## 题目描述 There are two sorted arrays nums1 and nums2 of size m and n respectively. Find the median of the two sorted arrays. The overall run time complexity should be O(log (m+n)). You may assume nums1 and nums2 cannot be both empty. Example 1: nums1 = [1, 3] nums2 = [2] The median is 2.0 Example 2: nums1 = [1, 2] nums2 = [3, 4] The median is (2 + 3)/2 = 2.5 ## 分析 算中位数,在我们平时的思路上, 就是把一组无序的数, 排序, 取位置在最中间的数,如果数组有偶数个数, 就取中间两个数的平均数 因此最容易想到的方法是, 把数组 nums1 和数组 nums2 合并起来, 然后使用sort()方法排序, 取中间数即可。 ```javascript /** * @param {number[]} nums1 * @param {number[]} nums2 * @return {number} */ var findMedianSortedArrays = function(nums1, nums2) { // 合并 let nums = nums1.concat(nums2) // 排序 nums.sort(function(a, b) { return a-b }) let l = nums.length // 偶数个 返回中间两数平均值 if (l % 2 === 0) { return (nums[l / 2 - 1] + nums[l / 2]) / 2 } else { // 奇数个 返回中间的数 return nums[parseInt(l / 2)] } }; ``` 但这样解其实是不符合题意的, 因为题目限定了时间复杂度为O(log(m + n)), 这是一个log级别的复杂度, 显然是不能使用任何排序算法的,这也是这个题目难度是困难的原因 这道题我是不会的, 因为我没有想到中位数另外一个定义: 将一个集合划分为两个长度相等的子集,其中一个子集中的元素总是大于另一个子集中的元素。 也就是说, 这是一种分而治之的思想, 如果num1长度为3, nums2长度为4, 我们可以从中间对nums1数组切一刀, 假设切一刀后, nums1左侧的元素个数为 1 个, 那么根据中位数定义中“将一个集合划分为两个长度相等的子集”,可以知道 ,此时num2左侧的元素个数一定要为 6, 因为只有这样切,才可以保证两边元素个数相等。 left | right nums1[0], nums1[1], ..., nums1[i-1] | nums1[i], nums1[i+1], ..., nums1[m-1] nums2[0], nums2[1], ..., nums2[j-1] | nums2[j], nums2[j+1], ..., nums2[n-1] 假设 L1 为 nums1 切面左侧的最后一个元素 num1[i - 1], R2为 nums2切面右侧第一个元素nums[j], 那么根据定义“其中一个子集中的元素总是大于另一个子集中的元素”可知 L1 一定要 小于 R2, 同理 L2一定要小于 R1, 如果不满足, 就要用到二分查找, 更新切面的位置,直到满足这两个定义: - 两个长度相等的子集 即 分别切开后, nums1和nums2左侧元素个数和右侧元素个数要相等 - 其中一个子集中的元素总是大于另一个子集中的元素。即 L1 < R2, L2 < R1 寻找到满足条件的切点后, 计算中位数,如果是奇数个, 取nums1, nums2左侧较大的数, 右侧较小的数,取平均值 如果是偶数个, 取右侧较小值即为所求 ## Javascript解法 ```javascript /** * @param {number[]} nums1 * @param {number[]} nums2 * @return {number} */ var findMedianSortedArrays = function(nums1, nums2) { // 过滤边界情况 let l1 = nums1.length, l2 = nums2.length if(l1 === 0 && l2 === 1) { return nums2[0] } if (l2 === 0 && l1 === 1) { return nums1[0] } if (l1 === 1 && l2 === 1) { return (nums1[0] + nums2[0]) / 2 } // 如果第一个数组长度大于第二个, 则把他们顺序颠倒 运行函数 // 因为知道了nums1在哪儿切, 就知道了nums2在哪儿切, 因此选数组长度小的切节省时间 if(nums1.length > nums2.length) { return findMedianSortedArrays(nums2, nums1) } // 二分查找切点位置的左右边界 let cutL = 0 let cutR = nums1.length let cut1 = 0, cut2 = 0 // 二分查找 while(cutL <= cutR) { // 根据中位数的特征 两个数组合起来的中位数, 在数组的中间位置切开 // 如果nums1 + nums2的总长度是5, 假设从nums1第二个位置切开, // nums1被切开的左侧有两个元素, 那么直接就可知道, 此时nums2在第三 // 个位置被切开 cut1 = parseInt((cutR - cutL) / 2) + cutL cut2 = parseInt((l1 + l2) / 2) - cut1 // 分别计算切面两侧的元素L1, L2, R1, R2 let L1 = (cut1 === 0) ? -Infinity : nums1[cut1 - 1] let L2 = (cut2 === 0) ? -Infinity : nums2[cut2 - 1] let R1 = (cut1 === l1) ? Infinity : nums1[cut1] let R2 = (cut2 === l2) ? Infinity : nums2[cut2] // 如果左侧大于了右侧, 说明该切点切得太靠后, 减少右边界 if (L1 > R2) { cutR = cut1 - 1 } else if (L2 > R1) { // 如果右侧大于左侧, 说明切点太靠前, 增加左边界 cutL = cut1 + 1 } else { // 当满足情况时, 取L1 L2中的最大的, R1,R2中最小的,即为合成后的数组的中间两数 如果数量为偶数个 返回均值 if ((l1 + l2) % 2 === 0) { L1 = L1 > L2 ? L1 : L2 R1 = R1 < R2 ? R1 : R2 return (L1 + R1) / 2 } else { // 如果是奇数个, 按照规律 直接返回R1 R2中的较大者即为所求 R1 = R1 < R2 ? R1 : R2 return R1 } } } return -1 }; ``` ## 运行结果 
post_id | 76,306,923 | ||||||
---|---|---|---|---|---|---|---|
author | hughie8 | ||||||
permlink | leetcode-4-or-median-of-two-sorted-arrays | ||||||
category | esteem | ||||||
json_metadata | {"image":["https:\/\/img.esteem.ws\/x1dpf7iozr.png"],"users":["param","param","return","param","param","return"],"tags":["esteem","cn","programming","cnstm","partiko"],"app":"esteem\/2.1.0-surfer","format":"markdown+html","community":"esteem.app"} | ||||||
created | 2019-06-13 03:55:00 | ||||||
last_update | 2019-06-13 03:55:00 | ||||||
depth | 0 | ||||||
children | 13 | ||||||
net_rshares | 850,988,566,878 | ||||||
last_payout | 2019-06-20 03:55:00 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 0.344 SBD | ||||||
curator_payout_value | 0.114 SBD | ||||||
pending_payout_value | 0.000 SBD | ||||||
promoted | 0.000 SBD | ||||||
body_length | 3,753 | ||||||
author_reputation | 107,977,516,232 | ||||||
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 SBD | ||||||
percent_steem_dollars | 10,000 | ||||||
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
good-karma | 0 | 14,513,832,470 | 1.11% | ||
corina | 0 | 4,375,901,807 | 100% | ||
mysteem | 0 | 0 | 1.11% | ||
svetlanaaa | 0 | 4,246,745,745 | 100% | ||
demo | 0 | 6,600,548 | 1.11% | ||
tumutanzi | 0 | 323,126,616 | 0.05% | ||
feruz | 0 | 68,392,654 | 1.11% | ||
carterx7 | 0 | 4,118,940,198 | 100% | ||
rishi556 | 0 | 32,333,337,096 | 100% | ||
esteemapp | 0 | 229,248,933,904 | 1.11% | ||
shafay | 0 | 5,917,934,955 | 100% | ||
frsvp | 0 | 3,991,818,100 | 100% | ||
gamemusic | 0 | 6,418,039,944 | 100% | ||
tilenpirih | 0 | 7,291,817,789 | 100% | ||
kavaeron | 0 | 6,543,213,268 | 100% | ||
mrwhitenight | 0 | 4,246,098,477 | 100% | ||
djbk | 0 | 7,487,999,739 | 100% | ||
tatyanamishenko | 0 | 6,542,092,006 | 100% | ||
dpakyaw | 0 | 6,491,524,246 | 100% | ||
afeliseth | 0 | 405,137,890 | 100% | ||
drotto | 0 | 12,472,331,054 | 3.13% | ||
lovejuice | 0 | 174,988,425,272 | 27.25% | ||
touristpk | 0 | 8,621,090,551 | 100% | ||
dipom98 | 0 | 2,537,685,960 | 100% | ||
dream.trip | 0 | 3,768,907,139 | 100% | ||
rudee | 0 | 3,855,781,283 | 100% | ||
kimzwarch | 0 | 5,294,864,866 | 4% | ||
davidke20 | 0 | 8,579,585,925 | 7.5% | ||
sostrin | 0 | 8,431,582,203 | 100% | ||
minloulou | 0 | 758,868,159 | 5% | ||
hannesl | 0 | 6,562,152,708 | 100% | ||
folker-wulff | 0 | 8,176,196,283 | 100% | ||
cryptocurrencyhk | 0 | 345,392,890 | 20% | ||
