#### Repository https://github.com/igormuba/EthereumSolidityClasses/tree/master/class10 #### What Will I Learn? - Multiple inheritance - Diamond problem and how to avoid it - Polymorphism in functions #### Requirements - Internet connection - Code editor - Browser #### Difficulty - Intermediate #### Tutorial Contents Solidity allows you to achieve polymorphism, but you need to be careful with some issues this may cause and you need to be mindful of your contract design choices # Multiple inheritance For this we will use three contracts, the first one is the parent that will have a variable and a function to retrieve it's variable. The second contract is the "child", it will inherit from the parent but will override the parent's function and will implement its own version of that function calling its own variable. Then the last contract will inherit from both the parent and the child and will call the function, that has the same name on both the parent and the child # Parent contract This is the base contract that will be inherited by the next two, it has it's own variable and a function that calls this variable ``` contract parent{ uint parentNumber=1; //unique variable to identify we called this contract function getNumber() public view returns (uint){ return parentNumber; //returns its unique variable } } ``` # Child contract This one inherits from the parent and implements its own function and variable so we can identify whether we called the child or the parent, the function from the parent gets overwritten by the new declaration on the child, so the child is parent but has its own implementation of the `getNumber()` function ``` contract kid is parent{ uint kidNumber=2; //variable different from the parent to identify function getNumber() public view returns (uint){ return kidNumber; //returns the kids variable } } ``` # The contract to test You need to inherit first from the parent, then from the child, both because else you will get an error because of the diamond inheritance problem (more on that later) and because it will help me show you one thing ``` contract test is parent, kid{ //inherits both function callNumber() public view returns (uint){ return getNumber(); //calls the function that has the same name on both } } ``` Now, as you can see, the contract `test` inherits from both the parent and the kid, and it calls the function that has the same name on both, so what do number will show on the result? 1, representing it called the parents variable or 2 for the child? 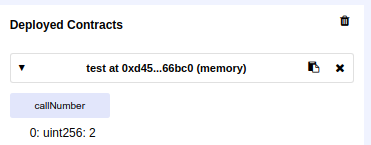 It has returned the kids number, even though we have said it to inherit from the parent first! This is because solidity prioritizes the child that is "the last on the family tree". We can still call the function from the parent, but first I need to explain why # The diamond problem 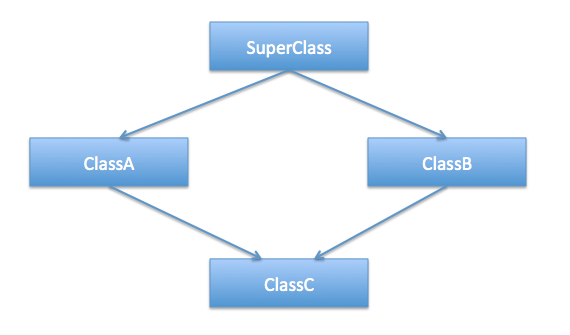 Some programming languages do not allow you to inherit from multiple parents to avoid the diamond problem, Solidity, though, do allow you to inherit from multiple objects. Inheriting from multiple contracts, in this case, allows you to have some cool features, but you need to be mindful of the functions naming. In case the compiler finds the same method on multiple contracts it will not be able to choose which one are you actually calling. By saying `contract test is parent, kid` we are solving the indecision from the compiler by making it explicit that we want the kid function to override the parent one. If you said, however, `contract test is kid, parent` it wouldn't make sense because you would tell the compiler that the functions from the parent are overriding the functions from the kid that are overriding the functions from the parent (because kid is parent). The diamond problem by itself would be worth a whole post, but here are some useful resources on this topic Higher level explanation of the problem: http://www.lambdafaq.org/what-about-the-diamond-problem/ In-depth explanation with examples in c++: https://medium.freecodecamp.org/multiple-inheritance-in-c-and-the-diamond-problem-7c12a9ddbbec A very good example explaining how Solidity deals with the problem https://delegatecall.com/questions/multiple-inheritance-question-9dcaa9e4-af20-4518-9a5d-033b11a0d97e # Calling the parent function In case you want to make a call to the function and get the number from the parent, you need to change the kid and implement this with the keyword `super`, what `super` does is it "ignores" that the function was overwritten and goes right into the parent one. Let me show with an example, on the code below the only thing that changes is the `kid` contract ``` pragma solidity ^0.5.0; contract parent{ uint parentNumber=1; function getNumber() public view returns (uint){ return parentNumber; } } contract kid is parent{ uint kidNumber=2; function getNumber() public view returns (uint){ return super.getNumber(); //searches for this function on the parent } } contract test is parent, kid{ function callNumber() public view returns (uint){ return getNumber(); } } ``` 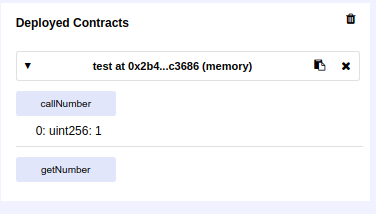 As you can see, this time the parent number was called because we told the kid to search for the function on the parent contract. # One function with many forms Polymorphism means many forms, the same way that contracts can have many forms, functions also can have many forms. One beautiful thing on polymorphism is that you can have multiple functions with the same name if the signature from the functions is different. That means if they have different inputs. In practice, this is as if there is only one function, but this one function has many forms, hence, the word polymorphism See the example below ``` pragma solidity ^0.5.0; contract polymorphism { function manyforms(uint numberOne) public pure returns (uint){ //fist form of function manyforms return numberOne; } function manyforms(uint numberOne, uint numberTwo) public pure returns(uint){ //second form of function manyforms return numberOne+numberTwo; } } ``` On the example above we have successfully implemented two functions with the exact same name, but we have had no errors raised from the Solidity compiler because their signature is different from each other, in this case, the Solidity code will choose which one to call based on the signature of the caller, if you pass to the function `manyforms()` only one number, the first one will be executed, if you pass two, there will be no ambiguity because it is obvious to the computer that you mean to call the second one that deals with two arguments # Calling a polymorphed function With a third function, we can test the polymorphed function `manyforms`, check the newly added function below ``` pragma solidity ^0.5.0; contract polymorphism { function manyforms(uint numberOne) public pure returns (uint){ return numberOne; } function manyforms(uint numberOne, uint numberTwo) public pure returns(uint){ return numberOne+numberTwo; } function callOneForm() public pure returns (uint){ //some code here } } ``` Inside the `callOneForm` we can choose whether we want to send one or two arguments. For this we call the function `manyforms()` and we can either pass one or two numbers, like in ``` function callOneForm() public pure returns (uint){ manyforms(1); } ``` or in ``` function callOneForm() public pure returns (uint){ manyforms(1,3); } ``` Doing the first form first, the one that receives only one number and calling the `callOneForm()` 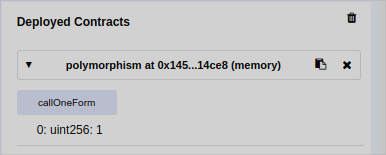 And now on the second form with 1 and 3 as arguments (notice that you have to compile and deploy the contract again) 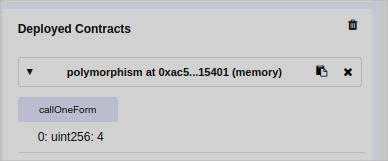 You can keep on adding more forms, maybe you would like to add another `manyforms` function that sums multiple numbers, this allows you to design more concise contracts, with the same functionality but prepared to receive different sets of arguments. #### Curriculum - [(Part 9) Ethereum Solidity Assembly - Return, Memory, Hexadecimal, Pointers, And Memory Addressing(PT 9)](https://steemit.com/utopian-io/@igormuba/part-9-ethereum-solidity-assembly-return-memory-hexadecimal-pointers-and-memory-addressing-pt-9) - [(Part 8) Ethereum Solidity - Assembly, Reducing Costs And Creation Your Low-Level Expression(PT 8)](https://steemit.com/utopian-io/@igormuba/part-8-ethereum-solidity-assembly-reducing-costs-and-creation-your-low-level-expression-pt-8) - [(Part 7) Ethereum Solidity - Fallback, Ethereum Fractions, And Collateral Backed Contract(PT 7)](https://steemit.com/utopian-io/@igormuba/part-7-ethereum-solidity-fallback-ethereum-fractions-and-collateral-backed-contract-pt-7) - [(Part 6) Ethereum Solidity - Custom Variable Functionalities, Libraries, Using Libraries For Security(PT 6)](https://steemit.com/utopian-io/@igormuba/part-6-ethereum-solidity-custom-varaible-functionalities-libraries-using-libraries-for-security-pt-6) - [(Part 5) Ethereum Solidity - Custom Access Modifiers, Security Breach Alerts, Assert And Require(PT 5)](https://steemit.com/utopian-io/@igormuba/part-4-ethereum-solidity-custom-access-modifiers-security-breach-alerts-assert-and-require-pt-4) - [(Part 4) Ethereum Solidity Development - Inheritance, Working With Multiple Contracts And Simple Mutability(PT 4)](https://steemit.com/utopian-io/@igormuba/part-4-ethereum-solidity-development-inheritance-working-with-multiple-contracts-and-simple-mutability-pt-4) - [(Part 3) Ethereum Solidity Development - Contract Mutability, DelegateCall And Calling Functions By Address(PT 3)](https://steemit.com/utopian-io/@igormuba/part-3-ethereum-solidity-development-contract-mutability-delegatecall-and-calling-functions-by-address-pt-3) - [(Part 2) Ethereum Solidity Development - Deploying, Security And ERC20 Compliance(PT 2)](https://steemit.com/utopian-io/@igormuba/part-2-ethereum-solidity-development-deploying-securiy-and-erc20-compliance-pt-2) - [(Part 1) Ethereum Solidity Development - Getting Started + Lower Level Explanation (PT 1)](https://steemit.com/utopian-io/@igormuba/part-1-ethereum-solidity-development-getting-started-lower-level-explanation-pt-1) # Beneficiaries This post has as beneficiaries @utopian.pay with 5% using the SteemPeak beneficiary tool 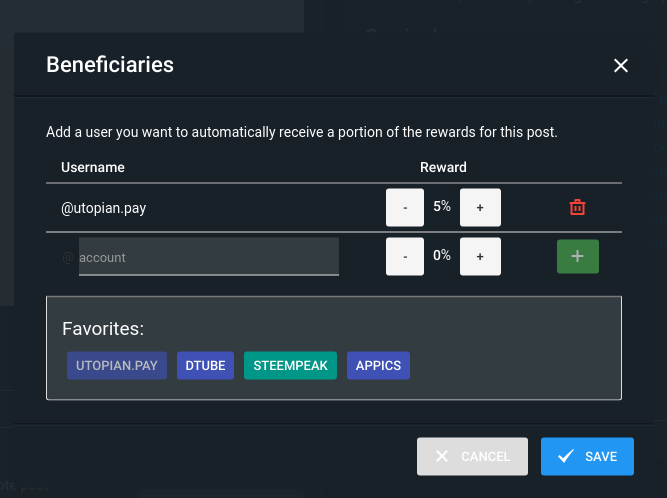
post_id | 68,616,082 | ||||||
---|---|---|---|---|---|---|---|
author | igormuba | ||||||
permlink | part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10 | ||||||
category | utopian-io | ||||||
json_metadata | {"community":"steempeak","app":"steempeak","format":"markdown","tags":["utopian-io","tutorials","ethereum","solidity","blockchain"],"users":["igormuba","utopian.pay"],"links":["https:\/\/github.com\/igormuba\/EthereumSolidityClasses\/tree\/master\/class10","http:\/\/www.lambdafaq.org\/what-about-the-diamond-problem\/","https:\/\/medium.freecodecamp.org\/multiple-inheritance-in-c-and-the-diamond-problem-7c12a9ddbbec","https:\/\/delegatecall.com\/questions\/multiple-inheritance-question-9dcaa9e4-af20-4518-9a5d-033b11a0d97e","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-9-ethereum-solidity-assembly-return-memory-hexadecimal-pointers-and-memory-addressing-pt-9","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-8-ethereum-solidity-assembly-reducing-costs-and-creation-your-low-level-expression-pt-8","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-7-ethereum-solidity-fallback-ethereum-fractions-and-collateral-backed-contract-pt-7","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-6-ethereum-solidity-custom-varaible-functionalities-libraries-using-libraries-for-security-pt-6","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-4-ethereum-solidity-custom-access-modifiers-security-breach-alerts-assert-and-require-pt-4","https:\/\/steemit.com\/utopian-io\/@igormuba\/part-4-ethereum-solidity-development-inheritance-working-with-multiple-contracts-and-simple-mutability-pt-4"],"image":["https:\/\/files.steempeak.com\/file\/steempeak\/igormuba\/LsuVa1St-image.png"]} | ||||||
created | 2019-01-10 01:23:15 | ||||||
last_update | 2019-01-10 01:23:15 | ||||||
depth | 0 | ||||||
children | 8 | ||||||
net_rshares | 51,219,038,642,243 | ||||||
last_payout | 2019-01-17 01:23:15 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 19.052 SBD | ||||||
curator_payout_value | 6.357 SBD | ||||||
pending_payout_value | 0.000 SBD | ||||||
promoted | 0.000 SBD | ||||||
body_length | 10,811 | ||||||
author_reputation | 79,636,306,810,404 | ||||||
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 SBD | ||||||
percent_steem_dollars | 10,000 | ||||||
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
tombstone | 0 | 1,926,829,810,024 | 7.61% | ||
snwolak | 0 | 2,747,304,279 | 50% | ||
matildapurse | 0 | 1,937,037,512 | 22% | ||
eforucom | 0 | 20,591,195,454 | 1% | ||
wagnertamanaha | 0 | 9,011,984,870 | 50% | ||
yehey | 0 | 10,065,044,054 | 10% | ||
mercadosaway | 0 | 1,412,906,385 | 100% | ||
sensation | 0 | 323,831,165 | 100% | ||
codingdefined | 0 | 16,685,266,923 | 15% | ||
bachuslib | 0 | 20,658,629,529 | 100% | ||
ralph-rennoldson | 0 | 945,604,897 | 0.4% | ||
dreamarif | 0 | 95,083,248 | 3% | ||
steemitri | 0 | 138,221,502,700 | 100% | ||
leir | 0 | 1,994,791,285 | 50% | ||
accelerator | 0 | 18,479,612,706 | 1.29% | ||
piaristmonk | 0 | 31,239,342,984 | 100% | ||
espoem | 0 | 7,170,211,963 | 4% | ||
mcfarhat | 0 | 16,036,676,915 | 11.14% | ||
birddroppings | 0 | 2,472,223,462 | 10% | ||
steem-plus | 0 | 3,522,276,798 | 0.28% | ||
utopian-io | 0 | 47,559,909,151,602 | 38.05% | ||
favcau | 0 | 59,917,233,748 | 100% | ||
jaff8 | 0 | 50,231,800,160 | 27.87% | ||
steemtaker | 0 | 2,314,963,676 | 3% | ||
newsrx | 0 | 86,845,673 | 6.96% | ||
shakailove | 0 | 213,856,502 | 2.75% | ||
aiyanna | 0 | 311,686,673 | 2.5% | ||
amosbastian | 0 | 63,517,659,431 | 27.87% | ||
daeshawn | 0 | 364,468,584 | 2.25% | ||
asaj | 0 | 16,940,629,157 | 100% | ||
osobiggie | 0 | 140,450,211 | 1.2% | ||
viperblckz | 0 | 4,289,941,688 | 100% | ||
kennybrown | 0 | 79,852,303 | 1% | ||
tobias-g | 0 | 4,278,417,230 | 2% | ||
amayahaley21 | 0 | 56,911,071 | 1% | ||
wdoutjah | 0 | 61,534,702 | 7.5% | ||
properfraction | 0 | 712,723,060 | 100% | ||
simplymike | 0 | 59,083,695,814 | 30% | ||
sweetpee | 0 | 165,725,192 | 1% | ||
effofex | 0 | 87,548,150 | 0.64% | ||
feronio | 0 | 1,039,392,639 | 100% | ||
indayclara | 0 | 256,718,846 | 7.5% | ||
pinas | 0 | 460,901,159 | 50% | ||
council | 0 | 948,850,636 | 10% | ||
mightypanda | 0 | 56,971,179,057 | 30% | ||
bestofph | 0 | 6,428,513,502 | 15% | ||
ulockblock | 0 | 59,922,066,811 | 21.11% | ||
ferdiycheapza | 0 | 513,645,687 | 100% | ||
contverbasys | 0 | 520,926,388 | 100% | ||
liaguelati | 0 | 525,508,493 | 100% | ||
verclandtebma | 0 | 524,372,785 | 100% | ||
luc.real | 0 | 219,723,789 | 100% | ||
nieloagranca | 0 | 3,184,990,057 | 4% | ||
taylory | 0 | 512,274,900 | 100% | ||
kylietjg4iclark | 0 | 521,130,730 | 100% | ||
steemchoose | 0 | 21,491,700,184 | 1% | ||
haleyy7qfwwhite | 0 | 523,287,138 | 100% | ||
blockgatorsarmy | 0 | 77,294,098 | 1.2% | ||
jmiller05 | 0 | 116,004,810 | 2% | ||
destinymd | 0 | 536,669,725 | 100% | ||
allisonot7 | 0 | 510,888,319 | 100% | ||
kaitlyneu3f5 | 0 | 533,544,944 | 100% | ||
brachetborde | 0 | 514,341,047 | 100% | ||
biolinghave | 0 | 521,107,555 | 100% | ||
bullinachinashop | 0 | 3,839,501,909 | 100% | ||
cutnur | 0 | 0 | 5% | ||
merlin7 | 0 | 76,405,838,331 | 3.6% | ||
steem-ua | 0 | 783,946,215,220 | 6.96% | ||
nfc | 0 | 8,258,771,134 | 2% | ||
teamcr | 0 | 796,301,384 | 100% | ||
curbot | 0 | 2,320,769,066 | 100% | ||
bluesniper | 0 | 54,656,450,171 | 4.45% | ||
primeradue | 0 | 10,240,053,921 | 15% | ||
whitebot | 0 | 56,689,465,555 | 3% | ||
ascorphat | 0 | 730,794,145 | 2.5% | ||
someaddons | 0 | 7,211,560,632 | 100% | ||
bejust | 0 | 1,770,120,954 | 100% | ||
progressing | 0 | 1,592,334,742 | 100% |
Hi, @igormuba! You just got a **0.28%** upvote from SteemPlus! To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn. If you're not using SteemPlus yet, please check our last posts in [here](https://steemit.com/@steem-plus) to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
post_id | 68,629,808 |
---|---|
author | steem-plus |
permlink | part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10---vote-steemplus |
category | utopian-io |
json_metadata | {} |
created | 2019-01-10 09:55:48 |
last_update | 2019-01-10 09:55:48 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-01-17 09:55:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 435 |
author_reputation | 247,995,867,762,997 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Thank you for your contribution. - It is interesting to learn further about solidity concepts and coding approaches. - The description about diamond problem might have been a bit too short, but providing references was helpful. - There were few typos in your content, as well as in the title. - More screenshots would have been a bit more helpful to the user. - Solidity output looks similar somewhat to Java's UI ? Your contribution has been evaluated according to [Utopian policies and guidelines](https://join.utopian.io/guidelines), as well as a predefined set of questions pertaining to the category. To view those questions and the relevant answers related to your post, [click here](https://review.utopian.io/result/8/2-1-1-1-4-3-1-3-). ---- Need help? Chat with us on [Discord](https://discord.gg/uTyJkNm). [[utopian-moderator]](https://join.utopian.io/)
post_id | 68,640,302 |
---|---|
author | mcfarhat |
permlink | re-igormuba-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t151536189z |
category | utopian-io |
json_metadata | {"tags":["utopian-io"],"links":["https:\/\/join.utopian.io\/guidelines","https:\/\/review.utopian.io\/result\/8\/2-1-1-1-4-3-1-3-","https:\/\/discord.gg\/uTyJkNm","https:\/\/join.utopian.io\/"],"app":"steemit\/0.1"} |
created | 2019-01-10 15:15:36 |
last_update | 2019-01-10 15:15:36 |
depth | 1 |
children | 4 |
net_rshares | 16,266,242,061,186 |
last_payout | 2019-01-17 15:15:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 6.368 SBD |
curator_payout_value | 2.018 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 867 |
author_reputation | 104,178,422,702,645 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yuxi | 0 | 32,043,453,673 | 100% | ||
igormuba | 0 | 15,697,059,318 | 100% | ||
codingdefined | 0 | 17,085,952,990 | 15% | ||
espoem | 0 | 27,020,753,545 | 15% | ||
utopian-io | 0 | 15,974,611,020,259 | 11.47% | ||
jaff8 | 0 | 133,031,472,044 | 75% | ||
emrebeyler | 0 | 35,543,195 | 0.01% | ||
amosbastian | 0 | 43,736,374,957 | 19.25% | ||
reazuliqbal | 0 | 6,395,189,540 | 5% | ||
ulockblock | 0 | 12,907,539,520 | 4.7% | ||
ascorphat | 0 | 790,782,207 | 2.5% | ||
yff | 0 | 2,886,919,938 | 100% |
Hi, thank you very much foe the insightful review and for giving me many points where I can improve, I sincerely appreciate By the way, what Java UI are you referring to? The IDE I am using is Remix from Ethereum, I will soon start tutorials about truffle but for now it is Remix only Posted using [Partiko Android](https://steemit.com/@partiko-android)
post_id | 68,640,717 |
---|---|
author | igormuba |
permlink | igormuba-re-mcfarhat-re-igormuba-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t152746319z |
category | utopian-io |
json_metadata | {"app":"partiko","client":"android"} |
created | 2019-01-10 15:28:03 |
last_update | 2019-01-10 15:28:03 |
depth | 2 |
children | 2 |
net_rshares | 22,359,296,775 |
last_payout | 2019-01-17 15:28:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 355 |
author_reputation | 79,636,306,810,404 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
mcfarhat | 0 | 22,359,296,775 | 15% |
You're welcome! I used to code a lot in JAVA many years back. This purple display looks a lot like JAVA runtime UI with its color and layout. 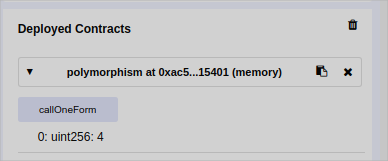
post_id | 68,640,960 |
---|---|
author | mcfarhat |
permlink | re-igormuba-igormuba-re-mcfarhat-re-igormuba-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t153339928z |
category | utopian-io |
json_metadata | {"tags":["utopian-io"],"image":["https:\/\/cdn.steemitimages.com\/DQmdWkvbh77yFsxfCTj8EQxZ2bEiiVQTCdNW4xuGhMj2ZoZ\/image.png"],"app":"steemit\/0.1"} |
created | 2019-01-10 15:33:39 |
last_update | 2019-01-10 15:33:39 |
depth | 3 |
children | 1 |
net_rshares | 0 |
last_payout | 2019-01-17 15:33:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 234 |
author_reputation | 104,178,422,702,645 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Thank you for your review, @mcfarhat! Keep up the good work!
post_id | 68,733,263 |
---|---|
author | utopian-io |
permlink | re-re-igormuba-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t151536189z-20190112t195825z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.9"} |
created | 2019-01-12 19:58:27 |
last_update | 2019-01-12 19:58:27 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-01-19 19:58:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 60 |
author_reputation | 152,913,012,544,965 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
#### Hi @igormuba! Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation! Your post is eligible for our upvote, thanks to our collaboration with @utopian-io! **Feel free to join our [@steem-ua Discord server](https://discord.gg/KpBNYGz)**
post_id | 68,641,538 |
---|---|
author | steem-ua |
permlink | re-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t154616z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.14"} |
created | 2019-01-10 15:46:18 |
last_update | 2019-01-10 15:46:18 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-01-17 15:46:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 287 |
author_reputation | 23,203,609,903,979 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hey, @igormuba! **Thanks for contributing on Utopian**. Weβre already looking forward to your next contribution! **Get higher incentives and support Utopian.io!** Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via [SteemPlus](https://chrome.google.com/webstore/detail/steemplus/mjbkjgcplmaneajhcbegoffkedeankaj?hl=en) or [Steeditor](https://steeditor.app)). **Want to chat? Join us on Discord https://discord.gg/h52nFrV.** <a href='https://steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1'>Vote for Utopian Witness!</a>
post_id | 68,655,366 |
---|---|
author | utopian-io |
permlink | re-part-10-ethereum-solidity-multiple-inheritance-diaomond-problem-and-function-polymorphism-pt-10-20190110t223609z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.9"} |
created | 2019-01-10 22:36:09 |
last_update | 2019-01-10 22:36:09 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-01-17 22:36:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 590 |
author_reputation | 152,913,012,544,965 |
root_title | "(Part 10) Ethereum Solidity - Multiple inheritance, Diaomond Problem And Function Polymorphism(PT 10)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |