import java.util.*; public class Satellite { private Node buildTree(int preId, List<Character> preorderInput, List<Character> inorderInput, int startId, int endId){ if(startId > endId) return null; Node local = new Node(preorderInput.get(preId)); if(startId == endId) return local; int index = startId; for(int i=startId; i<= endId;i++){ if(local.value == inorderInput.get(i)) index = i; } local.left = buildTree(++preId,preorderInput, inorderInput, startId, index-1); local.right = buildTree(++preId,preorderInput, inorderInput, index+1, endId); return local; } public Tree treeFromTraversals(List<Character> preorderInput, List<Character> inorderInput) { if(preorderInput.size() != inorderInput.size()) throw new IllegalArgumentException("traversals must have the same length"); Set<Character> setVer = new HashSet<>(preorderInput); if(setVer.size() != preorderInput.size()) throw new IllegalArgumentException("traversals must contain unique items"); // equals per verificare che due set abbiano gli stessi elmenti. Set<Character> setVerIn = new HashSet<>(inorderInput); if(!setVer.equals(setVerIn)) throw new IllegalArgumentException("traversals must have the same elements"); return new Tree(buildTree(0,preorderInput,inorderInput,0,preorderInput.size()-1)); } }
In the realm of live streaming, open-source solutions have emerged as powerful tools, offering flexibility, customization, and freedom from proprietary restrictions. Open source live streaming software empowers users to create, broadcast, and share live video content on their terms, fostering innovation and collaboration within the streaming community. In this guide, we delve into the world of open source live streaming, exploring its benefits, features, and popular software options. Understanding Open Source Live Streaming: Open source live streaming refers to the use of software with source code that is freely available for modification and redistribution by users. Unlike proprietary solutions, which are owned and controlled by a single entity, open source software encourages collaboration, transparency, and community-driven development. Key Features of Open Source Live Streaming Software: Flexibility and Customization: Open source live streaming software offers unparalleled flexibility, allowing users to modify, extend, and customize the software according to their specific needs and preferences. This enables users to tailor the software to suit their unique streaming requirements, whether for gaming, events, education, or business. Cost-Effectiveness: Open source software is typically available for free, eliminating the need for costly licensing fees or subscriptions. This makes <a href="https://www.red5.net/open-source-live-streaming/">open source live streaming</a> solutions a cost-effective option for individuals, organizations, and businesses looking to launch or scale their live streaming initiatives without breaking the bank. Community Support and Collaboration: The open source community fosters collaboration and knowledge sharing among developers, users, and enthusiasts. Users can benefit from community forums, documentation, tutorials, and user-contributed plugins and extensions, enhancing the functionality and usability of open source live streaming software. Transparency and Security: With open source software, the source code is publicly accessible, allowing users to inspect, audit, and verify the software's security and integrity. This transparency helps build trust and confidence among users and ensures that the software meets their security and privacy requirements. Platform Independence: Open source live streaming software is often platform-independent, meaning it can run on a wide range of operating systems and hardware configurations. This flexibility allows users to choose the platform that best suits their needs, whether it's Windows, macOS, Linux, or a cloud-based solution. Benefits of Open Source Live Streaming: Freedom from Vendor Lock-In: Open source live streaming software liberates users from vendor lock-in, giving them full control over their streaming infrastructure and workflows. Users are free to choose, modify, and switch between different software solutions without being tied to a single vendor. Innovation and Experimentation: The open source model encourages innovation and experimentation, driving continuous improvement and evolution of live streaming technology. Users can contribute to the development of new features, improvements, and optimizations, shaping the future of live streaming for the entire community. Community Engagement and Support: The open source community provides a vibrant ecosystem of developers, users, and contributors who actively collaborate, share knowledge, and support one another. Users can leverage community resources, forums, and channels to seek help, share ideas, and collaborate on projects, fostering a sense of belonging and camaraderie. Scalability and Performance: Open source live streaming software is designed to scale with the needs of users, whether they're streaming to a small audience or a global audience of millions. Users can deploy the software on their own infrastructure or leverage cloud-based solutions for scalability, reliability, and performance. Privacy and Data Ownership: With open source software, users retain full control over their data and content, ensuring privacy and data ownership. Unlike proprietary solutions that may collect user data for marketing or analytics purposes, open source live streaming software respects user privacy and autonomy. Popular Open Source Live Streaming Software: OBS Studio (Open Broadcaster Software): OBS Studio is a free and open-source live streaming and recording software known for its powerful features, customization options, and multi-platform support. Streamlabs OBS: Streamlabs OBS is a user-friendly live streaming software tailored for content creators and streamers, offering built-in tools for audience engagement, monetization, and analytics. FFmpeg: FFmpeg is a versatile multimedia framework that includes a suite of tools for encoding, decoding, transcoding, and streaming audio and video content. XSplit Broadcaster: XSplit Broadcaster is a live streaming and recording software with advanced features for gamers, content creators, and businesses, including scene transitions, chroma keying, and virtual sets. nginx-rtmp-module: nginx-rtmp-module is an open-source module for the nginx web server that enables live streaming and video-on-demand (VOD) delivery using the Real-Time Messaging Protocol (RTMP) and HTTP Live Streaming (HLS) protocols. Conclusion: Open source live streaming software empowers users with freedom, flexibility, and control over their live streaming workflows. With features such as customization, community support, and cost-effectiveness, open source solutions offer a compelling alternative to proprietary software for individuals, organizations, and businesses looking to harness the power of live video broadcasting. Whether it's for gaming, events, education, or business <a href="https://www.red5.net/open-source-live-streaming/">open source live streaming</a> software provides the tools and resources needed to create compelling live content and engage audiences in real-time.
import java.util.HashSet; public class PangramChecker { public boolean isPangram(String input) { HashSet<Character> letters = new HashSet<>(); for(char letter: input.toLowerCase().toCharArray()){ if(Character.isLetter(letter)){ letters.add(letter); } } return letters.size() >=26; } }
// @ts-check // // ☝🏽 The line above enables type checking for this file. Various IDEs interpret // the @ts-check directive. It will give you helpful autocompletion on the web // and supported IDEs when implementing this exercise. You don't need to // understand types, JSDoc, or TypeScript in order to complete this JavaScript // exercise, and can completely ignore this comment block and directive. // // 👋🏽 Hi again! // // A quick reminder about exercise stubs: // // 💡 You're allowed to completely clear any stub before you get started. Often // we recommend using the stub, because they are already set-up correctly to // work with the tests, which you can find in ./door-policy.spec.js. // // 💡 You don't need to write JSDoc comment blocks yourself; it is not expected // in idiomatic JavaScript, but some companies and style-guides do enforce them. // // Good luck with that door policy! /** * Respond with the correct character, given the line of the * poem, if this were said at the front door. * * @param {string} line * @returns {string} */ export function frontDoorResponse(line) { return line[0]; } /** * Format the password for the front-door, given the response * letters. * * @param {string} word the letters you responded with before * @returns {string} the front door password */ export function frontDoorPassword(word) { return word[0].toUpperCase()+word.slice(1,word.length).toLowerCase(); } /** * Respond with the correct character, given the line of the * poem, if this were said at the back door. * * @param {string} line * @returns {string} */ export function backDoorResponse(line) { return line.trim().slice(-1); } /** * Format the password for the back door, given the response * letters. * * @param {string} word the letters you responded with before * @returns {string} the back door password */ export function backDoorPassword(word) { return frontDoorPassword(word)+', please'; }
Hallo Leute. Heute kam mal eine richtig gute Nachricht in Sachen schönes Festival. Wir fahren dieses Jahr auf jeden Fall wieder auf das Ruhrpott Rodeo Festival in Hünxe bei Bottrop am Flughafen Schwarze Heide. Das Festival hat heute eine neue Band bestätigt. Und das sind die grandiosen ### NAPALM DEATH # Das ist mal eine gute Nachricht. Habt alle einen schönen Tag. Gruß vom @bitandi 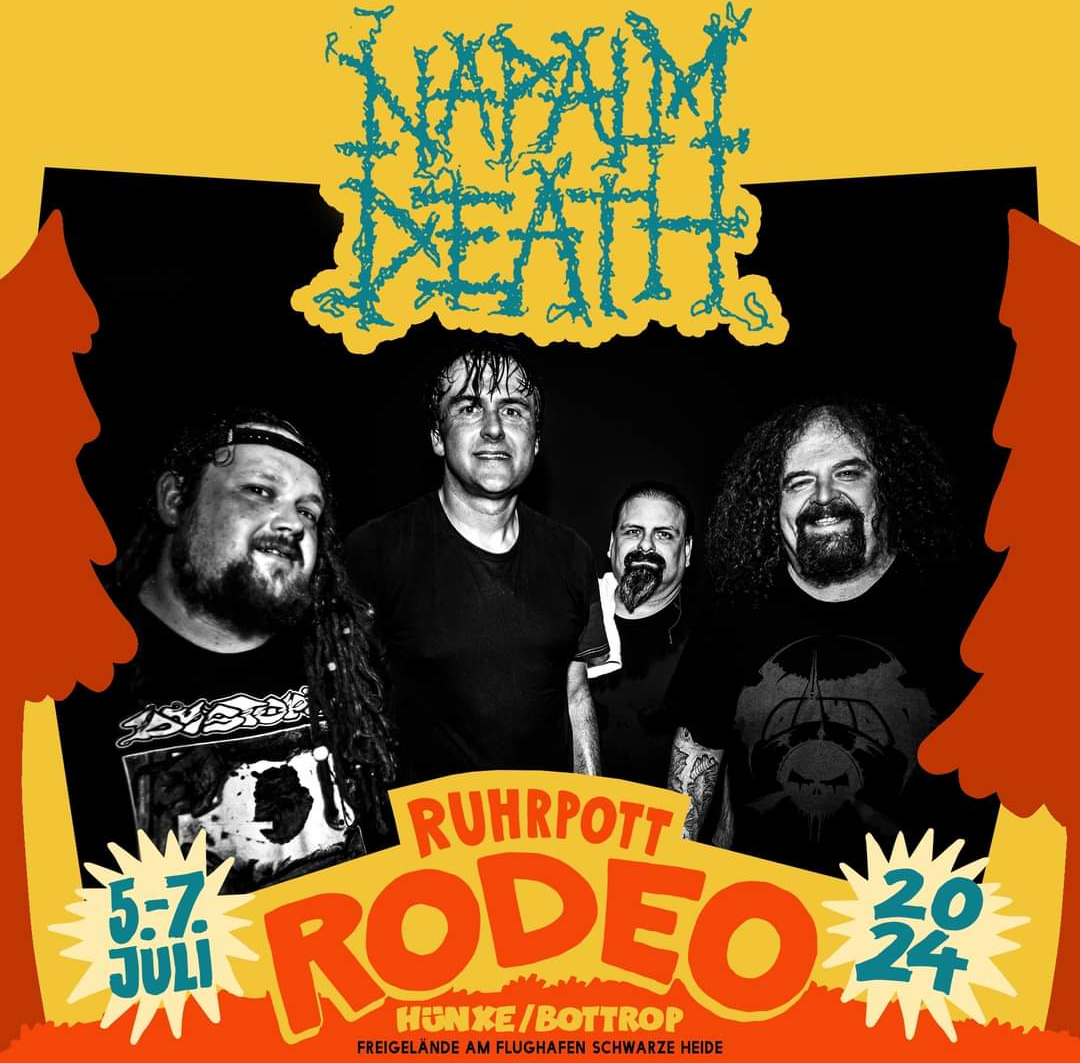
function wrap(text, tag) {return `<${tag}>${text}</${tag}>`;} function isTag(text, tag) {return text.startsWith(`<${tag}>`);} function parser(markdown, delimiter, tag) {return markdown.replace(new RegExp(`${delimiter}(.+)${delimiter}`), `<${tag}>$1</${tag}>`);} function parse__(markdown) {return parser(markdown, '__', 'strong');} function parse_(markdown) {return parser(markdown, '_', 'em');} function parseText(markdown, list) {if (list) {return parse_(parse__(markdown));} else {return wrap(parse_(parse__(markdown)), 'p');}} function parseHeader(markdown, list) { let count = 0; for (let i = 0; i < markdown.length; i++) { if(markdown[i] === '#'){count += 1;} else {break;} } if (count === 0 || count > 6) {return [null, list]; } const headerHtml = wrap(markdown.substring(count + 1), `h${count}`); return list? [`</ul>${headerHtml}`, false]: [headerHtml, false]; } function parseLineItem(markdown, list) { if (markdown.startsWith('*')) { const innerHtml = wrap(parseText(markdown.substring(2), true), 'li'); return (list) ? [innerHtml, true]:[`<ul>${innerHtml}`, true]; } return [null, list]; } function parseParagraph(markdown, list) {return (!list)? [parseText(markdown, false), false]: [`</ul>${parseText(markdown, false)}`, false];} function parseLine(markdown, list) { let [result, inListAfter] = parseHeader(markdown, list); if (result === null) {[result, inListAfter] = parseLineItem(markdown, list);} if (result === null) {[result, inListAfter] = parseParagraph(markdown, list);} if(result === null) {throw new Error('Invalid markdown');} return [result, inListAfter]; } export function parse(markdown) { const lines = markdown.split('\n'); let result = ''; let list = false; for (let i = 0; i < lines.length; i++) { let [lineResult, newList] = parseLine(lines[i], list); result += lineResult; list = newList; } return (list)? result + '</ul>': result; }
public class ElonsToyCar { private int distance = 0; private int battery = 100; public static ElonsToyCar buy() { ElonsToyCar car = new ElonsToyCar(); return car; } public String distanceDisplay() { return "Driven " +distance + " meters"; } public String batteryDisplay() { return battery==0 ? "Battery empty":"Battery at " + battery+ "%"; } public void drive() { if(battery!=0) { battery -= 1; distance +=20; } } }
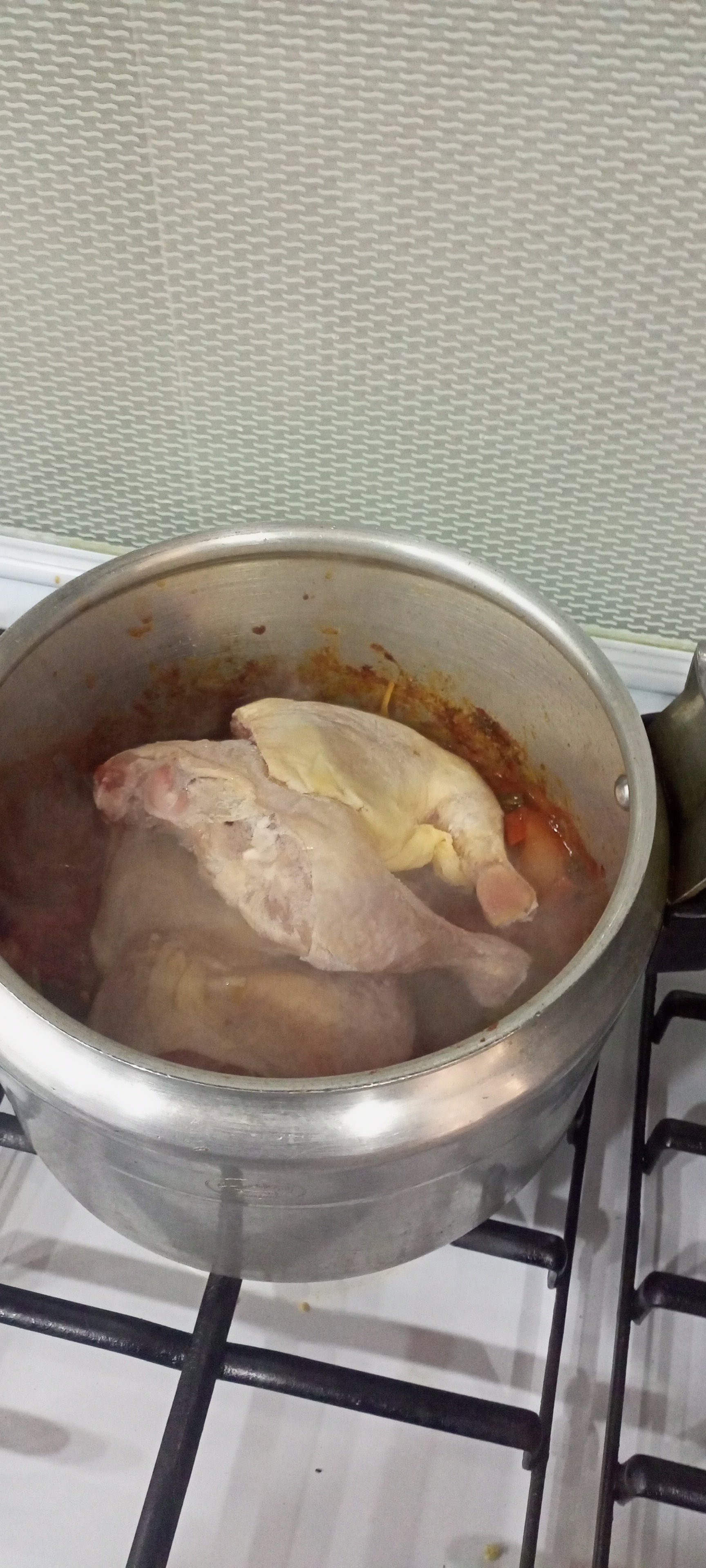
class CalculatorConundrum { public String calculate(int operand1, int operand2, String operation) { if (operation == null) {throw new IllegalArgumentException ("Operation cannot be null");} if(operation.equals("")) {throw new IllegalArgumentException ("Operation cannot be empty");} double total =0; try{switch(operation){ case "+": total = operand1+operand2; break; case "-": total = operand1-operand2; break; case "*": total = operand1*operand2; break; case "/": total = operand1/operand2; break; default: throw new IllegalOperationException (String.format("Operation '%s' does not exist", operation)); } } catch(ArithmeticException e){throw new IllegalOperationException("Division by zero is not allowed",e);} return String.format("%s %s %s = %d", operand1, operation, operand2, (int)total); } }
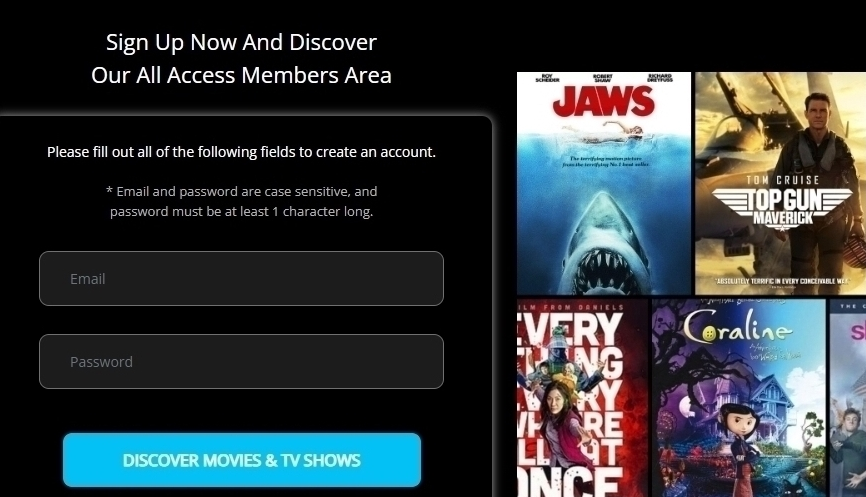 **Ingrese su información ahora para comenzar. (EC) Ecuador Desktop Credit Card Submit** **Desktop Credit Card Submit: https://singingfiles.com/show.php?l=0&u=1997254&id=64084**
class ScrabbleScore { static scoreWord(String word) { int total = 0 String words = word.toUpperCase() for(char letter in words){ switch(letter){ case "A": case "E": case "I": case "O": case "U": case "L": case "N": case "R": case "S": case "T" : total +=1 break case "D": case "G" : total +=2 break case "B": case "C" : case "M": case "P" : total +=3 break case "F": case "H" : case "V": case "W": case "Y": total +=4 break case "K": total +=5 break case "J": case "X" : total +=8 break case "Q": case "Z": total +=10 break } } return total; } }
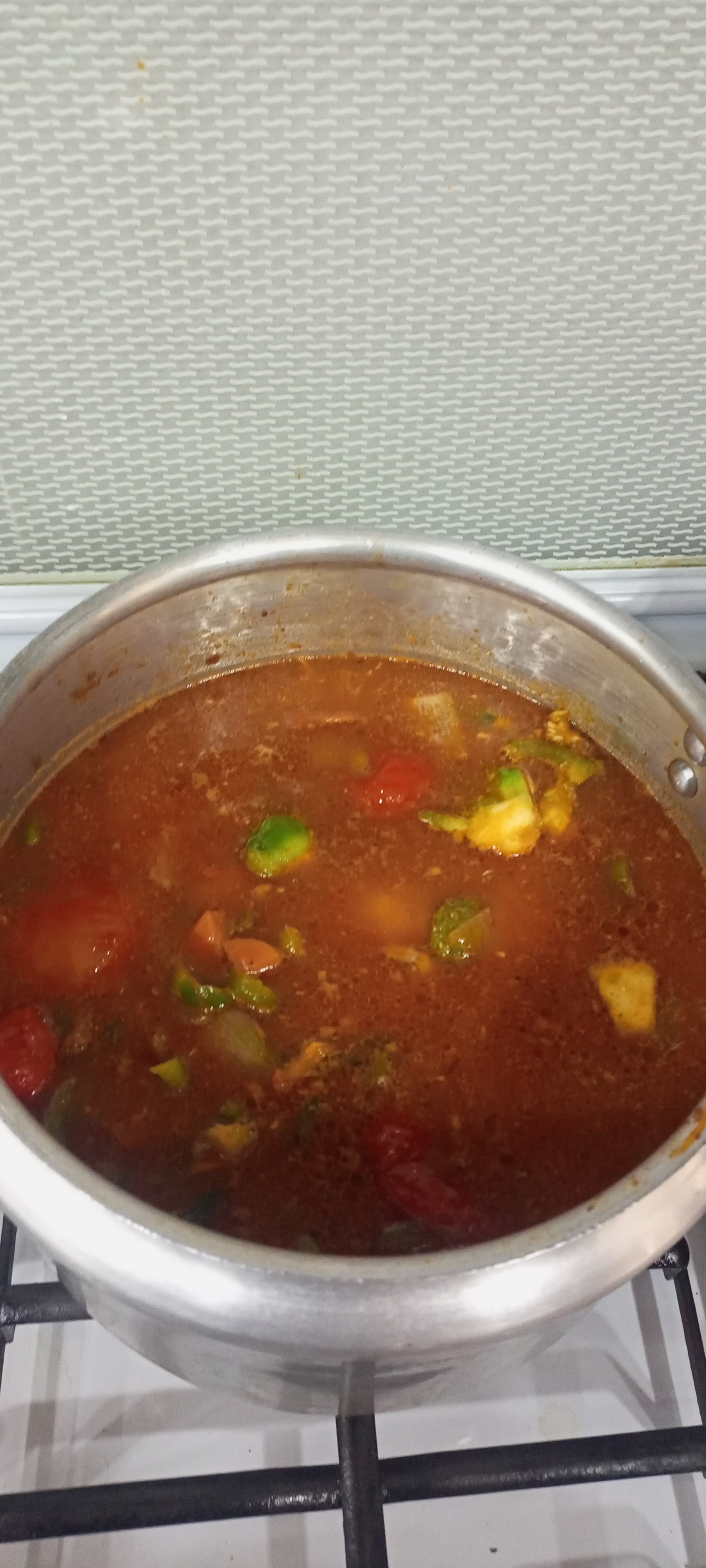
def square_of_sum(number): square = 0 for i in range(number+1): square += i return square ** 2 def sum_of_squares(number): sum = 0 for i in range(number+1): sum += i*i return sum def difference_of_squares(number): return square_of_sum(number) - sum_of_squares(number)
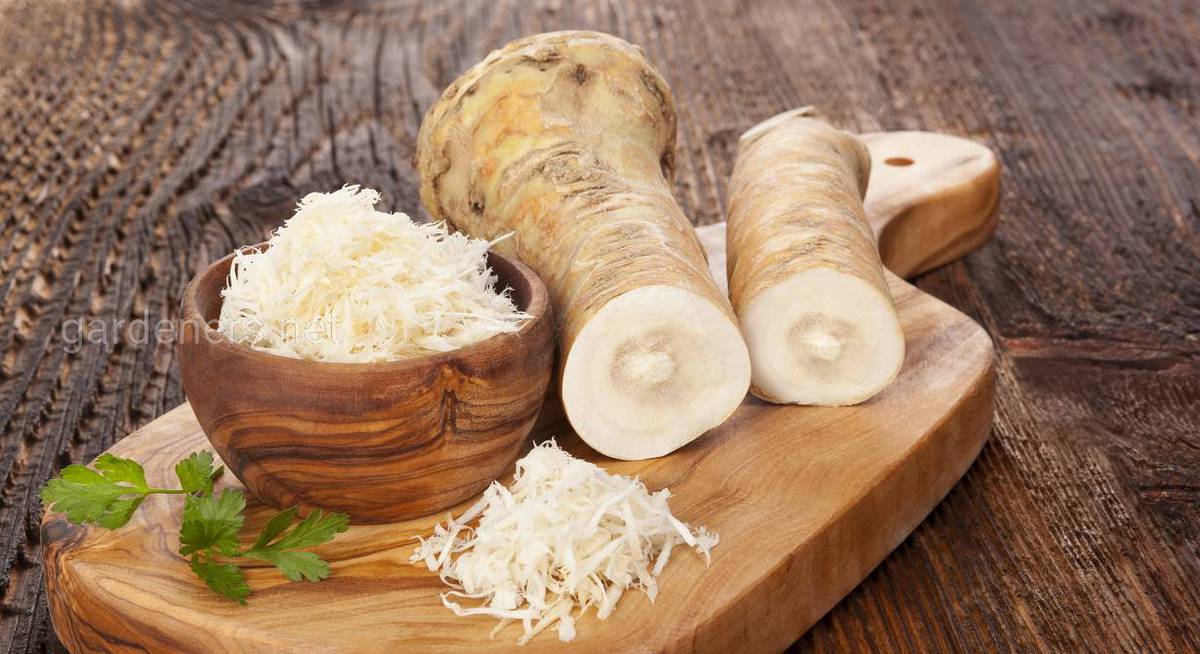 Chrzan pospolity (Armoracia rusticana) to znana od wieków roślina, ceniona nie tylko za swój ostry smak i aromat, ale również za liczne właściwości zdrowotne. Uprawiany w wielu zakątkach świata, chrzan zyskał miano "polskiego skarbu", a jego korzeń stanowi cenny dodatek do wielu potraw. Co kryje się w ostrym korzeniu chrzanu? Oto niektóre z jego cennych właściwości: Właściwości przeciwnowotworowe: Chrzan bogaty jest w glukozynolany i izotiocyjaniany, związki o potencjalnym działaniu antynowotworowym. Mogą one hamować wzrost komórek nowotworowych i wspomagać terapię przeciwnowotworową. Działanie przeciwutleniające: Chrzan zawiera silne przeciwutleniacze, które zwalczają wolne rodniki, chroniąc komórki organizmu przed uszkodzeniami i stresem oksydacyjnym. To działanie może zmniejszać ryzyko wielu chorób, w tym chorób serca, nowotworów i chorób neurodegeneracyjnych. Wsparcie układu odpornościowego: Chrzan bogaty jest w witaminę C i inne składniki odżywcze, które wzmacniają układ odpornościowy i pomagają organizmowi zwalczać infekcje. Poprawa trawienia: Chrzan pobudza wydzielanie soków żołądkowych i żółci, co ułatwia trawienie i wchłanianie pokarmów. Może również łagodzić dolegliwości trawienne, takie jak niestrawność, wzdęcia i zaparcia. Działanie antybakteryjne i przeciwwirusowe: Chrzan wykazuje właściwości antybakteryjne i przeciwwirusowe, które mogą być pomocne w zwalczaniu infekcji bakteryjnych i wirusowych. Właściwości przeciwzapalne: Chrzan zawiera związki o działaniu przeciwzapalnym, które mogą łagodzić ból i stan zapalny stawów, mięśni i innych tkanek. Detoksykacja organizmu: Chrzan wspomaga detoksykację organizmu poprzez stymulowanie pracy wątroby i nerek. Pomaga usuwać z organizmu szkodliwe toksyny i produkty przemiany materii. Inne właściwości: Chrzan może również korzystnie wpływać na krążenie krwi, obniżać poziom cholesterolu i regulować poziom cukru we krwi. Sposoby wykorzystania chrzanu: Chrzan można jeść na surowo, dodając go do kanapek, sałatek i sosów. Świetnie sprawdza się również jako dodatek do mięs, ryb i wędlin. Z chrzanu można również przygotować syrop, ocet chrzanowy, pastę chrzanową i wiele innych pysznych i zdrowych produktów. Należy jednak pamiętać, że chrzan jest rośliną ostrą i spożywany w nadmiarze może podrażniać żołądek i jelita. Osoby z chorobami żołądka, jelit, wątroby lub nerek powinny skonsultować się z lekarzem przed spożyciem chrzanu. Chrzan to nie tylko smaczny dodatek do potraw, ale również cenny dar natury o wielu właściwościach zdrowotnych. Warto włączyć go do swojej diety, aby skorzystać z jego dobroczynnych właściwości.
Shiitake, czyli twardnik japoński to gatunek grzyba jadalnego, rozpowszechniony w Chinach, Japonii i Korei. Ze względu na swoją wartość konsumpcyjną i zdrowotną staje się obecnie coraz popularniejszy w Europie. Jego uprawa nie nastręcza dużych trudności i udaje się nawet na wolnym powietrzu.Związki zawarte w shiitake mogą pomóc w walce z rakiem, wzmocnić odporność i wspierać zdrowie serca. Ten artykuł wyjaśnia wszystko, co musisz wiedzieć o grzybach shiitake. Czym są grzyby shiitake? Shiitake to jadalne grzyby pochodzące z Azji Wschodniej. Są od brązowego do ciemnobrązowego, z kapeluszami, które rosną od 5 do 10 cm. Chociaż zwykle je się je jak warzywa, shiitake to grzyby, które naturalnie rosną na rozkładających się drzewach liściastych. Około 83% shiitake uprawia się w Japonii, chociaż produkują je również Stany Zjednoczone, Kanada, Singapur i Chiny. Można je znaleźć świeże, suszone lub w różnych suplementach diety. Zdrowe serce Grzyby shiitake mogą poprawić prawidłową kondycje serca . Na przykład mają trzy związki, które pomagają obniżyć poziom cholesterolu (link ↗)(link ↗)(link ↗) Eritadenina. Związek ten hamuje enzym zaangażowany w produkcję cholesterolu. Sterole. Te cząsteczki pomagają blokować wchłanianie cholesterolu w jelitach. Beta glukany. Ten rodzaj błonnika może obniżyć poziom cholesterolu . Jedno badanie (link ↗) na szczurach z wysokim ciśnieniem krwi wykazało, że proszek shiitake zapobiegał wzrostowi ciśnienia krwi .Badanie (link ↗)na szczurach laboratoryjnych karmionych dietą wysoko tłuszczową wykazało, że te, którym podano shiitake, rozwinęły mniej tłuszczu w wątrobie, mniej płytki nazębnej na ścianach tętnic i niższy poziom cholesterolu niż te, które nie jadły żadnych grzybów . Jednak efekty te muszą zostać potwierdzone w badaniach na ludziach, zanim można będzie wyciągnąć jakiekolwiek solidne wnioski. Może wzmocnić układ odpornościowy Shiitake może również pomóc wzmocnić układ odpornościowy. Jedno z badań (link ↗)..dawano ludziom dwa suszone shiitake dziennie. Po miesiącu ich markery odpornościowe poprawiły się, a ich stan zapalny spadł. Ten efekt immunologiczny może być częściowo spowodowany jednym z polisacharydów w grzybach shiitake (link ↗). Podczas gdy układ odpornościowy ludzi słabnie wraz z wiekiem , badanie na myszach wykazało, że suplement pochodzący z shiitake pomógł odwrócić pewien związany z wiekiem spadek funkcji odpornościowych (link ↗). Zawierają związki o potencjalnym działaniu przeciwnowotworowym Polisacharydy w grzybach shiitake mogą również mieć działanie przeciwnowotworowe (link ↗). (link ↗).Na przykład polisacharyd lentinan pomaga zwalczać nowotwory poprzez aktywację układu odpornościowego (link ↗). (link ↗). Wykazano, że lentinan hamuje wzrost i rozprzestrzenianie się komórek białaczkowych(link ↗) . W Chinach i Japonii wstrzykiwaną formę lentinanu stosuje się wraz z chemioterapią i innymi poważnymi metodami leczenia raka w celu poprawy funkcji odpornościowej i jakości życia u osób z rakiem żołądka (link ↗)(link ↗) .Jednak dowody są niewystarczające, aby ustalić, czy jedzenie grzybów shiitake ma jakikolwiek wpływ na raka. Działanie przeciwbakteryjne i przeciwwirusowe Kilka związków zawartych w shiitake ma działanie przeciwbakteryjne, przeciwwirusowe i przeciwgrzybicze (link ↗) Ponieważ oporność na antybiotyki rośnie, niektórzy naukowcy uważają, że ważne jest zbadanie potencjału przeciwdrobnoustrojowego shiitake(link ↗) . Może wzmocnić twoje kości Grzyby są jedynym naturalnym roślinnym źródłem witaminy D. Twoje ciało potrzebuje witaminy D do budowy mocnych kości, ale bardzo niewiele produktów spożywczych zawiera ten ważny składnik odżywczy. Poziomy witaminy D w grzybach różnią się w zależności od sposobu ich uprawy. Pod wpływem światła UV rozwijają się wyższe poziomy tego związku. W jednym z badań u myszy karmionych dietą o niskiej zawartości wapnia i witaminy D wystąpiły objawy osteoporozy. Dla porównania, osoby, którym podano shiitake wzmocnione wapniem i promieniowaniem UV, miały wyższą gęstość kości (link ↗) .Należy jednak pamiętać, że shiitake dostarczają witaminę D2. Jest to gorsza forma w porównaniu z witaminą D3, którą można znaleźć w tłustych rybach i niektórych innych pokarmach zwierzęcych. Możliwe efekty uboczne Większość ludzi może bezpiecznie spożywać shiitake, chociaż mogą wystąpić pewne skutki uboczne. W rzadkich przypadkach ludzie mogą rozwinąć wysypkę skórną z powodu jedzenia lub obchodzenia się z surowym shiitake (link ↗) .Uważa się, że ten stan, zwany zapaleniem skóry shiitake, jest spowodowany przez lentinan (link ↗) .Ponadto stosowanie sproszkowanego ekstraktu z grzybów przez długi czas może powodować inne skutki uboczne, w tym rozstrój żołądka i wrażliwość na światło słoneczne (link ↗) (link ↗) .Niektórzy twierdzą również, że wysoki poziom puryn w grzybach może powodować objawy u osób z dną moczanową. Niemniej jednak badania sugerują, że jedzenie grzybów wiąże się z niższym ryzykiem dny moczanowej (link ↗) BIBLIOGRAFIA 1. Ng ML, Yap AT. Hamowanie rozwoju ludzkiego raka okrężnicy przez lentinan z grzybów shiitake (Lentinus edodes). J Altern Uzupełnienie Med. 2002;8:581-9. 2. Okamoto T, Kodoi R, Nonaka Y i in. Lentinan z grzyba shiitake (Lentinus edodes) hamuje ekspresję podrodziny cytochromu P450 1A w wątrobie myszy. Biofaktory. 2004;21:407-9. 3. Ngai PH, Ng TB. Lentin, nowe i silne białko przeciwgrzybicze z grzyba shitake o działaniu hamującym aktywność odwrotnej transkryptazy ludzkiego wirusa niedoboru odporności-1 i proliferacji komórek białaczki. Życie Sci. 2003;73:3363-74. 4. Israilides C, Kletsas D, Arapoglou D, et al. Właściwości cytostatyczne i immunomodulujące in vitro grzyba leczniczego Lentinula edodes. Fitomedycyna. 2008;15:512-9. 5. Akamatsu S, Watanabe A, Tamesada M i in. Hepatoprotekcyjne działanie ekstraktów z grzybni Lentinus edodes na uszkodzenie wątroby wywołane dimetylonitrozoaminą. Biol Pharm Byk. 2004;27:1957-60. 6. de Lima PL, Delmanto RD, Sugui MM i in. Letinula edodes (Berk.) Pegler (Shiitake) moduluje działanie genotoksyczne i mutagenne wywoływane przez środki alkilujące in vivo. Mutat Res. 2001;496:23-32. 7. Shouji N, Takada K, Fukushima K, Hirasawa M. Przeciwpróchniczy efekt składnika shiitake (grzyb jadalny). Próchnica Res. 2000;34:94-8. 8. deVere White RW, Hackman RM, Soares SE, Beckett LA, Sun B. Wpływ ekstraktu z grzybni grzybów na leczenie raka prostaty. Urologia. 2002;60:640-4. 9. Isoda N, Eguchi Y, Nukaya H, et al. Skuteczność kliniczna superdrobno rozproszonego lentinanu (beta-1,3-glukanu) u pacjentów z rakiem wątrobowokomórkowym. Hepatogastroenterologia. 2009;56:437-41. 10. Oba K, Kobayashi M, Matsui T, Kodera Y, Sakamoto J. Indywidualna metaanaliza lentinanu na podstawie nieoperacyjnego / nawrotowego raka żołądka. Przeciwnowotworowe Res. 2009;29:2739-45. 11. Hazama S, Watanabe S, Ohashi M i in. Skuteczność podawanego doustnie superdrobno rozproszonego lentinanu (beta-1,3-glukanu) w leczeniu zaawansowanego raka jelita grubego. Przeciwnowotworowe Res. 2009;29:2611-7. 12. Shimizu K, Watanabe S, Watanabe S, et al. Skuteczność podawanego doustnie superdrobno rozproszonego lentinanu w zaawansowanym raku trzustki. Hepatogastroenterologia. 2009;56:240-4. 13. Suzuki K, Tanaka H, Sugawara H, et al. Przewlekłe zapalenie płuc z nadwrażliwości wywołane przez zarodniki grzyba Shiitake związane z rakiem płuc. Stażysta Med. 2001;40:1132-5. 14. Hanada K, Hashimoto I. Grzybica wiciowców (Shiitake) zapalenie skóry i nadwrażliwość na światło. Dermatologia. 1998;197:255-7. 15. Levy AM, Kita H, Phillips SF i in. Eozynofilia i objawy żołądkowo-jelitowe po spożyciu grzybów shiitake. J Allergy Clin Immunol. 1998;101:613-20. 16. Garg S, Cockayne SE. Zapalenie skóry Shiitake zdiagnozowane po 16 latach! Dermatol łukowy. 2008;144:1241-2. 17. Goikoetxea MJ, FernĂndez-BenĂtez M, Sanz ML. Alergia pokarmowa na Shiitake (Lentinus edodes) objawiała się objawami ze strony przełyku u pacjenta z prawdopodobnym eozynofilowym zapaleniem przełyku. Allergol Immunopatol (Madr). 2009;37:333-4. 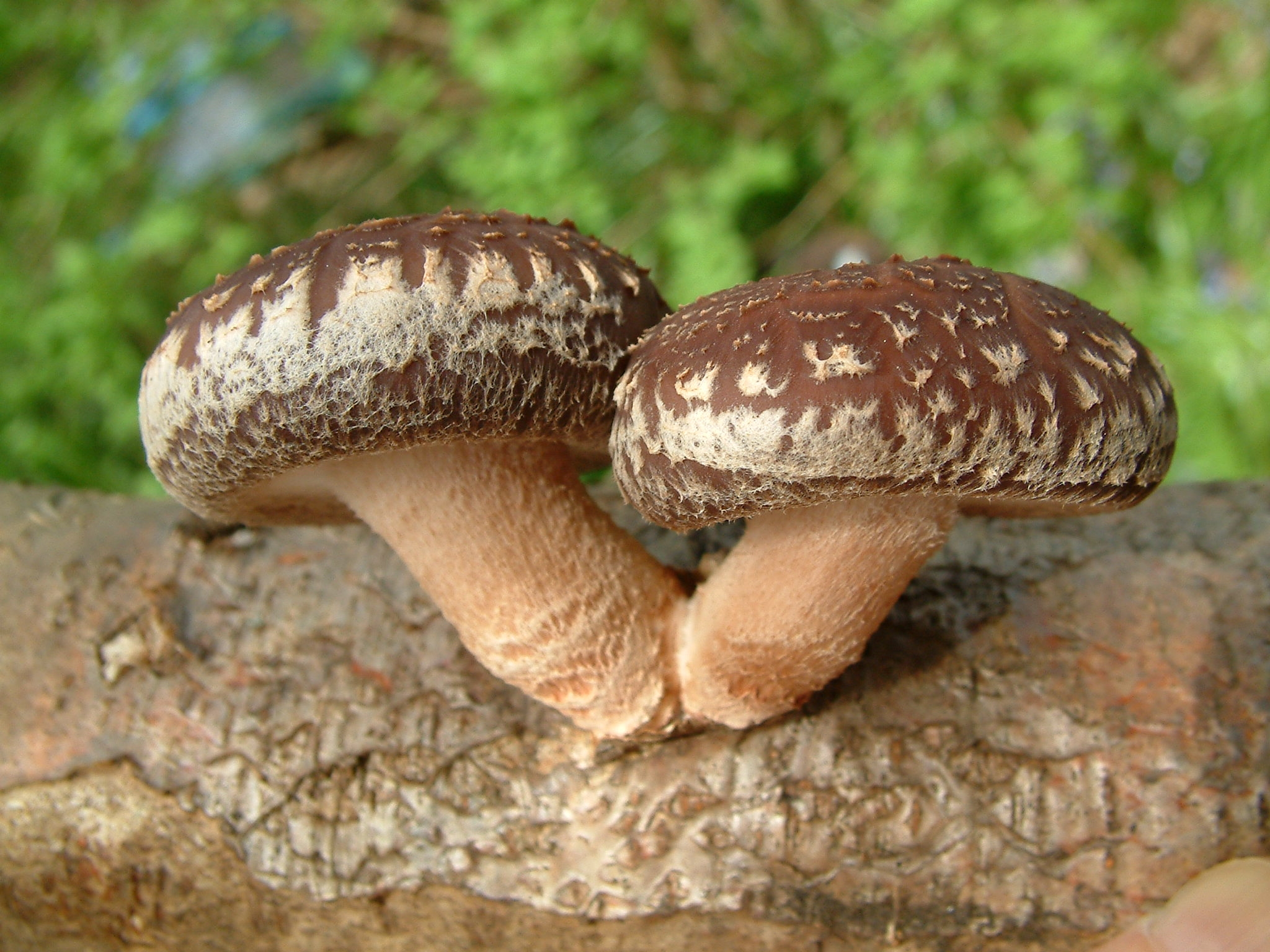 https://piewca.pl/shitake-twardnik-japonski-lentinula-edodes/ 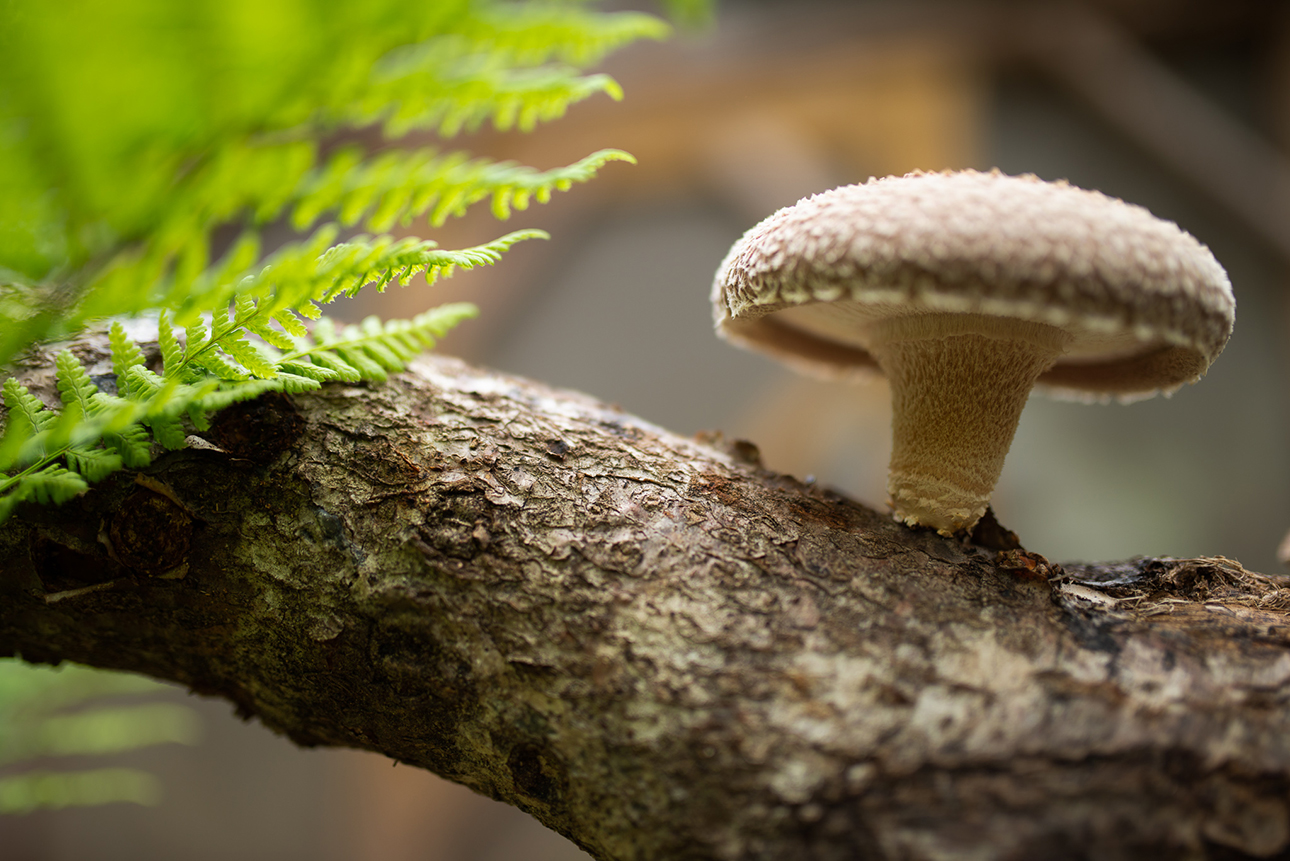
Hallo Leute. Wir haben uns mal wieder Tickets für ein schönes kleines Konzert gekauft. Die Band ### KETTCAR kommen mal nach Trier. Die Band spielt in Trier am Brunnenhof und das Konzert ist ein Open Air. Ich bin gespannt welche Band als Vorband bei Kettcar auftreten wird. Das wird bestimmt ein tolles Konzert. Habt alle einen schönen Tag. Gruß vom @bitandi 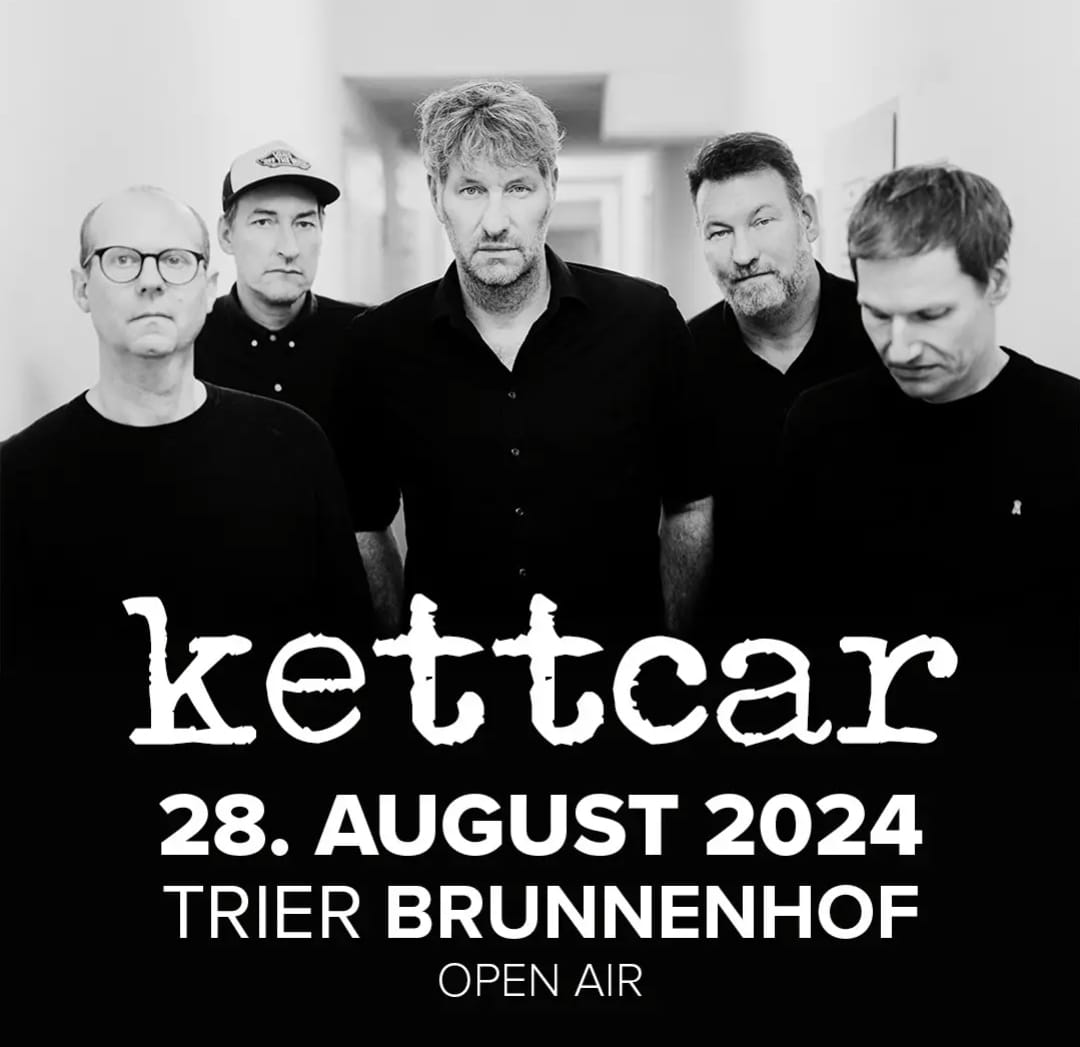
Every time I think about it, the world seems a little more insignificant than it already is. Putting all the physical and mathematical facts aside, in the end there are only two possibilities: Everything is determined, everything happens by chance. One side is depressing, you can't achieve anything anyway or you think you have control over what you do. The other side seems scary, also no control and you would have to rely on your fate if such a thing exists. Given these views, regardless of which of the two, does it even make sense to do anything?
<html> <p><img src="https://www.hindustantimes.com/ht-img/img/2023/10/06/550x309/CRICKET-ICC-MENS-WC-2023-ENGNZL-ODI-6_1696592836309_1696592836773.jpg"/></p> <p> USA vs Canada Live Score: Welcome to the live coverage of 1st T20I of Canada tour of USA, 2024. Match will start on 07 Apr 2024 at 08:30 PM<br/>Venue : Prairie View Cricket Complex, Prairie View, Texas<br/><br/>USA squad -<br/>Aaron Jones, Gajanand Singh, Nitish Kumar, Saiteja Mukkamalla, Steven Taylor, Corey Anderson, Harmeet Singh, Juanoy Drysdale, Milind Kumar, Nisarg Patel, Shadley van Schalkwyk, Andries Gous, Monank Patel, Shayan Jahangir, Jessy Singh, Nosthush Kenjige, Saurabh Netravalkar, Usman Rafiq<br/>Canada squad -<br/>Aaron Johnson, Ajayveer Hundal, Navneet Dhaliwal, Nicholas Kirton, Udaybir Walia, Dillon Heyliger, Dilpreet Bajwa, Harsh Thaker, Saad Bin Zafar, Sahib Malhotra, Shahid Ahmadzai, Shreyas Movva, Srimantha Wijeyeratne, Ishwarjot Sohi, Jeremy Gordon, Nikhil Dutta, Pargat Singh, Uday Bhagwan, Yuvraj Samra</p> <p><br/> <br/> <br/></p> </html>
1️⃣不在阿那亚园区住的话的话,可以在App上预约孤独图书馆或电影院观影进入园区,需要提前1天预约,实名预约,凭码入园,每天9点半以后开始。但是不住园区不能乘坐免非巴士,全 程靠腿💃还有不能在食堂消费,部分商铺是可以的。想看日出,建议住园区比较方便,淡季anlan499 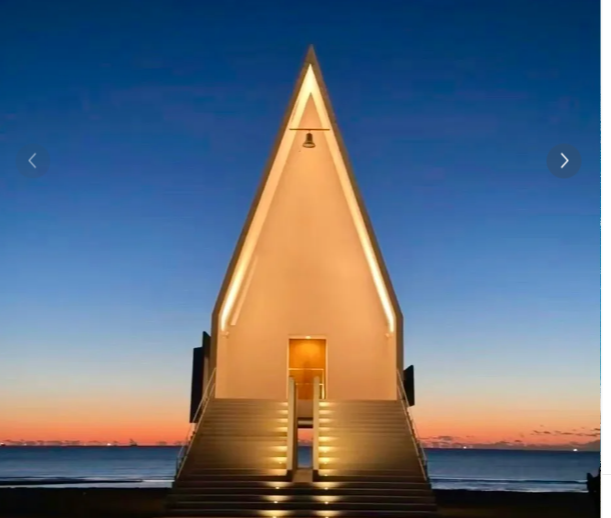 2️⃣这个季节大衣就行,但高低带个羽绒服或马甲类的,早晚温差大,海边风也大,戴帽子和围巾保暖拍照还有氛围,租车直接软件租就行,带好驾驶证身 份证。 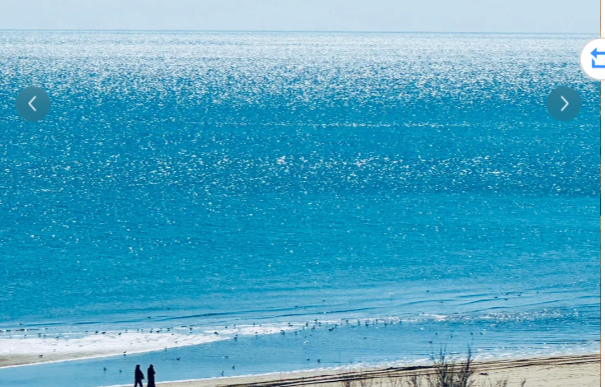 3️⃣阿那亚,有两个火车站,昌黎、北戴河,离阿那亚蕞方便的是北戴河站,出站后可以滴网约车,直接到酒店门口,票价35,另外阿那亚高铁站也有园区班车,票价10元,车程25分钟。 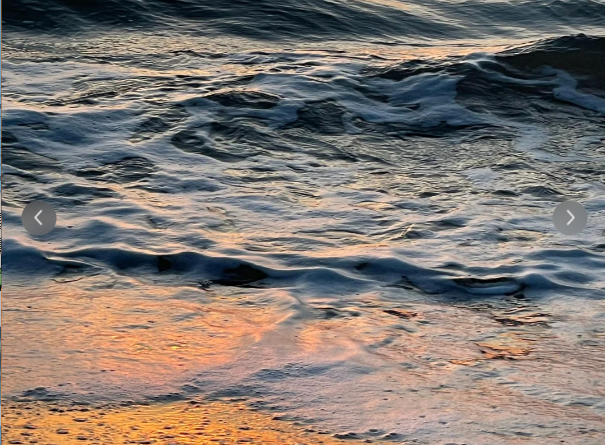 4️⃣四食堂的酒酿皮子、煎饼果子好吃到起飞,鸡汤馄饨、小麦穗的蒸饺,黄河入海的酸辣毛肚、烤菜饼、杏皮水,还有酿皮子,天花板了家人们,颠境昆明小锅米线,黑豆花,米酒,三件套要点,yyds第八食堂,常季海鲜杏仁茄子,颠覆了我对茄子的认知,很新奇的一道菜,跟想象中的口味不一样;还有赞爆的海盐冰淇淋好吃到哭。 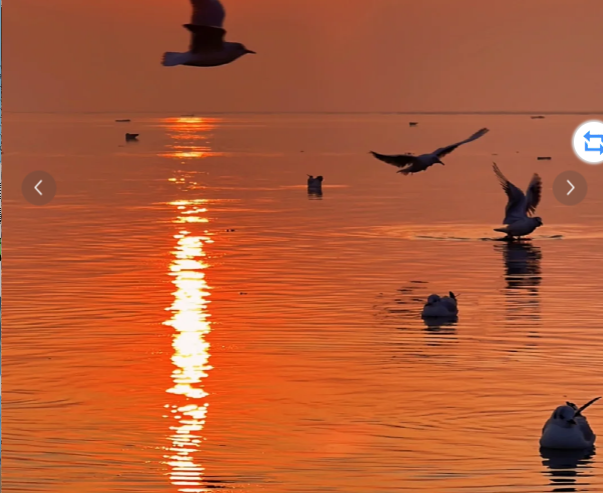 5️⃣找民宿,房源信息,光看屋里有啥没用,得往下拉,看看房东写的信息里是否有允许做饭,因为有的房东是不允许做饭的。洗澡水好像2.4期都是电热水器,6期好像燃气的? 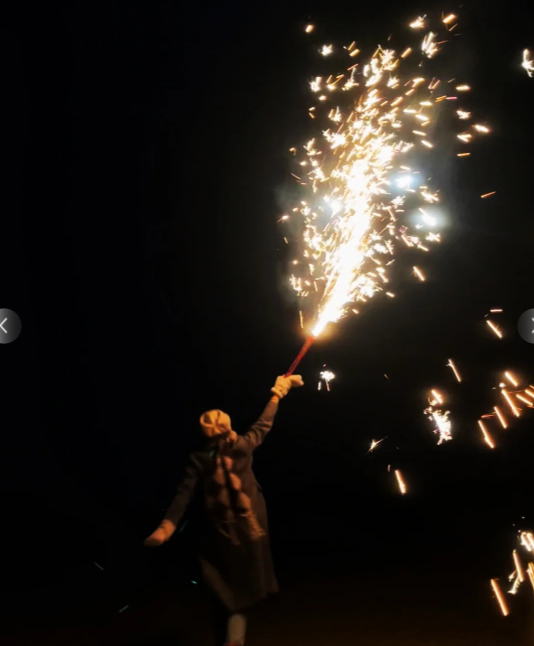 6️⃣高圆圆光顾的宿咕面包店真的好好吃,临走前打包三袋的程度,蛋挞,奶挞,苹果派,羊角,还有个长棍子也好好吃!除了贵没毛并 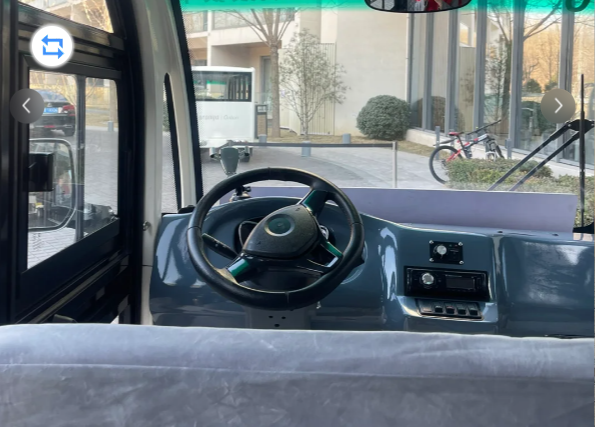 7️⃣安澜的接驳车贼慢,不过也没有别的选择 8️⃣我去了5次阿那亚,两次冬天三次夏天,对这里的整体感受还是很 好的,主打一个海边社区,随便放放空,两天一夜撑死三天两夜的小旅行,我看有人说,沿海城市的雹子不建议来,我倒觉得跟南方的海边体验感可不一样,尤 ,其是冬天,赶上天气给力,冬天飘雪看着教堂和结冰的海面真是太浪漫了 9️⃣如果住鸽子窝记得带水和吃的,晚上根本找不到一个买东西的地方(但住鸽子窝一晚很tui荐,因为看日落日出都钞方便,备好吃的啥都 不干躺床上看海就很享 受!如果能找到烟花可以放烟花,前两次去没找到,结果过去晚上有人在楼下放羡慕死了
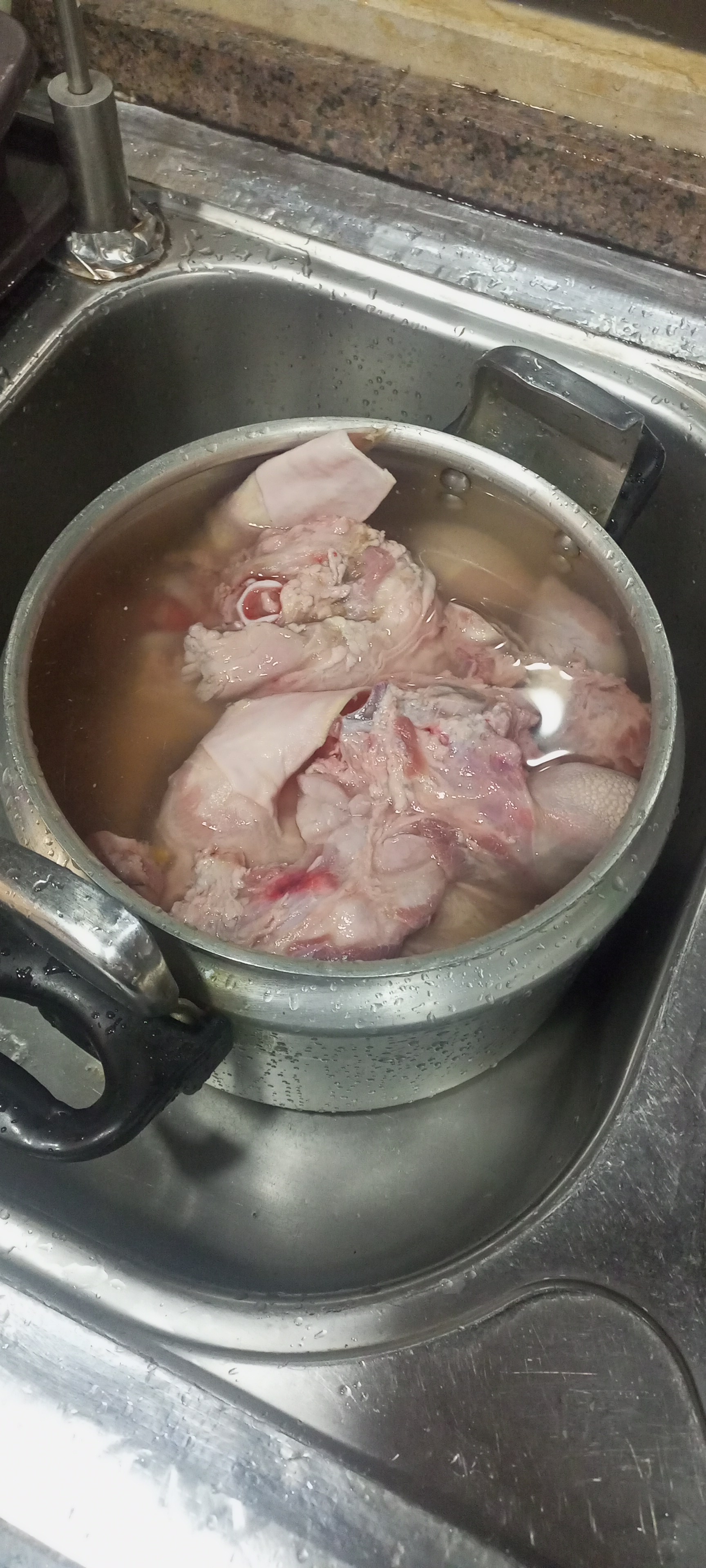