esteem.app | 0 | 196,186,711 | 1.11% | ||
jaforce | 0 | 8,345,841,891 | 100% | ||
lrd | 0 | 2,465,806,802 | 100% | ||
paradaise | 0 | 5,164,502,081 | 100% | ||
cnbuddy | 0 | 1,126,945,442 | 0.1% | ||
steemnews-fr | 0 | 5,323,589,539 | 100% | ||
r351574nc3 | 0 | 9,250,191,533 | 100% | ||
safedeposit | 0 | 3,947,171,338 | 100% | ||
rahulsingh25843 | 0 | 7,026,602,254 | 100% | ||
jason7282 | 0 | 5,984,448,047 | 100% | ||
allenaxie | 0 | 5,656,056,956 | 100% | ||
sljivanono | 0 | 1,709,802,722 | 100% | ||
sizandyola | 0 | 5,139,198,929 | 100% | ||
artoftherhyme | 0 | 5,822,700,511 | 100% | ||
joe420 | 0 | 4,531,074,398 | 100% | ||
starlord28 | 0 | 474,961,091 | 100% | ||
hugojimenez | 0 | 6,003,850,976 | 100% | ||
jonnyla08 | 0 | 1,284,303,153 | 100% | ||
coinzzz | 0 | 5,705,838,523 | 100% | ||
weimunyap | 0 | 22,981,197,530 | 15.48% | ||
terrywayne | 0 | 8,630,917,748 | 100% | ||
chuuuckie | 0 | 1,718,917,667 | 1.75% | ||
mysupport | 0 | 7,547,716,460 | 100% | ||
oclinton | 0 | 286,925,887 | 12.5% | ||
honestbot | 0 | 36,065,106,450 | 14.39% | ||
zapzap | 0 | 3,144,999,203 | 100% | ||
kirstin | 0 | 9,802,720,006 | 100% | ||
goldbloger-6 | 0 | 513,895,835 | 100% | ||
votefun | 0 | 847,833,279 | 25% | ||
aimal32 | 0 | 516,045,149 | 100% | ||
votepower | 0 | 4,800,426,486 | 100% | ||
dugan | 0 | 7,891,923,508 | 100% | ||
shunnedscarab55 | 0 | 627,562,407 | 75% | ||
curationkiwi | 0 | 8,627,703,320 | 25% | ||
thedoubleace123 | 0 | 235,974,258 | 100% | ||
cmono | 0 | 4,226,211,442 | 100% | ||
fron | 0 | 550,107,929 | 100% | ||
happy33 | 0 | 20,455,941,897 | 100% | ||
bugger | 0 | 5,568,112,928 | 100% | ||
teamcn-shop | 0 | 9,932,321,526 | 10% | ||
wokeprincess | 0 | 1,306,494,416 | 100% | ||
marwamohamed | 0 | 96,286,528 | 25% | ||
astrochologist | 0 | 2,277,248,709 | 100% | ||
steemexplorers | 0 | 3,185,149,519 | 100% | ||
monstermadness | 0 | 1,299,595,909 | 100% | ||
kingtherion | 0 | 4,428,458,827 | 100% | ||
tombramycin | 0 | 2,139,629,392 | 100% | ||
just4kicks46 | 0 | 1,452,296,658 | 100% | ||
hozn4ukhlytriwc | 0 | 146,199,643 | 15% | ||
thecryptohoarder | 0 | 4,505,662,576 | 100% | ||
hughie8 | 0 | 1,055,559,144 | 100% |
<p>This post has received a 3.13 % upvote from @drotto thanks to: @hughie8.</p>
post_id | 76,307,572 |
---|---|
author | drotto |
permlink | re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t041342961z |
category | esteem |
json_metadata | {"tags":["esteem"],"app":"drotto\/0.0.5pre2"} |
created | 2019-06-13 04:13:42 |
last_update | 2019-06-13 04:13:42 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 04:13:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 80 |
author_reputation | 424,402,347,817 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
This post has received a 27.25% upvote from @lovejuice thanks to @hughie8. They love you, so does Aggroed. Please be sure to vote for Witnesses at https://steemit.com/~witnesses.
post_id | 76,308,161 |
---|---|
author | lovejuice |
permlink | re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t043205757z |
category | esteem |
json_metadata | {"app":"postpromoter\/1.7.4"} |
created | 2019-06-13 04:32:06 |
last_update | 2019-06-13 04:32:06 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 04:32:06 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 178 |
author_reputation | 10,525,002,852,777 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
You just received a 14.39% upvote from @honestbot, courtesy of @hughie8! 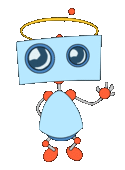
post_id | 76,308,474 |
---|---|
author | honestbot |
permlink | re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t043949600z |
category | esteem |
json_metadata | {"app":"postpromoter\/1.8.6"} |
created | 2019-06-13 04:39:48 |
last_update | 2019-06-13 04:39:48 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 04:39:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 179 |
author_reputation | -221,026,549,797 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
赞如果是买的就不好了。#esteem 有抽成10% Posted using [Partiko Android](https://partiko.app/referral/davidke20)
post_id | 76,309,865 |
---|---|
author | davidke20 |
permlink | davidke20-re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t052653866z |
category | esteem |
json_metadata | {"app":"partiko","client":"android"} |
created | 2019-06-13 05:26:54 |
last_update | 2019-06-13 05:26:54 |
depth | 1 |
children | 1 |
net_rshares | 0 |
last_payout | 2019-06-20 05:26:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 97 |
author_reputation | 317,850,004,724,810 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
(⊙o⊙)哦 我不知道哦,, 多谢告知、 我也是刚装了eSteem试试, 感觉界面好看 小买 都是minimum payment, 花的少, 主要为了reputation提的快
post_id | 76,310,205 | ||||||
---|---|---|---|---|---|---|---|
author | hughie8 | ||||||
permlink | re-davidke20-2019613t133719660z | ||||||
category | esteem | ||||||
json_metadata | {"tags":["esteem"],"app":"esteem\/2.1.0-surfer","format":"markdown+html","community":"esteem.app"} | ||||||
created | 2019-06-13 05:37:42 | ||||||
last_update | 2019-06-13 05:37:42 | ||||||
depth | 2 | ||||||
children | 0 | ||||||
net_rshares | 0 | ||||||
last_payout | 2019-06-20 05:37:42 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 0.000 SBD | ||||||
curator_payout_value | 0.000 SBD | ||||||
pending_payout_value | 0.000 SBD | ||||||
promoted | 0.000 SBD | ||||||
body_length | 88 | ||||||
author_reputation | 107,977,516,232 | ||||||
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 SBD | ||||||
percent_steem_dollars | 10,000 |
Thanks for using **eSteem**! <br>Your post has been voted as a part of [eSteem encouragement program](https://steemit.com/esteem/@good-karma/encouragement-program-continues-82eafcd10a299). Keep up the good work! Install [Android](https://play.google.com/store/apps/details?id=app.esteem.mobile), [iOS](https://itunes.apple.com/WebObjects/MZStore.woa/wa/viewSoftware?id=1451896376&mt=8) Mobile app or [Windows, Mac, Linux](https://github.com/esteemapp/esteem-surfer/releases) Surfer app, if you haven't already!<br>Learn more: https://esteem.app <br>Join our discord: https://discord.gg/8eHupPq
post_id | 76,309,928 |
---|---|
author | esteemapp |
permlink | re-2019613t72850990z |
category | esteem |
json_metadata | {"tags":["esteem"],"app":"esteem\/2.0-welcome","format":"markdown+html","community":"esteem.app"} |
created | 2019-06-13 05:28:51 |
last_update | 2019-06-13 05:28:51 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 05:28:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 593 |
author_reputation | 396,075,316,369,469 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
你那里天气如何?来一份新手村小卖部的美食吧!@teamcn-shop倘若你想让我隐形,请回复“取消”。
post_id | 76,312,832 |
---|---|
author | cnbuddy |
permlink | re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t065610036z |
category | esteem |
json_metadata | {} |
created | 2019-06-13 06:56:09 |
last_update | 2019-06-13 06:56:09 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 06:56:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 51 |
author_reputation | -1,405,328,253,928 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
你好鸭,hughie8! @cnbuddy给您叫了一份外卖! 由 @sasaadrian 一灯大师 迎着沙尘暴 跑着步 念着软哥金句:"别以为你长的稀有样我们就应该物以稀为贵。" 给您送来 **大闸蟹** <br>  吃饱了吗?跟我猜拳吧! **石头,剪刀,布~** 如果您对我的服务满意,请不要吝啬您的点赞~ @onepagex
post_id | 76,312,836 |
---|---|
author | teamcn-shop |
permlink | re-hughie8-leetcode-4-or-median-of-two-sorted-arrays-20190613t065610036z |
category | esteem |
json_metadata | "{"app":"teamcn-shop bot\/1.0"}" |
created | 2019-06-13 06:56:15 |
last_update | 2019-06-13 06:56:15 |
depth | 1 |
children | 2 |
net_rshares | 0 |
last_payout | 2019-06-20 06:56:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 247 |
author_reputation | 66,748,950,931,416 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
石头
post_id | 76,419,686 |
---|---|
author | hughie8 |
permlink | pt4gue |
category | esteem |
json_metadata | {"tags":["esteem"],"app":"steemit\/0.1"} |
created | 2019-06-15 04:08:42 |
last_update | 2019-06-15 04:08:42 |
depth | 2 |
children | 1 |
net_rshares | 0 |
last_payout | 2019-06-22 04:08:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 2 |
author_reputation | 107,977,516,232 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
https://4.bp.blogspot.com/-mmW-s8jFGGo/WoGwFCWm5jI/AAAAAAATXI4/T9UiUyrg7acAFnpPigWlgbdyo-Cb4M8AgCLcBGAs/s1600/AW785125_15.gif You win!!!! 你赢了! 给你1枚SHOP币!
post_id | 76,419,687 |
---|---|
author | teamcn-shop |
permlink | pt4gue |
category | esteem |
json_metadata | "{"app":"teamcn-shop bot\/1.0"}" |
created | 2019-06-15 04:08:48 |
last_update | 2019-06-15 04:08:48 |
depth | 3 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-22 04:08:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 155 |
author_reputation | 66,748,950,931,416 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Congratulations @hughie8! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) : <table><tr><td><img src="https://steemitimages.com/60x70/http://steemitboard.com/@hughie8/voted.png?201906130534"></td><td>You received more than 500 upvotes. Your next target is to reach 1000 upvotes.</td></tr> </table> <sub>_You can view [your badges on your Steem Board](https://steemitboard.com/@hughie8) and compare to others on the [Steem Ranking](https://steemitboard.com/ranking/index.php?name=hughie8)_</sub> <sub>_If you no longer want to receive notifications, reply to this comment with the word_ `STOP`</sub> ###### [Vote for @Steemitboard as a witness](https://v2.steemconnect.com/sign/account-witness-vote?witness=steemitboard&approve=1) to get one more award and increased upvotes!
post_id | 76,319,542 |
---|---|
author | steemitboard |
permlink | steemitboard-notify-hughie8-20190613t095553000z |
category | esteem |
json_metadata | {"image":["https:\/\/steemitboard.com\/img\/notify.png"]} |
created | 2019-06-13 09:55:51 |
last_update | 2019-06-13 09:55:51 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 09:55:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 840 |
author_reputation | 38,705,954,145,809 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
You got voted by @curationkiwi thanks to hughie8! This bot is managed by [KiwiJuce3](https://bit.ly/2HQOvIO) and run by [Rishi556](https://bit.ly/2OmBsQF), you can check both of them out there. To receive upvotes on your own posts, you need to join the [Kiwi Co. Discord](https://bit.ly/2U8Mo9M) and go to the room named #CurationKiwi. Submit your post there using the command "!upvote (post link)" to receive upvotes on your post. CurationKiwi is currently supported by donations from users like you, so feel free to leave an upvote on our posts or comments to support us! We have also recently added a new whitelist feature for those who would like to support CurationKiwi even more! If you would like to receive upvotes more than 2x greater than the normal upvote, all you need to do is delegate 50 SP to @CurationKiwi using [this link](https://app.steemconnect.com/sign/delegateVestingShares?account=curationkiwi&delegatee=curationkiwi&vesting_shares=99572.000000%20VESTS).
post_id | 76,333,342 |
---|---|
author | curationkiwi |
permlink | re-leetcode-4-or-median-of-two-sorted-arrays |
category | esteem |
json_metadata | {"app":"Discord"} |
created | 2019-06-13 16:28:15 |
last_update | 2019-06-13 16:28:15 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-06-20 16:28:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 978 |
author_reputation | 2,747,191,214,125 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
You have been upvoted by all four members of the @steemexplorers team. Steemexplorers is an initiative to help bring all Steemians information on various services and communities operating all on the STEEM blockchain in a centralized location to save you time and help you grow here on Steemit. It's free, it's easy, and there's a whole lot of information here that you can put to good use. Please come by our discord channel to learn more and feel free to ask as many questions as you'd like. We're here to help! Link to Discord: https://discord.gg/6QrMCFq
post_id | 76,333,353 |
---|---|
author | steemexplorers |
permlink | re-leetcode-4-or-median-of-two-sorted-arrays |
category | esteem |
json_metadata | {"app":"Discord"} |
created | 2019-06-13 16:28:36 |
last_update | 2019-06-13 16:28:36 |
depth | 1 |
children | 0 |
net_rshares | 71,269,420,874 |
last_payout | 2019-06-20 16:28:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.032 SBD |
curator_payout_value | 0.007 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 557 |
author_reputation | 65,900,527,192,856 |
root_title | "LeetCode 4 | Median of Two Sorted Arrays 寻找两个有序数组的中位数" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
givememonsters | 0 | 46,526,607,674 | 100% | ||
dusthero | 0 | 24,742,813,200 | 100% |