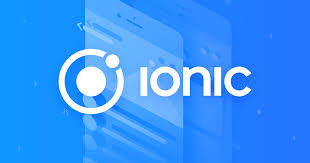 [Image Source](https://ionicframework.com/) **Repository**: [Ionic App Repository Github](https://github.com/ionic-team/ionic) **Software Requirements**: Visual Studio Code(Or any preferred code editor) Chrome(Or any preferred browser) **What you will learn**: In this tutorial you would learn how to live code a real ionic application with practical examples including these major concepts: - Developing a simple back-end for your application - Making HTTP requests in ionic - Managing data with the appropriate database **Difficulty**: Intermediate **Tutorial** So this tutorial is a continuation of the last in this series. And if we have a brief recap we would see that we were able to kick-start the development of a server end application for our ionic app. This server side would help us store data on a date base which in this case is MongoDb through HTTP requests made from the application. We were using postman for this tutorial to simulate server side requests, but in this tutorial we would use the ionic application directly and add json packaging. The first thing we'll be doing today is adding the data base to our server-side. To do this, we'll be using mlab. Quickly [Click this link](https://mlab.com/) and set up a free account to use to manage your database. Next thing you'll do is create a new database  When you create this database copy the url ![mlab 2.png] (https://cdn.steemitimages.com/DQmdJqyMKAgeZwWco4JUURqNBqYpJXsuZWEwErdoWdGqgS1/mlab%202.png) That is the url below. After this, create a new folder in the root directory of your folder and create a file called ``db.js`` In this file link your created database like this ``` module.exports = { url : YOUR URL HERE }; ``` Note: Replace the username and password areas with the ``username`` and ``password`` you used to create the mongoDb account. You then update your server.js file to include the mongoDb database were adding ``` const express = require('express');//All our earlier requirements const MongoClient = require('mongodb').MongoClient; const bodyParser = require('body-parser'); const db = require('./config/db');//This is the line that shows where we are referring to the database from. const app = express(); const port = 8000; app.use(bodyParser.urlencoded({ extended: true })); MongoClient.connect(db.url, (err, database) => { if (err) return console.log(err) require('./app/routes')(app, database); app.listen(port, () => { console.log('We are live on ' + port); }); }) ``` So lets analyze the code that helps us connect ``` MongoClient.connect(db.url, (err, database) => {//Here we create a database and use the database perculiar to our mongoDb url. if (err) return console.log(err) require('./app/routes')(app, database); app.listen(port, () => { console.log('We are live on ' + port); }); ``` Add this piece of code within your code if you are using a more recent version of mongoDb, which would be <3.0.0. Now we can go ahead to creating a collection. Collections are units of storage which mongoDb uses to store data in a adequately managed form that is easily accessible. To create a new note which we would be adding to our database we will use the ``post`` method. Since i am creating an app that deals with business transactions and stores the data from stores, i will make a collection called ``stores``. So let me start with a post method. The idea is that whenever you send a post request with '/stores', it will add a new store to the store database. Lets see how to do this. ``` module.exports = function(app, db) { const collection = app.post('/newStore', (req, res) => { const note = { text: req.body.body, title: req.body.title };//Well send requests where the title is the name of the store and the body is the contents of the store db.collection('store').insert(store, (err, result) => { if (err) { //We also need to check for errors and log them to our console. res.send({ 'error': 'An error has occurred' }); } else { res.send(result.ops[0]); } }); }); }; ``` So now lets try using this from our ionic services. The first thing we'll do is run our server. To do this head to your command line and run ``` npm run dev ``` This would run a development server and be ready to listen to any requests from our app. So head to the provider for your app and lets code In our ``store.ts`` file, we will make our requests using the code below ``` import { HttpClient } from '@angular/common/http'; import { Injectable } from '@angular/core'; @Injectable() export class StoreProvider { store: string; constructor(public http: HttpClient) { this.store = 'My Store'; } createNewUser(){ this.http.post( 'localhost:8000, {userDetails}//This would be an object containing the array of the details collected which are required to create a new user {}//At this point we would not send any header. } login(){ //This is where we would send a request to get the id for this particular user } //Ignore this function for now /*addToStore(item){ this.http.put(/This is how we would send the request to add a product to this user 'localhost:8000/createStore', {item}, {} )*/ } } ``` So as seen above we have created the first function in our service to create a new user. You could try this out by importing this service into a dummy page and creating a simple interface that collects some data. When you do this create a button that will trigger this service and pass data to your database. To import a service into a page, simply; ``` import { StoreProvider} from '..providers/stroreprovider' constructor(public store: StoreProvider){ addToStore(){ //use it here like this this.store.createNewUser } ``` So thats it for today, You can see the prototype document i am using to create this app from my repository [Here](www.github.com/yalzeee)
post_id | 64,709,641 |
---|---|
author | yalzeee |
permlink | tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2 |
category | utopian-io |
json_metadata | {"format":"markdown","tags":["utopian-io","tutorials","programming","stach","wafrica"],"app":"steemit\/0.1","image":["https:\/\/cdn.steemitimages.com\/DQmS4ho2iWke6Uc4wmNdSTD2GCtYJZFb7rRzMD2LLV49Dqs\/download.jpeg"],"links":["https:\/\/ionicframework.com\/","https:\/\/github.com\/ionic-team\/ionic","https:\/\/mlab.com\/","www.github.com\/yalzeee"]} |
created | 2018-10-22 12:37:18 |
last_update | 2018-10-22 12:37:18 |
depth | 0 |
children | 8 |
net_rshares | 5,948,017,941,500 |
last_payout | 2018-10-29 12:37:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 5.318 SBD |
curator_payout_value | 1.568 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 6,192 |
author_reputation | 12,492,997,891,187 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
azizbd | 0 | 41,500,107,514 | 40% | ||
miniature-tiger | 0 | 156,253,488,792 | 100% | ||
anouk.nox | 0 | 7,492,657,658 | 25% | ||
kymaticus | 0 | 497,024,066 | 100% | ||
rafalski | 0 | 40,372,816,079 | 100% | ||
followforupvotes | 0 | 4,689,015,941 | 9% | ||
fbslo | 0 | 71,254,254,252 | 25% | ||
espoem | 0 | 10,584,449,803 | 7.25% | ||
nmalove | 0 | 408,938,759 | 3.41% | ||
utopian-io | 0 | 5,063,848,181,951 | 3.49% | ||
shammi | 0 | 70,401,415,813 | 75% | ||
ayasha | 0 | 14,594,210,655 | 32% | ||
greenorange | 0 | 551,769,017 | 100% | ||
amosbastian | 0 | 36,406,893,825 | 18.13% | ||
resteemator | 0 | 248,918,603 | 8% | ||
yalzeee | 0 | 2,183,919,902 | 100% | ||
sudefteri | 0 | 2,604,824,024 | 100% | ||
erikklok | 0 | 12,034,991,921 | 100% | ||
reazuliqbal | 0 | 12,202,079,154 | 20% | ||
properfraction | 0 | 698,553,993 | 100% | ||
lordjames | 0 | 1,328,329,770 | 3.41% | ||
hakancelik | 0 | 20,116,328,913 | 30% | ||
codebull | 0 | 228,459,630 | 52% | ||
mhossain | 0 | 488,665,071 | 40% | ||
sheikhsayem | 0 | 847,320,099 | 40% | ||
wafrica | 0 | 39,629,411,852 | 6.83% | ||
coinsandchains | 0 | 3,840,792,732 | 8.15% | ||
linco | 0 | 13,504,719,295 | 40% | ||
drsensor | 0 | 11,430,548,665 | 100% | ||
zayedsakib | 0 | 8,836,080,489 | 40% | ||
steem-ua | 0 | 293,736,843,393 | 2.28% | ||
ilovecoding | 0 | 795,556,881 | 10% | ||
ecodesigns | 0 | 432,591,310 | 3.41% | ||
curbot | 0 | 3,973,781,678 | 10% |
Hello! Your post has been resteemed and upvoted by @ilovecoding because **we love coding**! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!  *Reply !stop to disable the comment. Thanks!*
post_id | 64,709,651 |
---|---|
author | ilovecoding |
permlink | 20181022t123730043z |
category | utopian-io |
json_metadata | {"tags":["ilovecoding"],"app":"ilovecoding"} |
created | 2018-10-22 12:37:30 |
last_update | 2018-10-22 12:37:30 |
depth | 1 |
children | 0 |
net_rshares | 371,941,440 |
last_payout | 2018-10-29 12:37:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 323 |
author_reputation | 40,842,386,526 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
ilovecoding | 0 | 371,941,440 | 5% |
As a follower of @followforupvotes this post has been randomly selected and upvoted! Enjoy your upvote and have a great day!
post_id | 64,710,467 |
---|---|
author | followforupvotes |
permlink | re-yalzeee-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181022t125318148z |
category | utopian-io |
json_metadata | {} |
created | 2018-10-22 12:53:21 |
last_update | 2018-10-22 12:53:21 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-10-29 12:53:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 124 |
author_reputation | 24,990,662,983,063 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hello! I find your post valuable for the wafrica community! Thanks for the great post! We encourage and support quality contents and projects from the West African region.<br>Do you have a suggestion, concern or want to appear as a guest author on WAfrica, join our [discord server](https://discord.gg/AfFUhnC) and discuss with a member of our curation team.<br>Don't forget to join us every Sunday by 20:30GMT for our Sunday WAFRO party on our [discord channel](https://discord.gg/AfFUhnC). Thank you.
post_id | 64,712,768 |
---|---|
author | wafrica |
permlink | re-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181022t133629 |
category | utopian-io |
json_metadata | {} |
created | 2018-10-22 13:36:30 |
last_update | 2018-10-22 13:36:30 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-10-29 13:36:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 503 |
author_reputation | 38,213,975,622,361 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
I thank you for your contribution. Here are my thoughts. Note that, my thoughts are my personal ideas on your post and they are not directly related to the review and scoring unlike the answers I gave in the questionnaire; * **Structure** * I advise you to consider using paragraphs. Separating each sentence with a blank line makes it harder to read and understand. * It's hard to figure out which part does what. So, I advise you using headers to categorize the post. You talked about 3 major concepts at the beginning. Simply writing each with a header would make it easier to read. * **Language** * Some of your sentences are artificial. This makes them hard to read and understand. To overcome that, I advise you to check the structure of your sentences. For example, using "we"/"you" for each sentence in the post makes it boring to read. Here is an example sentence: * "The first thing we'll be doing today is adding the data base to our server-side." Instead of the one above, you can use the below one. * "First part of this tutorial is adding a database to server-side." As you can see, removing persons from the sentence made it look more natural. You can improve it by rewriting sentences after doing this and repeating the same process if necessary. * There are some sentences which are hard to understand because of the vocabulary and grammar. I advise you to proofread your posts before posting. It can increase the efficiency of your post. * **Content** * What you really have shown is doing it by yourself, not teaching. I advise you to change this approach to increase teaching efficiency. Here is an example: * "After this, create a new folder in the root directory of your folder and create a file called db.js In this file link your created database like this" This sentence just says what to do, so it reduces the efficiency by implying user that the way you show is the only way to accomplish that. ---- Your contribution has been evaluated according to [Utopian policies and guidelines](https://join.utopian.io/guidelines), as well as a predefined set of questions pertaining to the category. To view those questions and the relevant answers related to your post, [click here](https://review.utopian.io/result/8/22313444). ---- Need help? Write a ticket on https://support.utopian.io/. Chat with us on [Discord](https://discord.gg/uTyJkNm). [[utopian-moderator]](https://join.utopian.io/)
post_id | 64,785,773 |
---|---|
author | yokunjon |
permlink | re-yalzeee-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181023t153807372z |
category | utopian-io |
json_metadata | {"app":"steemit\/0.1","tags":["utopian-io"],"links":["https:\/\/join.utopian.io\/guidelines","https:\/\/review.utopian.io\/result\/8\/22313444","https:\/\/support.utopian.io\/","https:\/\/discord.gg\/uTyJkNm","https:\/\/join.utopian.io\/"]} |
created | 2018-10-23 15:38:09 |
last_update | 2018-10-23 15:38:09 |
depth | 1 |
children | 2 |
net_rshares | 6,756,482,076,755 |
last_payout | 2018-10-30 15:38:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 5.880 SBD |
curator_payout_value | 1.887 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 2,482 |
author_reputation | 14,981,508,863,374 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yuxi | 0 | 8,626,348,564 | 30% | ||
mys | 0 | 20,002,897,637 | 16.1% | ||
pixelfan | 0 | 1,229,442,561 | 0.6% | ||
jadabug | 0 | 527,740,947 | 2.5% | ||
utopian-io | 0 | 6,649,668,322,230 | 4.6% | ||
emrebeyler | 0 | 43,699,466,670 | 3% | ||
amosbastian | 0 | 16,972,008,028 | 8.72% | ||
oups | 0 | 2,206,962,275 | 4% | ||
indianteam | 0 | 36,787,863 | 8.72% | ||
hakancelik | 0 | 13,512,099,980 | 20% |
Thanks for the tips@yokunjon I really appreciate the personal suggestions, already made a new post before i saw this but would surely implement this is the next tutorial i'm going to create.
post_id | 64,788,179 |
---|---|
author | yalzeee |
permlink | re-yokunjon-re-yalzeee-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181023t162210636z |
category | utopian-io |
json_metadata | {"app":"steemit\/0.1","tags":["utopian-io"]} |
created | 2018-10-23 16:22:12 |
last_update | 2018-10-23 16:22:12 |
depth | 2 |
children | 0 |
net_rshares | 12,637,449,642 |
last_payout | 2018-10-30 16:22:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 190 |
author_reputation | 12,492,997,891,187 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yalzeee | 0 | 2,629,745,518 | 100% | ||
yokunjon | 0 | 10,007,704,124 | 100% |
Thank you for your review, @yokunjon! So far this week you've reviewed 2 contributions. Keep up the good work!
post_id | 65,024,423 |
---|---|
author | utopian-io |
permlink | re-re-yalzeee-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181023t153807372z-20181027t164521z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.9"} |
created | 2018-10-27 16:45:24 |
last_update | 2018-10-27 16:45:24 |
depth | 2 |
children | 0 |
net_rshares | 11,355,023,575 |
last_payout | 2018-11-03 16:45:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 111 |
author_reputation | 152,913,012,544,965 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yokunjon | 0 | 11,355,023,575 | 100% |
#### Hi @yalzeee! Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation! Your post is eligible for our upvote, thanks to our collaboration with @utopian-io! **Feel free to join our [@steem-ua Discord server](https://discord.gg/KpBNYGz)**
post_id | 64,787,957 |
---|---|
author | steem-ua |
permlink | re-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181023t161832z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.7"} |
created | 2018-10-23 16:18:33 |
last_update | 2018-10-23 16:18:33 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-10-30 16:18:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 286 |
author_reputation | 23,203,609,903,979 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hey, @yalzeee! **Thanks for contributing on Utopian**. Weβre already looking forward to your next contribution! **Get higher incentives and support Utopian.io!** Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via [SteemPlus](https://chrome.google.com/webstore/detail/steemplus/mjbkjgcplmaneajhcbegoffkedeankaj?hl=en) or [Steeditor](https://steeditor.app)). **Want to chat? Join us on Discord https://discord.gg/h52nFrV.** <a href='https://steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1'>Vote for Utopian Witness!</a>
post_id | 65,062,815 |
---|---|
author | utopian-io |
permlink | re-tutorial-ionic-tutorials-making-http-request-and-developing-a-backend-for-our-ionic-application-part-2-20181028t115012z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.9"} |
created | 2018-10-28 11:50:12 |
last_update | 2018-10-28 11:50:12 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-11-04 11:50:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 589 |
author_reputation | 152,913,012,544,965 |
root_title | "[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |