#### Repository
https://github.com/firebase
#### What Will I Learn?
- Form edit forum
- Update data forum
#### Requirements
- Basic Javascript
- Install Firebase
#### Resources
- Firebase-https://firebase.google.com/
#### Difficulty
Basic
### Tutorial Content
In this tutorial, I will continue the previous tutorial series. This tutorial I will use the edit form to edit the forum that we have created. with the system, we have created [before](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-10-authorized-domain-and-user-forum-ownership-1560338464527) we can ensure that the forum can only be edited by the owner of the forum. For those of you who are just following this tutorial, there are several series that you should follow first so that you don't have problems following this tutorial.
### Form edit forum
In this section we will use the form to edit the forum, here I will use ***bootstrap***. In bootstrap, I will use the form-input component. The following is the display of the interface:
**forum-details.hbs**
```
<div class="is-hidden">
<form>
<div class="form-group">
<label for="title">Title</label>
<input type="text" class="form-control" id="title" value="{{forum.title}}">
</div>
<div class="form-group">
<label for="desc">Desciptions</label>
<textarea class="form-control" id="desc" rows="3">{{forum.desc}}</textarea>
</div>
<button class="btn btn-info" type="button" name="button" onclick="editTopic()" id="update-btn">Update</button>
</form>
</div>
<script type="text/javascript" src="/assets/js/auth.js"></script>
</body>
<style type="text/css">
.is-hidden {
display: none;
}
</style>
```
We have passed the object ***forum*** section in the **forum-details.hbs** template. Now we can use it to fill in the edit form with the **value** of the ***forum*** object.
We will make an **input text** for the *title* ```<input type="text" class="form-control" id="title" value="{{forum.title}}">``` and the value will be taken from the ```forum.title``` object.
And then we will fill in the description with the textarea ``` <textarea class="form-control" id="desc" rows="3">{{forum.desc}}</textarea>```. WWe get the value from ```forum.desc```.
If you followed the previous tutorial you would know that we have a class that is used to hide elements from users who are not forum owners ```<div class="is-hidden">```.
- **Edit forum with ownership access**
We can see in the form we will make the element form hidden by default. We will change the situation if the user who is logged in is the user who owns the forum. We will add a ***onclick*** function to the edit button, for more details we can see in the code below:
**forum-details.hbs**
```
<h2>Forum {{forum.title}}</h2>
<p>{{forum.desc}} <span><i>by: <b>{{forum.user.username}}</b></i></span></p>
<input type="hidden" name="owner_uid" id="owner_id" value="{{forum.user.user_id}}">
<a href="#" class="btn btn-info is-hidden" id="btn-edit" onclick="showEditForm()">Edit</a>
```
The function that I will use above is ```showEditForm ()```. We will use this function to display the edit form. now we will make the function like the code below.
```
<script type="text/javascript">
function showEditForm() {
document.getElementById('editForm').classList.remove('is-hidden')
}
</script>
```
The function above is quite simple, we will only remove the ***is-hidden*** *class* in the edit form element. **editForm** is an ***id*** from form edit element, we can give the id like this:
```
<div class="is-hidden" id="editForm"></div>
```
We can see the results from the form edit interface as shown below:

We can see in the picture above, the input from the form ***has been filled*** in from the object forum that we have passed in the **forum-details.hbs** template.
The edit button only ***appears*** in the owner of the forum, so other users ***can not edit*** the forum that is not theirs, as we can see in the demonstration below:
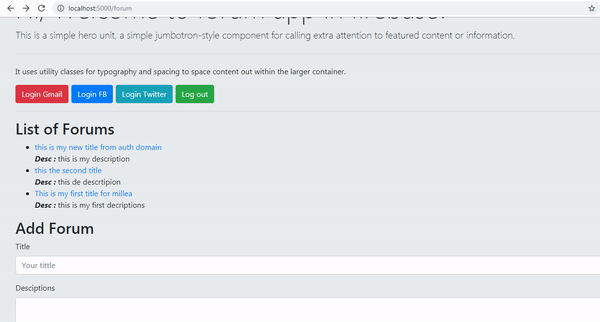
You can see in the picture above there is a forum that cannot bring up the edit button because the forum is not his. until here our forum ownership system has been successful.
### Update data forum
We have created a form to edit the data, and then we will learn how to update data in the application of our Firebase forum. We have created a form to edit the data. For those of you who are just following this tutorial for information that in this tutorial we use Firestore as the database if you don't know what firestore is you can see my previous tutorial in the **curriculum** section.
<br>
- **Create new rules for update data**
We will start to make the data update feature by creating new rules on our Firestore database, here we will create a rule for updates, here is an example:
```
service cloud.firestore {
match /databases/{database}/documents {
match /users/{document=**} {
allow read, create: if request.auth != null;
}
match /forums/{slug} {
allow read;
allow create: if request.auth != null
&& request.resource.data.title.size() > 10
allow update: if request.auth.uid == resource.data.user.user_id
&& request.resource.data.title.size() > 10
}
}
}
```

Here I add for the update section, in the update section we only allow updates when ```request.auth.uid == resource.data.user.user_id```. This means that the user ID must be the same as user_id in the Firestore database because we will guarantee that the person who can edit the forum is the owner of the forum.
<br>
- **Update data forum**
Now that we have completed the rules in the database, now we will update the data in the forum. Forum data stored in the database is in a document and the name of the document is the name that was automatically generated by ***Firebase***. For more details, we can see in the picture below:

We need the **document ID** to update the data in the document. * than where can we get the ID?*
We can get the id when we draw the *single forum* data at the ```app.getURL('/ forum /: slug', function (req, res) { }```.
**index.js**
```
db.collection('forums').where('slug', '==', req.params.slug).get()
.then(snapshot => {
snapshot.forEach(doc => {
forum = doc.data()
console.log(doc.id)
})
res.render('forum-detail', {forum:forum})
}).catch(err => {
console.log(err)
})
```
I will do a ```console log()``` on doc which is the response we received when pulling single forum data.

We see in the picture above we managed to get the document ID we need. We can get the id on the key id on the doc object. now *we will pass the **ID** to the **frontend***.
**index.js**
```
db.collection('forums').where('slug', '==', req.params.slug).get()
.then(snapshot => {
snapshot.forEach(doc => {
forum = doc.data()
forum.id = doc.id
})
res.render('forum-detail', {forum:forum})
})
```
I will pass the value to the object ***forum*** with the key ***id*** ```forum.id = doc.id```. now we will render the id as a parameter to the ```updateForum()``` function. We will render the id like in the example below:
**forum-details.hbs**
```
<form>
<div class="form-group">
<label for="title">Title</label>
<input type="text" class="form-control" id="title" value="{{forum.title}}" placeholder="Your tittle">
</div>
<div class="form-group">
<label for="desc">Desciptions</label>
<textarea class="form-control" id="desc" rows="3">{{forum.desc}}</textarea>
</div>
<button class="btn btn-info" type="button" name="button" id="update-btn" onclick="updateForum('{{forum.id}}')">Update</button>
</form>
```
We will create a function and will make the document id as a parameter so we can update the forum according to the forum we are editing ```onclick="updateForum('{{forum.id}}')"```.

<br>
- **Create function ```updateForum()```**
In the ```updateForum()``` function, I will receive the **document id** parameter that will be used to access documents in our database, so that we can update the data according to the forum that we are updating. The following is an example of a forum update:
```
function updateForum(id) {
db.collection('forums').doc(id).set({
title : document.getElementById('title').value
desc : document.getElementById('desc').value
updated_at : new Date()
}, {merge : true})
.then(function(){
location.reload()
}).catch(function(err){
console.log(err)
})
}
```
In this function, we will use a ***instance*** of the firebase firestore that we have defined in a var variable ```db = firebase.firestore ();``` and then we can access the collection of ***forums***. then using the parameters that we have passed in this function to access the document we are editing.
For the value values from our forum we can get it from the input form we have created with each id. For the **title** we can get the value from ```document.getElementById('title').value```. For the **desc** we can get the value from ```document.getElementById('desc').value```.
**title and desc** are ***keys*** or fields in the Firestore database. It *should* be noted that the key must be the same as the database, otherwise, an error will occur, firestore will not recognize the field. If all the steps work well, then we can see the results as in the demonstration below:
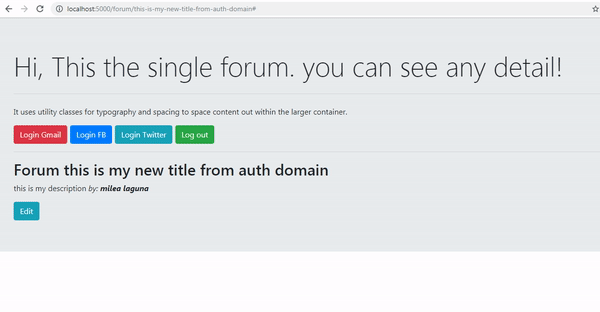.gif)

Well, we can see the result that we have successfully updated the data that is on the **Firestore database**. We have updated the title and desc also updates the update time. Until here is our tutorial, hopefully, you will benefit from this tutorial, thank you.
### Curriculum
- **Forum app**
[Firebase app#1](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-1-firebase-init-and-cloud-backend-function-1557758217688), [Firebase app#2](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-2-local-server-routing-and-template-login-gmail), [Firebase app#3](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-3-logout-function-and-login-with-facebook-account), [Firebase app#4](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-3-logout-function-and-login-with-facebook-account), [Firebase app#5](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-4-create-apps-and-login-with-a-twitter-account-1558448308709), [Firebase app#6](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-6-forums-collection-and-add-data-in-collection-1559196649948), [Firebase app#7](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-7-store-user-data-in-forums-and-firebase-admin-1559372598705), [Firebase app#8](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-8-fetch-data-from-collection-and-render-data-cloud-function-1559462309843), [Firebase app#9](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-9-implement-slug-as-url-params-and-single-app-forum), [Firebase app#10](https://steemit.com/utopian-io/@duski.harahap/firebase-firestore-in-the-forum-application-10-authorized-domain-and-user-forum-ownership-1560338464527),
#### Proof of work done
https://github.com/milleaduski/firebase-forum