## Solution The problem of this contest was to test two methods to approximate pi and measure how accurate they get compared to how many digits it gets right. I wrote a simple code which uses the following two methods: 1. Count how many grid dots are in a circle(can be done in linear time when using the circle function). 2. Use the following sequence to apprixmate it: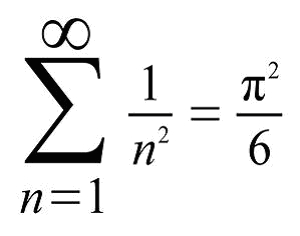 I decided to go with a maximum accuracy of twenty binary digits. Here is my code ``` import javax.swing.JFrame; import java.awt.Graphics; import java.awt.Color; public class abcd extends JFrame { int[] firstMethod; int[] secondMethod; public abcd() { setSize(800, 400); setVisible(true); doFirstMethod(); doSecondMethod(); repaint(); } // return accuracy in bits: public int getAccuracy(double approx) { if((int)approx != 3) return 0; long approxL = Double.doubleToLongBits(approx); long piL = Double.doubleToLongBits(Math.PI); long mantissaStart = 0x0008000000000000L; int prec = 0; for(long i = mantissaStart; i >= 0; i >>= 1) { if((approxL & i) != (piL & i)) return prec; prec++; } return prec; } public void doFirstMethod() { firstMethod = new int[20]; int index = 0; for(int i = 0; index < 20; i += 1+(i>>3)) { double π = countDots(i); int numDigits = getAccuracy(π); if(numDigits >= index+1) { // Repeat the calculation a couple of times to increase time accuracy long t1 = System.nanoTime(); for(int j = 0; j < 1000000; j++) getAccuracy(π); long t2 = System.nanoTime(); firstMethod[index] = (int)((t2-t1)/1000000.0); index++; } } } public void doSecondMethod() { secondMethod = new int[20]; int index = 0; for(int i = 0; index < 20; i += 1+(i>>3)) { double π = sumUp(i); int numDigits = getAccuracy(π); if(numDigits >= index+1) { // Repeat the calculation a couple of times to increase time accuracy long t1 = System.nanoTime(); for(int j = 0; j < 1000000; j++) getAccuracy(π); long t2 = System.nanoTime(); secondMethod[index] = (int)((t2-t1)/1000000.0); index++; } } } // Use the partial sums of the infinite sequence sum 1/n² = π²/6: public double sumUp(int n) { double sum = 0; for(int i = 1; i <= n; i++) { sum += 1.0/i/i; } return Math.sqrt(sum*6); } // Counts how many grid points lie in-/outside a quarter circle. Kind of similar to integrating the circle function. public double countDots(int r) { long num = 0; long rSqr = r*r; for(int i = 0; i < r; i++) { num += (long)(Math.sqrt(rSqr-i*i)); // Use the floor function to find the number of grid points inside the circle at this position. } return 4*num/(double)rSqr; } public void paint(Graphics g) { g.setColor(Color.BLACK); for(int i = 1; i < 20; i++) { g.drawLine(i*10, 200-firstMethod[i-1], i*10+10, 200-firstMethod[i]); g.drawLine(200+i*10, 200-secondMethod[i-1], 200+i*10+10, 200-secondMethod[i]); } } public static void main(String[] args) { new abcd(); } } ``` This code outputs the following two graphs: 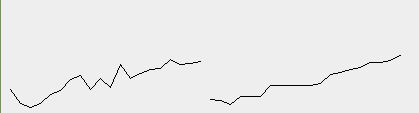 Not much to see except from the fact that both graphs are growing kind of linear. *↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓* ### List of participants with their entries: Name | Solution found | Comment - | - | - @lallo | Integrating the circle function | Strange that your function seems to grow exponentially, while mine are at least on the scale I observed mostly linear. Probably python is doing something strange? *↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓* ## Winners: Congratulations @lallo, you won 2 SBI! *↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓↑↓* ### The next contest starts tomorrow. Don't miss it!
post_id | 82,554,330 |
---|---|
author | quantumdeveloper |
permlink | computation-contest-7-results-and-solution |
category | programming |
json_metadata | {"tags":["programming","puzzle","contest","steembasicincome","stem","math","steemstem","steemiteducation","palnet","sbi"],"users":["lallo"],"image":["http:\/\/www.people.vcu.edu\/%7Epdattalo\/sum_infinite_series.jpg","https:\/\/cdn.steemitimages.com\/DQmYSwjWAxGnkxfca2Qyp87HuPV5n8VGMPWdLMCNyqXBucA\/Screenshot%20from%202019-12-14%2019-52-06.png"],"app":"palnet\/0.1","format":"markdown","canonical_url":"undefined\/@quantumdeveloper\/computation-contest-7-results-and-solution"} |
created | 2019-12-14 19:10:21 |
last_update | 2019-12-14 19:10:21 |
depth | 0 |
children | 6 |
net_rshares | 1,637,460,246,901 |
last_payout | 2019-12-21 19:10:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.159 SBD |
curator_payout_value | 0.154 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 3,935 |
author_reputation | 4,501,252,062,061 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
zorg67 | 0 | 1,302,956,820 | 100% | ||
edkarnie | 0 | 75,358,245,189 | 20% | ||
crokkon | 0 | 60,265,877,119 | 100% | ||
storysharing | 0 | 20,052,185,273 | 100% | ||
dobartim | 0 | 142,975,305,674 | 2% | ||
mytechtrail | 0 | 42,079,237,283 | 25% | ||
mmmmkkkk311 | 0 | 0 | -15% | ||
stmdev | 0 | 148,936,128 | 1% | ||
gadrian | 0 | 73,553,697,108 | 30% | ||
tonimontana | 0 | 1,687,518,764 | 100% | ||
nin4i | 0 | 58,156,643 | 100% | ||
sbi6 | 0 | 233,542,619,147 | 17.24% | ||
zuerich | 0 | 449,320,912,526 | 30% | ||
light-hearted | 0 | 242,477,777 | 100% | ||
skylinebuds | 0 | 1,362,366,650 | 2% | ||
definethedollar | 0 | 167,323,329,996 | 50% | ||
remlaps-lite | 0 | 11,077,216,147 | 100% | ||
laissez-faire | 0 | 73,936,962 | 100% | ||
darklands | 0 | 106,057,517,608 | 58% | ||
lallo | 0 | 12,976,496,850 | 100% | ||
driveforkids | 0 | 34,059,843,546 | 40% | ||
limka | 0 | 0 | 1% | ||
constant-flux | 0 | 5,168,319 | 45% | ||
onespringday | 0 | 1,244,990,848 | 10% | ||
onecent | 0 | 1,441,676,482 | 22% | ||
babytarazkp | 0 | 6,758,884,994 | 100% | ||
trendovoter | 0 | 78,608,464,293 | 55.45% | ||
mapxv | 0 | 41,240,194,154 | 4.59% | ||
abh12345.stem | 0 | 529,317,361 | 50% | ||
giphy | 0 | 16,101,311,994 | 10% | ||
brustem | 0 | 348,942,071 | 100% | ||
untersatz | 0 | 53,608,627,170 | 10% | ||
stem.alfa | 0 | 448,439,164 | 100% | ||
stemd | 0 | 320,065,984 | 100% | ||
thebilpcointrain | 0 | 63,647,718 | 1% | ||
bilpcoin.pay | 0 | 201,617,443 | 1% | ||
yggdrasil.laguna | 0 | 336,623,957 | 70% | ||
cd-stem | 0 | 542,913,606 | 100% | ||
miti.stem | 0 | 336,782,309 | 100% | ||
stemisaria | 0 | 1,833,623,394 | 100% | ||
suhinal | 0 | -9,169,322 | -10% | ||
copral | 0 | -2,382,950 | -10% | ||
hiphup | 0 | -9,162,649 | -10% | ||
fionnet | 0 | -9,162,649 | -10% | ||
updooter | 0 | 0 | 100% | ||
ilovemk311 | 0 | 0 | 50% | ||
mashkova | 0 | 0 | 100% |
A member bonus $trendotoken tip, and !trendovoter, for @quantumdeveloper from MAPXV! These bonuses are for members, selected at random, and last for a few days. Also consider our [MAPR fund](https://steem-engine.com/?p=market&t=MAPR) and [MAXUV](https://steem-engine.com/?p=market&t=MAXUV) vote bonds too. MAP Steem Fintech: growing your STEEM without SP.
post_id | 82,554,511 |
---|---|
author | mapxv |
permlink | re-computation-contest-7-results-and-solution-20191214t191824z |
category | programming |
json_metadata | {"app":"rewarding\/0.1.5"} |
created | 2019-12-14 19:18:27 |
last_update | 2019-12-14 19:18:27 |
depth | 1 |
children | 1 |
net_rshares | 0 |
last_payout | 2019-12-21 19:18:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 361 |
author_reputation | 9,672,874,554,958 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Congratulations @mapxv, you successfuly trended the post shared by @quantumdeveloper! @quantumdeveloper will receive <b>4.40698725</b> [TRDO](https://steem-engine.com/?p=history&t=TRDO) & @mapxv will get <b>2.93799150</b> [TRDO](https://steem-engine.com/?p=history&t=TRDO) curation in 3 Days from Post Created Date! <b>"Call [TRDO](https://steem-engine.com/?p=history&t=TRDO), Your Comment Worth Something!"</b> --- <sup>To view or trade TRDO go to [steem-engine.com](https://steem-engine.com/?p=market&t=TRDO) Join [TRDO Discord Channel](https://discord.gg/wySP8T9) or Join [TRDO Web Site](http://www.trendotoken.info/)</sup>
post_id | 82,554,514 |
---|---|
author | trendotoken |
permlink | re-mapxv-re-computation-contest-7-results-and-solution-20191214t191824z-20191214t191837699z |
category | programming |
json_metadata | {"tags":["comments-scot","trendo-bot"],"app":"comments-scot\/1.1","format":"markdown"} |
created | 2019-12-14 19:18:39 |
last_update | 2019-12-14 19:18:39 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-12-21 19:18:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 628 |
author_reputation | 6,277,370,726,158 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Congratulations @mapxv, <b>55.45%</b> upvote has been shared with your successful call on the post that shared by @quantumdeveloper! --- <sup>Support <b>@trendotoken</b> projects by delegating : <b>[100SP](https://beta.steemconnect.com/sign/delegateVestingShares?delegator=YOURUSERNAME&delegatee=trendovoter&vesting_shares=100.000%20SP)</b> , <b>[200SP](https://beta.steemconnect.com/sign/delegateVestingShares?delegator=YOURUSERNAME&delegatee=trendovoter&vesting_shares=200.000%20SP) , <b>[500SP](https://beta.steemconnect.com/sign/delegateVestingShares?delegator=YOURUSERNAME&delegatee=trendovoter&vesting_shares=500.000%20SP)</b> , <b>[1000SP](https://beta.steemconnect.com/sign/delegateVestingShares?delegator=YOURUSERNAME&delegatee=trendovoter&vesting_shares=1000.000%20SP)</b> , <b>[2000SP](https://beta.steemconnect.com/sign/delegateVestingShares?delegator=YOURUSERNAME&delegatee=trendovoter&vesting_shares=2000.000%20SP)</b></sup>
post_id | 82,554,515 |
---|---|
author | trendovoter |
permlink | re-quantumdeveloper-computation-contest-7-results-and-solution-20191214t191839047z |
category | programming |
json_metadata | {"tags":["trdo","newsteem"],"app":"trdo-voter\/1.0","format":"markdown"} |
created | 2019-12-14 19:18:39 |
last_update | 2019-12-14 19:18:39 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-12-21 19:18:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 944 |
author_reputation | 60,565,069,820 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
A bonus $trendotoken tip from ONECENT! Also consider [MAPR fund](https://steem-engine.com/?p=market&t=MAPR) and [MAXUV](https://steem-engine.com/?p=market&t=MAXUV) vote bonds too. MAP Steem FinTech: growing your STEEM without SP.
post_id | 82,554,534 |
---|---|
author | onecent |
permlink | re-computation-contest-7-results-and-solution-20191214t191936z |
category | programming |
json_metadata | {"app":"rewarding\/0.1.5"} |
created | 2019-12-14 19:19:39 |
last_update | 2019-12-14 19:19:39 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-12-21 19:19:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 231 |
author_reputation | 16,426,897,814,016 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
I think in my case it grows exponentially because my algorithm doesn't stop when a certain level of precision is achieved, but it stops after doing n iterations. So it depends on the input that is exponential. But I'm not sure. I should modify the algorithm and find the exactly number of iteration and time needed to achieve 1,2,3,4,5,... decimal digits correct. And see if it changes.
post_id | 82,555,235 |
---|---|
author | lallo |
permlink | q2iq8n |
category | programming |
json_metadata | {"app":"steemit\/0.1"} |
created | 2019-12-14 20:00:30 |
last_update | 2019-12-14 20:00:30 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-12-21 20:00:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 386 |
author_reputation | 8,754,316,063,692 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Congratulations @quantumdeveloper, your post successfully recieved <b>4.40698725</b> [TRDO](https://steem-engine.com/?p=history&t=TRDO) from below listed TRENDO callers:<br> > <sup>@mapxv earned : **2.9379915** [TRDO](https://steem-engine.com/?p=history&t=TRDO) curation</sup> --- <sup>To view or trade TRDO go to [steem-engine.com](https://steem-engine.com/?p=market&t=TRDO) Join [TRDO Discord Channel](https://discord.gg/wySP8T9) or Join [TRDO Web Site](http://www.trendotoken.info/)</sup>
post_id | 82,633,499 |
---|---|
author | trendotoken |
permlink | re-quantumdeveloper-computation-contest-7-results-and-solution-20191217t193109359z |
category | programming |
json_metadata | {"tags":["trdo","trendo-bot"],"app":"comments-scot\/1.1","format":"markdown"} |
created | 2019-12-17 19:31:27 |
last_update | 2019-12-17 19:31:27 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-12-24 19:31:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 494 |
author_reputation | 6,277,370,726,158 |
root_title | "Computation Contest #7 Results and Solution" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |