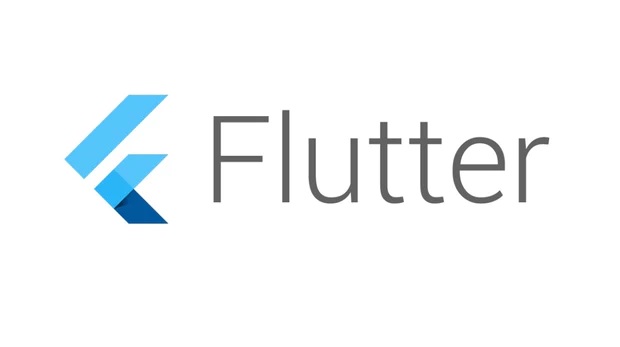 #### Repository https://github.com/flutter/flutter #### What Will I Learn? - You will learn how to use add authentication to Firebase/Firestore projects - You will learn how to use OAuth 2.0 with Firebase/Firestore - You will learn how to use image filter widgets in flutter - You will learn how to manage singleton object in a provider class - You will learn how to use assertions and logic to verify data #### Requirements ##### System Requirements: - [IDEA intellij](https://www.jetbrains.com/idea/), [Visual Studio Code](https://code.visualstudio.com/) with the Dart/Flutter [Plugins](https://github.com/flutter/flutter-intellij), [Android Studio](https://developer.android.com/studio/index.html) or [Xcode](https://developer.apple.com/xcode/) - The [Flutter SDK](https://flutter.io/get-started/install/) on the latest Master Build - An Android or iOS Emulator or device for testing ##### OS Support for Flutter: - Windows 7 SP1 or later (64-bit) - macOS (64-bit) - Linux (64-bit) #### Required Knowledge - A little understanding of key/value stores - A fair understanding of Mobile development and Imperative or Object Oriented Programming - Some understanding of realtime databases #### Resources for Flutter and this Project: - Flutter Website: https://flutter.io/ - Flutter Official Documentation: https://flutter.io/docs/ - Flutter Installation Information: https://flutter.io/get-started/install/ - Flutter GitHub repository: https://github.com/flutter/flutter - Flutter Dart 2 Preview Information: https://github.com/flutter/flutter/wiki/Trying-the-preview-of-Dart-2-in-Flutter - Flutter Technical Overview: https://flutter.io/technical-overview/ - Dart Website: https://www.dartlang.org/ - Flutter Awesome GitHub Repository: https://github.com/Solido/awesome-flutter #### Sources: Flutter Logo (Google): https://flutter.io/ #### Difficulty - Intermediate #### Description ##### Outline and Overview In this **Flutter Video Tutorial**, we take a look at how it is possible to authenticate a user using the **Google Sign In** plugin inside of **Firebase** and **Firestore**. The application uses a login page which allows the user to navigate to the home page only if the user is authenticated by Google. Google sends a token to the **Firebase console** which them verifies that the user is authenticated. If they are authenticated then the user can read and write to our **Firestore database**. We also make use of the Image Filter Widget in this application to allow us to create a unique aesthetic for our login page. The Singleton pattern is also used and we use assertions in our provider logic. ##### Outline for this Tutorial ##### Item 1: Authentication via Google for Firebase/Firestore There are various reasons why you would want to authenticate a user in a mobile application. With **Firebase** we are able to not only restrict which users can read and write from our database, we can also use the authentication to identify the users. This allows us to preform **analytics** as well as save **security data** in the cloud. We are also able to **personalize the application experience** with this information to increase application retention. 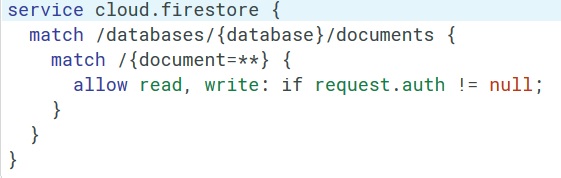 This image shows the rules that were used for our **cloud firestore database** in this application. These **rules** only allow a user to **read and write our data** if the request they make is **authenticated** beforehand. This rule set is very simple but it can be expanded in different ways for various types of applications. ##### Item 2: Using OAuth 2.0 Inside of Flutter One of the larger advantages of using the **Firebase Authentication system** is that it makes use of the **OAuth 2.0 standard**. This allows us to authenticate our users from a single click. We also **avoid potential security risks** which come from using a more traditional validation system that actually requires that the user inputs their email and password into our application. 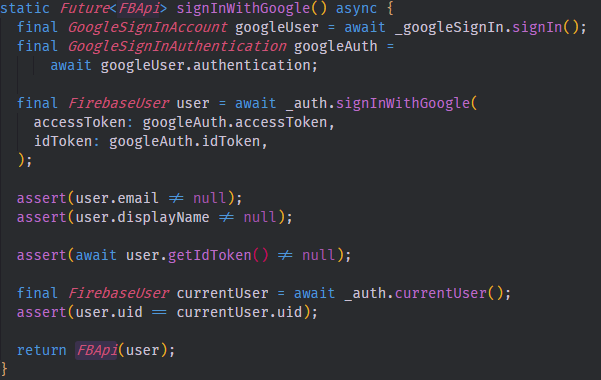 This image shows the method that we use to authenticate the user inside of our application. First, we ask **Google** to authenticate the user by having them sign in to the **Google Playstore** or another **Google application**. We then are able to get the Authentication Tokens from this sign in event through our `GoogleSignInAuthentication` object. These tokens are passed into the **FirebaseUser object** which then confirms that the user is indeed who they say they are. The information is then passed back into **firebase** on a **successful authentication**. ##### Item 3: Using the Singleton Pattern to Fetch Consistent Data **Firebase Authentication** and the libraries surrounding Firebase all use the **Singleton Pattern**. This is useful because it allows the application to keep the **same instance of the object** consistent across the entire code base. In this case, we are able to easily pass the user data from the **API provider** to the **view layer** of our application.  