018-01-03 07:43:09 | ||||||
last_update | 2018-01-05 05:40:12 | ||||||
depth | 0 | ||||||
children | 38 | ||||||
net_rshares | 6,866,864,711,104 | ||||||
last_payout | 2018-01-10 07:43:09 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 48.410 SBD | ||||||
curator_payout_value | 15.308 SBD | ||||||
pending_payout_value | 0.000 SBD | ||||||
promoted | 0.000 SBD | ||||||
body_length | 8,787 | ||||||
author_reputation | 283,284,114,896,802 | ||||||
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 SBD | ||||||
percent_steem_dollars | 10,000 | ||||||
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
sandra | 0 | 13,472,937,121 | 13% | ||
bue | 0 | 27,618,205,140 | 100% | ||
jason | 0 | 16,963,447,174 | 13% | ||
nanzo-scoop | 0 | 40,957,390,078 | 1% | ||
mummyimperfect | 0 | 418,276,998 | 1% | ||
scalextrix | 0 | 24,596,700,566 | 100% | ||
ak2020 | 0 | 247,808,721 | 1% | ||
ausbitbank | 0 | 164,768,722,334 | 1% | ||
vortac | 0 | 704,067,654,322 | 20% | ||
deanliu | 0 | 899,733,338,761 | 70% | ||
kenjiby | 0 | 4,612,033,484 | 60% | ||
kaykunoichi | 0 | 13,654,259,466 | 53% | ||
hms818 | 0 | 2,643,380,238 | 5% | ||
sc-steemit | 0 | 11,311,120,432 | 15% | ||
moisesmcardona | 0 | 4,229,779,408 | 5% | ||
stokjockey | 0 | 24,342,163,786 | 50% | ||
immarojas | 0 | 137,726,246,106 | 49% | ||
kyusho | 0 | 9,384,681,708 | 5% | ||
dgiors | 0 | 48,668,413,078 | 30% | ||
ivarlan | 0 | 386,637,577 | 100% | ||
crowdfundedwhale | 0 | 50,135,700,450 | 12% | ||
teamhumble | 0 | 53,239,473,159 | 8% | ||
mafeeva | 0 | 566,581,046 | 1% | ||
osmanbugra | 0 | 12,848,321,334 | 100% | ||
alinamarin | 0 | 7,007,920,137 | 100% | ||
alexvan | 0 | 2,218,751,391 | 5% | ||
newhope | 0 | 1,813,508,306,120 | 23% | ||
anonimnotoriu | 0 | 624,375,112 | 2% | ||
teammo | 0 | 105,762,227,480 | 50% | ||
oneshot | 0 | 3,656,151,642 | 100% | ||
choogirl | 0 | 27,930,136,028 | 3% | ||
andersokohler | 0 | 2,724,554,809 | 100% | ||
practicaleric | 0 | 2,376,515,070 | 100% | ||
solarcoach | 0 | 1,067,594,112 | 100% | ||
costopher | 0 | 8,098,970,419 | 30% | ||
cecicastor | 0 | 34,738,762,073 | 30% | ||
amazonpricecheck | 0 | 5,730,261,328 | 70% | ||
teutorigos | 0 | 3,172,446,750 | 100% | ||
nadieyja | 0 | 96,395,574 | 10% | ||
zneeke | 0 | 2,916,266,789 | 25% | ||
cryptochallenge | 0 | 5,741,764,884 | 100% | ||
jmourad | 0 | 89,547,624 | 20% | ||
bubke | 0 | 207,550,324,677 | 100% | ||
thinknzombie | 0 | 2,332,630,278 | 1% | ||
theguruasia | 0 | 192,826,218 | 100% | ||
blazing | 0 | 894,456,007 | 100% | ||
barteksiama | 0 | 3,226,642,957 | 100% | ||
dailygrcstats | 0 | 172,650,855 | 5% | ||
embalsespr | 0 | 53,416,619 | 5% | ||
duekie | 0 | 68,808,653 | 65.77% | ||
santigs | 0 | 548,246,697 | 100% | ||
kromtar | 0 | 5,566,882,621 | 50% | ||
nachon | 0 | 547,010,039 | 100% | ||
trailhispano | 0 | 54,255,304 | 5% | ||
mystic-natura | 0 | 108,806,831 | 20% | ||
americanrabbit | 0 | 256,717,193 | 50% | ||
delph1 | 0 | 618,039,701 | 100% | ||
calatorulmiop | 0 | 3,695,344,452 | 20% | ||
moni.sagenscheid | 0 | 603,553,008 | 100% | ||
fritz.langen | 0 | 605,837,123 | 100% | ||
julia.mueller | 0 | 599,937,000 | 100% | ||
qaismx | 0 | 5,977,239,137 | 20% | ||
cliffy32 | 0 | 543,257,283 | 100% | ||
outwalking | 0 | 269,806,029 | 20% | ||
melanie.fischer | 0 | 605,636,758 | 100% | ||
sandra.weber | 0 | 607,840,092 | 100% | ||
anja.meyer | 0 | 605,316,933 | 100% | ||
steemclaira | 0 | 143,381,168 | 100% | ||
nicole.wagner | 0 | 599,937,000 | 100% | ||
three.colours | 0 | 52,302,200 | 12% | ||
nadine.becker | 0 | 606,078,925 | 100% | ||
sabrina.hoffmann | 0 | 603,873,241 | 100% | ||
christina.schulz | 0 | 600,016,975 | 100% | ||
daniela.schaefer | 0 | 602,952,455 | 100% | ||
anna.koch | 0 | 600,496,884 | 100% | ||
kath.richter | 0 | 606,479,288 | 100% | ||
bienvenida | 0 | 66,259,165 | 5% | ||
drishitmitra | 0 | 952,561,631 | 100% | ||
katja.schroeder | 0 | 605,958,092 | 100% | ||
nina.wolf | 0 | 601,789,500 | 100% | ||
claudia.klein | 0 | 600,136,922 | 100% | ||
mdf-365 | 0 | 2,282,603,391 | 100% | ||
utopian-io | 0 | 2,309,654,481,929 | 1.32% | ||
tony10 | 0 | 3,219,702,905 | 11% | ||
amigoos | 0 | 52,302,200 | 10% | ||
ccworld | 0 | 55,282,513 | 100% | ||
rohit12123 | 0 | 115,141,366 | 100% | ||
mjhiggs16 | 0 | 631,212,835 | 100% | ||
akandadelwar | 0 | 169,550,459 | 15% | ||
superwhale | 0 | 522,050,102 | 100% | ||
raihan12123 | 0 | 189,272,006 | 100% | ||
beatenegg | 0 | 57,587,600 | 100% | ||
mstafford | 0 | 671,569,899 | 100% | ||
vigneshwar66 | 0 | 240,566,180 | 100% | ||
gwys | 0 | 465,430,912 | 100% | ||
nexit | 0 | 122,638,894 | 100% | ||
fonsetucker | 0 | 91,581,464 | 0.2% | ||
oguzhangazi | 0 | 649,697,661 | 100% | ||
lauriki | 0 | 1,958,598,654 | 100% | ||
sfeos | 0 | 4,457,962,557 | 100% | ||
cryptobokster | 0 | 1,125,684,333 | 100% | ||
azwargolden | 0 | 272,715,483 | 100% | ||
gujjar | 0 | 1,137,279,688 | 100% | ||
saft | 0 | 1,119,872,333 | 100% |
I will try this after my comment on your post has went through.. Am glad steemit is growing higher since the beginning of this year
post_id | 23,258,574 |
---|---|
author | harbecity |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t074435561z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 07:44:39 |
last_update | 2018-01-03 07:44:39 |
depth | 1 |
children | 0 |
net_rshares | 16,421,936,062 |
last_payout | 2018-01-10 07:44:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.188 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 131 |
author_reputation | 433,178,274,150 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,421,936,062 | 5% |
That's pretty neat. Can come in handy, gonna check it out asap,
post_id | 23,258,732 |
---|---|
author | barteksiama |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t074551328z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 07:45:57 |
last_update | 2018-01-03 07:45:57 |
depth | 1 |
children | 0 |
net_rshares | 21,344,935,863 |
last_payout | 2018-01-10 07:45:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.244 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 63 |
author_reputation | 1,119,151,519,991 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,421,936,062 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% | ||
barteksiama | 0 | 2,615,768,194 | 100% | ||
devilisinme | 0 | 1,143,076,721 | 100% |
"Social Insights" is an amazing tool of steem.supply ...Thanks @dragosroua for sharing these programming details....
post_id | 23,258,848 |
---|---|
author | hms818 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t074655042z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io"]}" |
created | 2018-01-03 07:46:57 |
last_update | 2018-01-03 07:46:57 |
depth | 1 |
children | 0 |
net_rshares | 54,357,146,354 |
last_payout | 2018-01-10 07:46:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.566 SBD |
curator_payout_value | 0.061 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 116 |
author_reputation | 11,510,948,505,908 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
hms818 | 0 | 36,771,055,406 | 100% | ||
dragosroua | 0 | 16,421,936,062 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Thanks so much for posting more details this tool. I actually really enjoy using all the different features of steem.supply.
post_id | 23,260,215 |
---|---|
author | rockjon |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t075747192z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 07:57:48 |
last_update | 2018-01-03 07:57:48 |
depth | 1 |
children | 0 |
net_rshares | 17,586,111,232 |
last_payout | 2018-01-10 07:57:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.170 SBD |
curator_payout_value | 0.030 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 124 |
author_reputation | 9,822,504,178,255 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,421,956,346 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Great work @dragosroua, going to try it as fast I can
post_id | 23,262,899 |
---|---|
author | fastfingers |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t081802241z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io"]}" |
created | 2018-01-03 08:18:18 |
last_update | 2018-01-03 08:18:18 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 08:18:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 53 |
author_reputation | 362,150,333,012 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
I'll definitely check out using this amazing feature at steem.supply. I wasn't aware of it before.
post_id | 23,263,076 |
---|---|
author | xalos |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t081938789z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:19:42 |
last_update | 2018-01-03 08:19:42 |
depth | 1 |
children | 0 |
net_rshares | 17,586,181,306 |
last_payout | 2018-01-10 08:19:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.194 SBD |
curator_payout_value | 0.006 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 99 |
author_reputation | 27,192,206,173,573 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,422,026,420 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
great post. Code goes so far over my head I really tried to learn it but just can't rap my brain around actually understanding it. Great tutorial here!
post_id | 23,263,493 |
---|---|
author | mjhiggs16 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t082259837z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:22:51 |
last_update | 2018-01-03 08:22:51 |
depth | 1 |
children | 0 |
net_rshares | 622,195,509 |
last_payout | 2018-01-10 08:22:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 153 |
author_reputation | 1,230,268,770 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
mjhiggs16 | 0 | 622,195,509 | 100% |
thanks for post
post_id | 23,263,497 |
---|---|
author | mhmtdr0 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t082252515z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:22:54 |
last_update | 2018-01-03 08:22:54 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 08:22:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 15 |
author_reputation | 4,582,591,140,074 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Thank you so much for this tool. i love the features of steem
post_id | 23,263,722 |
---|---|
author | sourovafrin |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t082432453z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:24:33 |
last_update | 2018-01-03 08:24:33 |
depth | 1 |
children | 0 |
net_rshares | 18,160,992,552 |
last_payout | 2018-01-10 08:24:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.206 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 62 |
author_reputation | 186,685,725,170,609 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,422,026,420 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% | ||
sourovafrin | 0 | 574,811,246 | 100% |
Very helpul tool for steem rate & calculative post!
post_id | 23,264,286 |
---|---|
author | jerge |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t082827959z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:28:51 |
last_update | 2018-01-03 08:28:51 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 08:28:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 52 |
author_reputation | 8,170,003,116,977 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Good job @dragosroua. very helpful tips.
post_id | 23,264,430 |
---|---|
author | akandadelwar |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t083000166z |
category | utopian-io |
json_metadata | "{"app": "busy/2.2.0", "community": "busy", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:30:00 |
last_update | 2018-01-03 08:30:00 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 08:30:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 40 |
author_reputation | 3,449,671,794,381 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
post_id | 23,266,032 |
---|---|
author | qaismx |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t084141707z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:41:42 |
last_update | 2018-01-03 08:41:42 |
depth | 1 |
children | 0 |
net_rshares | 30,215,045,945 |
last_payout | 2018-01-10 08:41:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.346 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 48 |
author_reputation | 224,445,607,823 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
qaismx | 0 | 29,414,308,388 | 100% | ||
dang20 | 0 | 800,737,557 | 100% |
@dragosroua I love when you bring this to the table and show us this Great Stuff..........
post_id | 23,266,969 |
---|---|
author | stokjockey |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t084853136z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io"]}" |
created | 2018-01-03 08:49:00 |
last_update | 2018-01-03 08:49:00 |
depth | 1 |
children | 0 |
net_rshares | 24,888,835,974 |
last_payout | 2018-01-10 08:49:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.284 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 90 |
author_reputation | 120,226,443,461,741 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
stokjockey | 0 | 7,302,649,135 | 15% | ||
dragosroua | 0 | 16,422,031,953 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
This is an amzing tutorial which will definitely help me to understand #steemit in more innovative way. Big thanks to @dragosroua who is always there to help us Stay Blessed, Steem On!
post_id | 23,267,236 |
---|---|
author | salmanbukhari54 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t085045164z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io", "steemit"]}" |
created | 2018-01-03 08:50:45 |
last_update | 2018-01-03 08:50:45 |
depth | 1 |
children | 0 |
net_rshares | 17,586,186,839 |
last_payout | 2018-01-10 08:50:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.188 SBD |
curator_payout_value | 0.012 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 186 |
author_reputation | 36,215,033,301,240 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,422,031,953 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
This is interesting. Now my math and programming subjects back then now have a purpose in my life! :-P Just kidding! Thank you for sharing!!!
post_id | 23,267,843 |
---|---|
author | beatenegg |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t085514898z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:55:15 |
last_update | 2018-01-03 08:55:15 |
depth | 1 |
children | 0 |
net_rshares | 17,586,186,839 |
last_payout | 2018-01-10 08:55:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.194 SBD |
curator_payout_value | 0.006 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 143 |
author_reputation | 1,328,413,661,710 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,422,031,953 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
you always focus our attention on simple things that have a big impact..we always fail to notice them unless someone like you brings it for the discussion :)
post_id | 23,268,170 |
---|---|
author | steemclaira |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t085738241z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 08:57:30 |
last_update | 2018-01-03 08:57:30 |
depth | 1 |
children | 0 |
net_rshares | 17,586,186,839 |
last_payout | 2018-01-10 08:57:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.194 SBD |
curator_payout_value | 0.006 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 157 |
author_reputation | 667,489,509,314 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,422,031,953 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Very useful, but I use minnowbooster sometimes for an upvote and because they use other peoples account to vote it is difficult to see whats a real vote or a paid one.
post_id | 23,271,057 |
---|---|
author | tony10 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t091816573z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 09:18:15 |
last_update | 2018-01-03 09:18:15 |
depth | 1 |
children | 0 |
net_rshares | 29,759,539,871 |
last_payout | 2018-01-10 09:18:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.254 SBD |
curator_payout_value | 0.085 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 167 |
author_reputation | 2,966,348,839,177 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
tony10 | 0 | 29,759,539,871 | 100% |
Thanks for the info
post_id | 23,271,253 |
---|---|
author | nachon |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t091937012z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 09:19:42 |
last_update | 2018-01-03 09:19:42 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 09:19:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 19 |
author_reputation | 585,838,322,788 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
quite awesome this is thanks for sharing time to check engagement ratio :)
post_id | 23,280,125 |
---|---|
author | blazing |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t102211949z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 10:22:12 |
last_update | 2018-01-03 10:22:12 |
depth | 1 |
children | 0 |
net_rshares | 17,600,974,256 |
last_payout | 2018-01-10 10:22:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.187 SBD |
curator_payout_value | 0.012 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 74 |
author_reputation | 103,117,727,459,305 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,436,819,370 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Damn, this is very useful for keeping an eye on your engagement success, thank you very much for creating this informative post!
post_id | 23,281,264 |
---|---|
author | stochasticmind |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t103008710z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 10:30:09 |
last_update | 2018-01-03 10:30:09 |
depth | 1 |
children | 0 |
net_rshares | 16,436,819,370 |
last_payout | 2018-01-10 10:30:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.187 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 128 |
author_reputation | 271,227,257,933 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,436,819,370 | 5% |
Nice! I suspect 10% is actually pretty strong given the dead followers.
post_id | 23,281,466 |
---|---|
author | miniature-tiger |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t103143175z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 10:31:42 |
last_update | 2018-01-03 10:31:42 |
depth | 1 |
children | 1 |
net_rshares | 17,600,974,256 |
last_payout | 2018-01-10 10:31:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.200 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 71 |
author_reputation | 74,702,189,892,733 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,436,819,370 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Yes, anything over 10% is very good, especially if the number of followers is beyond a few thousands.
post_id | 23,281,849 |
---|---|
author | dragosroua |
permlink | re-miniature-tiger-re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t103427824z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 10:34:27 |
last_update | 2018-01-03 10:34:27 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 10:34:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 101 |
author_reputation | 283,284,114,896,802 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Good good... Pleace vote me
post_id | 23,289,920 |
---|---|
author | syarol |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t113132504z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 11:31:33 |
last_update | 2018-01-03 11:31:33 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 11:31:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 29 |
author_reputation | 31,461,380,686 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
It's very much important to know one's daily engagement. However, it's more important to know which chunk of the audience is proactively participating in social media so that you could *device* your next SM strategy accordingly. Having that being said, *php-steem-tools* is very good tool one should have for Steemit. Also, this seems to be the first one on Steem engagement, if I'm not wrong. Thanks @dragosroua. Definitely going to share with my audience and I hope they love it. Steem On!
post_id | 23,299,776 |
---|---|
author | ugetfunded |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t123859627z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io"]}" |
created | 2018-01-03 12:39:00 |
last_update | 2018-01-03 12:39:00 |
depth | 1 |
children | 0 |
net_rshares | 17,603,141,001 |
last_payout | 2018-01-10 12:39:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.194 SBD |
curator_payout_value | 0.006 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 496 |
author_reputation | 13,558,830,206,659 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,438,986,115 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
super nice code man. thanks for adding this feature! :)
post_id | 23,311,680 |
---|---|
author | teamhumble |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t135126336z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 13:51:27 |
last_update | 2018-01-03 13:51:27 |
depth | 1 |
children | 0 |
net_rshares | 17,610,341,963 |
last_payout | 2018-01-10 13:51:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.199 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 55 |
author_reputation | 302,768,794,114,468 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 16,446,187,077 | 5% | ||
raluca | 0 | 1,164,154,886 | 5% |
Helpful post..thanks for sharing it. Upvoted and resteemed
post_id | 23,316,593 |
---|---|
author | raihan12123 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t141749864z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 14:17:54 |
last_update | 2018-01-03 14:17:54 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-10 14:17:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 58 |
author_reputation | 106,878,140,422 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
its a very helpful.........../////////
post_id | 23,321,729 |
---|---|
author | faisal79 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180103t144440760z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 14:44:42 |
last_update | 2018-01-03 14:44:42 |
depth | 1 |
children | 0 |
net_rshares | 857,958,905 |
last_payout | 2018-01-10 14:44:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 38 |
author_reputation | 1,362,838,658,835 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
faisal79 | 0 | 857,958,905 | 100% |
super decent code man. a debt of gratitude is in order for including this element.Upvoted and resteemed
post_id | 23,324,396 |
---|---|
author | rohit12123 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t045414564z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-03 14:58:48 |
last_update | 2018-01-03 14:58:48 |
depth | 1 |
children | 0 |
net_rshares | 18,276,022,438 |
last_payout | 2018-01-10 14:58:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.192 SBD |
curator_payout_value | 0.012 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 103 |
author_reputation | 3,557,223,159,170 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 18,276,022,438 | 6% |
That's a usefull tool thanks @dragosroua
post_id | 23,424,368 |
---|---|
author | myeasin |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t013426144z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "users": ["dragosroua"], "tags": ["utopian-io"]}" |
created | 2018-01-04 01:34:33 |
last_update | 2018-01-04 01:34:33 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-11 01:34:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 40 |
author_reputation | 3,801,893,963,205 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Your contribution cannot be approved yet. See the [Utopian Rules](https://utopian.io/rules). - Contributors should not ask for Steem/Steemit related activities in their posts, such as upvotes, resteems and follows. Please edit your contribution to reapply for approval. You may edit your post [here](https://utopian.io/utopian-io/@dragosroua/how-to-calculate-your-engagement-rate-using-php-steem-tools), as shown below:  You can contact us on [Discord](https://discord.gg/UCvqCsx). **[[utopian-moderator]](https://utopian.io/moderators)**
post_id | 23,437,012 |
---|---|
author | damla |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t030645355z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-04 03:06:39 |
last_update | 2018-01-04 03:06:39 |
depth | 1 |
children | 3 |
net_rshares | 0 |
last_payout | 2018-01-11 03:06:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 635 |
author_reputation | 13,839,203,997,366 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Pardon me?
post_id | 23,598,595 |
---|---|
author | dragosroua |
permlink | re-damla-re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t204517595z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-04 20:45:18 |
last_update | 2018-01-04 20:45:18 |
depth | 2 |
children | 2 |
net_rshares | 0 |
last_payout | 2018-01-11 20:45:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 10 |
author_reputation | 283,284,114,896,802 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Please remove from your signature "You can also vote for me as witness here" to reapply for approval.
post_id | 23,648,558 |
---|---|
author | damla |
permlink | re-dragosroua-re-damla-re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180105t024434769z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-05 02:44:33 |
last_update | 2018-01-05 02:44:33 |
depth | 3 |
children | 1 |
net_rshares | 0 |
last_payout | 2018-01-12 02:44:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 101 |
author_reputation | 13,839,203,997,366 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Wow you are the first prorammer I've stubbled upon on steemit. I myself did a post on a redesign of steemit's website well pretty much a dashboard. You can check it out if you'd like. Great post btw. Do you use laravel?
post_id | 23,437,966 |
---|---|
author | dang20 |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t031310627z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-04 03:13:09 |
last_update | 2018-01-04 03:13:09 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-11 03:13:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 220 |
author_reputation | 4,222,362,886 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Thanks you so much for the contribution. this tool will be really useful :)
post_id | 23,473,613 |
---|---|
author | praful |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180104t072953738z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-04 07:29:39 |
last_update | 2018-01-04 07:31:09 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-11 07:29:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 75 |
author_reputation | 2,789,687,494,229 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Thank you for the contribution. It has been approved. You can contact us on [Discord](https://discord.gg/UCvqCsx). **[[utopian-moderator]](https://utopian.io/moderators)**
post_id | 23,674,237 |
---|---|
author | damla |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180105t054014677z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-05 05:40:21 |
last_update | 2018-01-05 05:40:21 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-12 05:40:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 172 |
author_reputation | 13,839,203,997,366 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
### Hey @dragosroua I am @utopian-io. I have just upvoted you! #### Achievements - WOW WOW WOW People loved what you did here. GREAT JOB! - You are generating more rewards than average for this category. Super!;) - Seems like you contribute quite often. AMAZING! #### Suggestions - Contribute more often to get higher and higher rewards. I wish to see you often! - Work on your followers to increase the votes/rewards. I follow what humans do and my vote is mainly based on that. Good luck! #### Get Noticed! - Did you know project owners can manually vote with their own voting power or by voting power delegated to their projects? Ask the project owner to review your contributions! #### Community-Driven Witness! I am the first and only Steem Community-Driven Witness. <a href="https://discord.gg/zTrEMqB">Participate on Discord</a>. Lets GROW TOGETHER! - <a href="https://v2.steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1">Vote for my Witness With SteemConnect</a> - <a href="https://v2.steemconnect.com/sign/account-witness-proxy?proxy=utopian-io&approve=1">Proxy vote to Utopian Witness with SteemConnect</a> - Or vote/proxy on <a href="https://steemit.com/~witnesses">Steemit Witnesses</a> [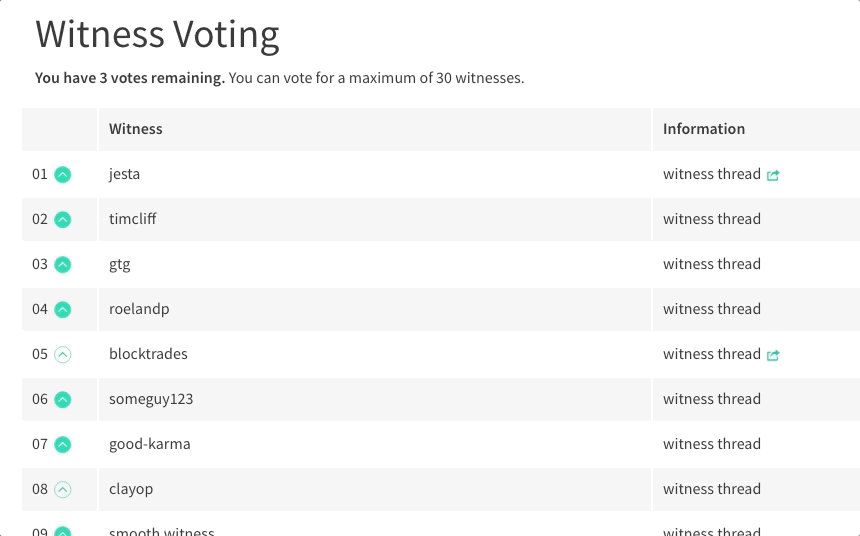](https://steemit.com/~witnesses) **Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x**
post_id | 23,804,329 |
---|---|
author | utopian-io |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180105t191639602z |
category | utopian-io |
json_metadata | "{"app": "utopian/1.0.0", "community": "utopian", "tags": ["utopian-io"]}" |
created | 2018-01-05 19:16:39 |
last_update | 2018-01-05 19:16:39 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-01-12 19:16:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 1,562 |
author_reputation | 152,913,012,544,965 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hello, Dragos! It's interesting to know how to calculate the engagement rate. Thanks for the codes. Have a nice day!
post_id | 24,144,755 |
---|---|
author | allesia |
permlink | re-dragosroua-how-to-calculate-your-engagement-rate-using-php-steem-tools-20180107t121439771z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-01-07 12:14:39 |
last_update | 2018-01-07 12:14:39 |
depth | 1 |
children | 0 |
net_rshares | 15,816,651,079 |
last_payout | 2018-01-14 12:14:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.155 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 117 |
author_reputation | 10,992,870,481,217 |
root_title | "How To Calculate Your Engagement Rate Using php-steem-tools" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
dragosroua | 0 | 14,898,741,797 | 4% | ||
raluca | 0 | 917,909,282 | 4% |