Just as we were able to use a **Stream Builder widget** to dynamically change the widget tree in the base application, we are also able to display information about the user to our home screen. In this case, we are taking the `FirebaseAuth` instance object and calling the `currentUser` method on it to get the currently signed in user. We are then able to access any piece of information associated with this user in the widget tree below this **Stream Builder**. The source code for this project can be found [here](https://github.com/tensor-programming/flutter_fb_google_auth_example) The Commands and Firebase Rules used in this tutorial can be found [here](https://gist.github.com/tensor-programming/9a5a24874860cfa365b1049d094465d7) #### Video Tutorial <iframe width="560" height="315" src="https://www.youtube.com/embed/ZNt_e5ojGzQ" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe> #### Related Videos - [Using Cloud Firestore as a Realtime Datastore for CRUD with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-cloud-firestore-as-a-realtime-datastore-for-crud-with-dart-s-flutter-framework) #### Projects and Series > ##### Stand Alone Projects: > - [Dart Flutter Cross Platform Chat Application Tutorial](https://steemit.com/@tensor/dart-flutter-cross-platform-chat-application-tutorial) > - [Building a Temperature Conversion Application using Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-temperature-conversion-application-using-dart-s-flutter-framework) > - [Managing State with Flutter Flux and Building a Crypto Tracker Application with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/managing-state-with-flutter-flux-and-building-a-crypto-tracker-application-with-dart-s-flutter-framework) > ##### Building a Calculator > - [Building a Calculator Layout using Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-calculator-layout-using-dart-s-flutter-framework) > - [Finishing our Calculator Application with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/finishing-our-calculator-application-with-dart-s-flutter-framework) > ##### Movie Searcher Application > - [Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 1)](https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-1) > - [Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 2)](https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-2) > - [Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 3, Final)](https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-3-final) > #### Minesweeper Game > - [Building a Mine Sweeper Game using Dart's Flutter Framework (Part 1)](https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-1) > - [Building a Mine Sweeper Game using Dart's Flutter Framework (Part 2)](https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-2) > - [Building a Mine Sweeper Game using Dart's Flutter Framework (Part 3)](https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-3) > - [Building a Mine Sweeper Game using Dart's Flutter Framework (Part 4, Final)](https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-4-final) > #### Weather Application > - [Building a Weather Application with Dart's Flutter Framework (Part 1, Handling Complex JSON with Built Code Generation)](https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-1-handling-complex-json-with-built-code-generation) > - [Building a Weather Application with Dart's Flutter Framework (Part 2, Creating a Repository and Model)](https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-2-creating-a-repository-and-model) > - [Building a Weather Application with Dart's Flutter Framework (Part 3, RxCommand (RxDart) and Adding an Inherited Widget)](https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-3-rxcommand-rxdart-and-adding-an-inherited-widget) > - [Building a Weather Application with Dart's Flutter Framework (Part 4, Using RxWidget to Build a Reactive User Interface)](https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-4-using-rxwidget-to-build-a-reactive-user-interface) > - [Localize and Internationalize Applications with Intl and the Flutter SDK in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/localize-and-internationalize-applications-with-intl-and-the-flutter-sdk-in-dart-s-flutter-framework) #### Curriculum - [Building a Multi-Page Application with Dart's Flutter Mobile Framework](https://steemit.com/utopian-io/@tensor/building-a-multi-page-application-with-dart-s-flutter-mobile-framework) - [Making Http requests and Using Json in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/making-http-requests-and-using-json-in-dart-s-flutter-framework) - [Building Dynamic Lists with Streams in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-dynamic-lists-with-streams-in-dart-s-flutter-framework) - [Using GridView, Tabs, and Steppers in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-gridview-tabs-and-steppers-in-dart-s-flutter-framework) - [Using Global Keys to get State and Validate Input in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-global-keys-to-get-state-and-validate-input-in-dart-s-flutter-framework) - [The Basics of Animation with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/the-basics-of-animation-with-dart-s-flutter-framework) - [Advanced Physics Based Animations in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/advanced-physics-based-animations-in-dart-s-flutter-framework) - [Building a Drag and Drop Application with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-drag-and-drop-application-with-dart-s-flutter-framework) - [Building a Hero Animation and an Application Drawer in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-hero-animation-and-an-application-drawer-in-dart-s-flutter-framework) - [Using Inherited Widgets and Gesture Detectors in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-inherited-widgets-and-gesture-detectors-in-dart-s-flutter-framework) - [Using Gradients, Fractional Offsets, Page Views and Other Widgets in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-gradients-fractional-offsets-page-views-and-other-widgets-in-dart-s-flutter-framework) - [Making use of Shared Preferences, Flex Widgets and Dismissibles with Dart's Flutter framework](https://steemit.com/utopian-io/@tensor/making-use-of-shared-preferences-flex-widgets-and-dismissibles-with-dart-s-flutter-framework) - [Using the Different Style Widgets and Properties in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-the-different-style-widgets-and-properties-in-dart-s-flutter-framework) - [Composing Animations and Chaining Animations in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/composing-animations-and-chaining-animations-in-dart-s-flutter-framework) - [Building a Countdown Timer with a Custom Painter and Animations in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-countdown-timer-with-a-custom-painter-and-animations-in-dart-s-flutter-framework) - [Reading and Writing Data and Files with Path Provider using Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/reading-and-writing-data-and-files-with-path-provider-using-dart-s-flutter-framework) - [Exploring Webviews and the Url Launcher Plugin in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/exploring-webviews-and-the-url-launcher-plugin-in-dart-s-flutter-framework) - [Adding a Real-time Database to a Flutter application with Firebase](https://steemit.com/utopian-io/@tensor/adding-a-real-time-database-to-a-flutter-application-with-firebase) - [Building a List in Redux with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-list-in-redux-with-dart-s-flutter-framework) - [Managing State with the Scoped Model Pattern in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/managing-state-with-the-scoped-model-pattern-in-dart-s-flutter-framework) - [Authenticating Guest Users for Firebase using Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/authenticating-guest-users-for-firebase-using-dart-s-flutter-framework) - [How to Monetize Your Flutter Applications Using Admob](https://steemit.com/utopian-io/@tensor/how-to-monetize-your-flutter-applications-using-admob) - [Using Geolocator to Communicate with the GPS and Build a Map in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-geolocator-to-communicate-with-the-gps-and-build-a-map-in-dart-s-flutter-framework) - [Managing the App Life Cycle and the Screen Orientation in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/managing-the-app-life-cycle-and-the-screen-orientation-in-dart-s-flutter-framework) - [Making use of General Utility Libraries for Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/making-use-of-general-utility-libraries-for-dart-s-flutter-framework) - [Interfacing with Websockets and Streams in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/interfacing-with-websockets-and-streams-in-dart-s-flutter-framework) - [Playing Local, Network and YouTube Videos with the Video Player Plugin in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/playing-local-network-and-youtube-videos-with-the-video-player-plugin-in-dart-s-flutter-framework) - [Building Custom Scroll Physics and Simulations with Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-custom-scroll-physics-and-simulations-with-dart-s-flutter-framework) - [Making Dynamic Layouts with Slivers in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/making-dynamic-layouts-with-slivers-in-dart-s-flutter-framework) - [Building a Sketch Application by using Custom Painters in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/building-a-sketch-application-by-using-custom-painters-in-dart-s-flutter-framework) - [Using Dart Isolates, Dependency Injection and Future Builders in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/using-dart-isolates-dependency-injection-and-future-builders-in-dart-s-flutter-framework) - [Looking at the Main Features of the Beta Three Release of Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/looking-at-the-main-features-of-the-beta-three-release-of-dart-s-flutter-framework) - [Working with Material Theme and Custom Fonts in Dart's Flutter Framework](https://steemit.com/utopian-io/@tensor/working-with-material-theme-and-custom-fonts-in-dart-s-flutter-framework) #### Proof of Work Done https://github.com/tensor-programming
post_id | 48,592,325 |
---|---|
author | tensor |
permlink | authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework |
category | utopian-io |
json_metadata | "{"links": ["https://github.com/flutter/flutter", "https://www.jetbrains.com/idea/", "https://code.visualstudio.com/", "https://github.com/flutter/flutter-intellij", "https://developer.android.com/studio/index.html", "https://developer.apple.com/xcode/", "https://flutter.io/get-started/install/", "https://flutter.io/", "https://flutter.io/docs/", "https://github.com/flutter/flutter/wiki/Trying-the-preview-of-Dart-2-in-Flutter", "https://flutter.io/technical-overview/", "https://www.dartlang.org/", "https://github.com/Solido/awesome-flutter", "https://github.com/tensor-programming/flutter_fb_google_auth_example", "https://gist.github.com/tensor-programming/9a5a24874860cfa365b1049d094465d7", "https://www.youtube.com/embed/ZNt_e5ojGzQ", "https://steemit.com/utopian-io/@tensor/using-cloud-firestore-as-a-realtime-datastore-for-crud-with-dart-s-flutter-framework", "https://steemit.com/@tensor/dart-flutter-cross-platform-chat-application-tutorial", "https://steemit.com/utopian-io/@tensor/building-a-temperature-conversion-application-using-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/managing-state-with-flutter-flux-and-building-a-crypto-tracker-application-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-calculator-layout-using-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/finishing-our-calculator-application-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-1", "https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-2", "https://steemit.com/utopian-io/@tensor/building-a-movie-searcher-with-rxdart-and-sqlite-in-dart-s-flutter-framework-part-3-final", "https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-1", "https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-2", "https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-3", "https://steemit.com/utopian-io/@tensor/building-a-mine-sweeper-game-using-dart-s-flutter-framework-part-4-final", "https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-1-handling-complex-json-with-built-code-generation", "https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-2-creating-a-repository-and-model", "https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-3-rxcommand-rxdart-and-adding-an-inherited-widget", "https://steemit.com/utopian-io/@tensor/building-a-weather-application-with-dart-s-flutter-framework-part-4-using-rxwidget-to-build-a-reactive-user-interface", "https://steemit.com/utopian-io/@tensor/localize-and-internationalize-applications-with-intl-and-the-flutter-sdk-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-multi-page-application-with-dart-s-flutter-mobile-framework", "https://steemit.com/utopian-io/@tensor/making-http-requests-and-using-json-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-dynamic-lists-with-streams-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-gridview-tabs-and-steppers-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-global-keys-to-get-state-and-validate-input-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/the-basics-of-animation-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/advanced-physics-based-animations-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-drag-and-drop-application-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-hero-animation-and-an-application-drawer-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-inherited-widgets-and-gesture-detectors-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-gradients-fractional-offsets-page-views-and-other-widgets-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/making-use-of-shared-preferences-flex-widgets-and-dismissibles-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-the-different-style-widgets-and-properties-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/composing-animations-and-chaining-animations-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-countdown-timer-with-a-custom-painter-and-animations-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/reading-and-writing-data-and-files-with-path-provider-using-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/exploring-webviews-and-the-url-launcher-plugin-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/adding-a-real-time-database-to-a-flutter-application-with-firebase", "https://steemit.com/utopian-io/@tensor/building-a-list-in-redux-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/managing-state-with-the-scoped-model-pattern-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/authenticating-guest-users-for-firebase-using-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/how-to-monetize-your-flutter-applications-using-admob", "https://steemit.com/utopian-io/@tensor/using-geolocator-to-communicate-with-the-gps-and-build-a-map-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/managing-the-app-life-cycle-and-the-screen-orientation-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/making-use-of-general-utility-libraries-for-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/interfacing-with-websockets-and-streams-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/playing-local-network-and-youtube-videos-with-the-video-player-plugin-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-custom-scroll-physics-and-simulations-with-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/making-dynamic-layouts-with-slivers-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/building-a-sketch-application-by-using-custom-painters-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/using-dart-isolates-dependency-injection-and-future-builders-in-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/looking-at-the-main-features-of-the-beta-three-release-of-dart-s-flutter-framework", "https://steemit.com/utopian-io/@tensor/working-with-material-theme-and-custom-fonts-in-dart-s-flutter-framework", "https://github.com/tensor-programming"], "format": "markdown", "app": "steemit/0.1", "image": ["https://steemitimages.com/DQmbFAKjCq1GWcT8qxs3NWXo5zJywJcVv9Eec35euxMs41F/flutter-logo.jpg"], "tags": ["utopian-io", "video-tutorials", "steemstem", "steemiteducation", "technology"]}" |
created | 2018-05-15 02:23:00 |
last_update | 2018-05-15 02:25:54 |
depth | 0 |
children | 5 |
net_rshares | 26,449,372,345,272 |
last_payout | 2018-05-22 02:23:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 99.424 SBD |
curator_payout_value | 30.640 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 16,905 |
author_reputation | 87,767,420,253,600 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
freyman | 0 | 15,350,673,214 | 100% | ||
penguinpablo | 0 | 396,975,688,485 | 20% | ||
yuxi | 0 | 4,304,662,235 | 20% | ||
redes | 0 | 876,324,619,198 | 18% | ||
tibra | 0 | 0 | 100% | ||
mercadosaway | 0 | 1,276,982,450 | 100% | ||
sensation | 0 | 209,005,639 | 100% | ||
steemitri | 0 | 100,894,776,887 | 100% | ||
mlaura | 0 | 482,340,222 | 100% | ||
piaristmonk | 0 | 7,235,215,967 | 100% | ||
naegling11 | 0 | 307,741,082 | 1% | ||
utopian-io | 0 | 24,895,157,277,458 | 17% | ||
steemitstats | 0 | 113,644,801 | 5% | ||
rantar | 0 | 20,385,814,027 | 100% | ||
cryptonized | 0 | 44,559,071,329 | 20% | ||
luoq | 0 | 26,096,645,652 | 100% | ||
tdre | 0 | 29,927,109,477 | 66% | ||
edetebenezer | 0 | 3,098,034,957 | 100% | ||
midun | 0 | 2,434,718,939 | 100% | ||
tdogvoid | 0 | 220,812,011 | 100% | ||
polbot | 0 | 788,244,158 | 100% | ||
mindark | 0 | 482,825,953 | 100% | ||
sbi2 | 0 | 6,377,497,304 | 2% | ||
qurator-tier-0 | 0 | 6,981,224,234 | 2% | ||
nwambe | 0 | 614,451,323 | 100% | ||
bobzone | 0 | 5,713,578,629 | 100% | ||
steem.curator | 0 | 702,870,128 | 1% | ||
lucky-robin | 0 | 1,678,985,599 | 100% | ||
steem0r | 0 | 589,302,104 | 100% | ||
alan369 | 0 | 88,531,810 | 100% |
<div class="pull-left"><img src="" /></div> <center>You just received a Tier 0 upvote! Looking for bigger rewards? Click [here](https://steemit.com/qurator/@qurator/qurator-tier-changes) and learn how to get them or visit us on [Discord](https://discord.gg/nhQehdv)</center>
post_id | 48,594,313 |
---|---|
author | qurator-tier-0 |
permlink | re-authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework-20180515t024206 |
category | utopian-io |
json_metadata | {} |
created | 2018-05-15 02:42:06 |
last_update | 2018-05-15 02:42:06 |
depth | 1 |
children | 1 |
net_rshares | 0 |
last_payout | 2018-05-22 02:42:06 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 377 |
author_reputation | 59,338,058,627 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Here we go again...
post_id | 48,600,454 |
---|---|
author | tensor |
permlink | re-qurator-tier-0-re-authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework-20180515t024206-20180515t033820116z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-05-15 03:38:21 |
last_update | 2018-05-15 03:38:21 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-05-22 03:38:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 19 |
author_reputation | 87,767,420,253,600 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Your contribution has been evaluated according to [Utopian rules and guidelines](https://utopian.io/rules), as well as a predefined set of questions pertaining to the category. To view those questions and the relevant answers related to your post,[Click here](https://review.utopian.io/result/9/112221122) Great work @tensor. ---- Need help? Write a ticket on https://support.utopian.io/. Chat with us on [Discord](https://discord.gg/uTyJkNm). [[utopian-moderator]](https://join.utopian.io/)
post_id | 48,628,025 |
---|---|
author | rosatravels |
permlink | re-tensor-authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework-20180515t080006889z |
category | utopian-io |
json_metadata | "{"links": ["https://utopian.io/rules", "https://review.utopian.io/result/9/112221122", "https://support.utopian.io/", "https://discord.gg/uTyJkNm", "https://join.utopian.io/"], "app": "steemit/0.1", "users": ["tensor"], "tags": ["utopian-io"]}" |
created | 2018-05-15 08:00:12 |
last_update | 2018-05-15 08:00:12 |
depth | 1 |
children | 1 |
net_rshares | 17,667,938,351 |
last_payout | 2018-05-22 08:00:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.063 SBD |
curator_payout_value | 0.021 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 499 |
author_reputation | 446,683,592,150,961 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
tensor | 0 | 17,667,938,351 | 100% |
As always, thank you for moderating my contribution.
post_id | 48,646,185 |
---|---|
author | tensor |
permlink | re-rosatravels-re-tensor-authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework-20180515t104445238z |
category | utopian-io |
json_metadata | "{"app": "steemit/0.1", "tags": ["utopian-io"]}" |
created | 2018-05-15 10:44:45 |
last_update | 2018-05-15 10:44:45 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-05-22 10:44:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 52 |
author_reputation | 87,767,420,253,600 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hey @tensor **Thanks for contributing on Utopian**. Weβre already looking forward to your next contribution! **Contributing on Utopian** Learn how to contribute on <a href='https://join.utopian.io'>our website</a> or by watching <a href='https://www.youtube.com/watch?v=8S1AtrzYY1Q'>this tutorial</a> on Youtube. **Want to chat? Join us on Discord https://discord.gg/h52nFrV.** <a href='https://v2.steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1'>Vote for Utopian Witness!</a>
post_id | 48,628,526 |
---|---|
author | utopian-io |
permlink | re-authenticating-users-with-google-sign-in-and-firebase-firestore-inside-of-dart-s-flutter-framework-20180515t080505z |
category | utopian-io |
json_metadata | "{"app": "beem/0.19.29"}" |
created | 2018-05-15 08:05:06 |
last_update | 2018-05-15 08:05:06 |
depth | 1 |
children | 0 |
net_rshares | 17,346,703,108 |
last_payout | 2018-05-22 08:05:06 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.063 SBD |
curator_payout_value | 0.021 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 503 |
author_reputation | 152,913,012,544,965 |
root_title | "Authenticating Users with Google Sign In and Firebase\Firestore inside of Dart's Flutter Framework" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
tensor | 0 | 17,346,703,108 | 100% |