在我的一个Python小脚本中,我有一个变态的需求,在一个函数中调用不同的函数,这个时候最简单的方法就是将待调用的函数作为参数传入。 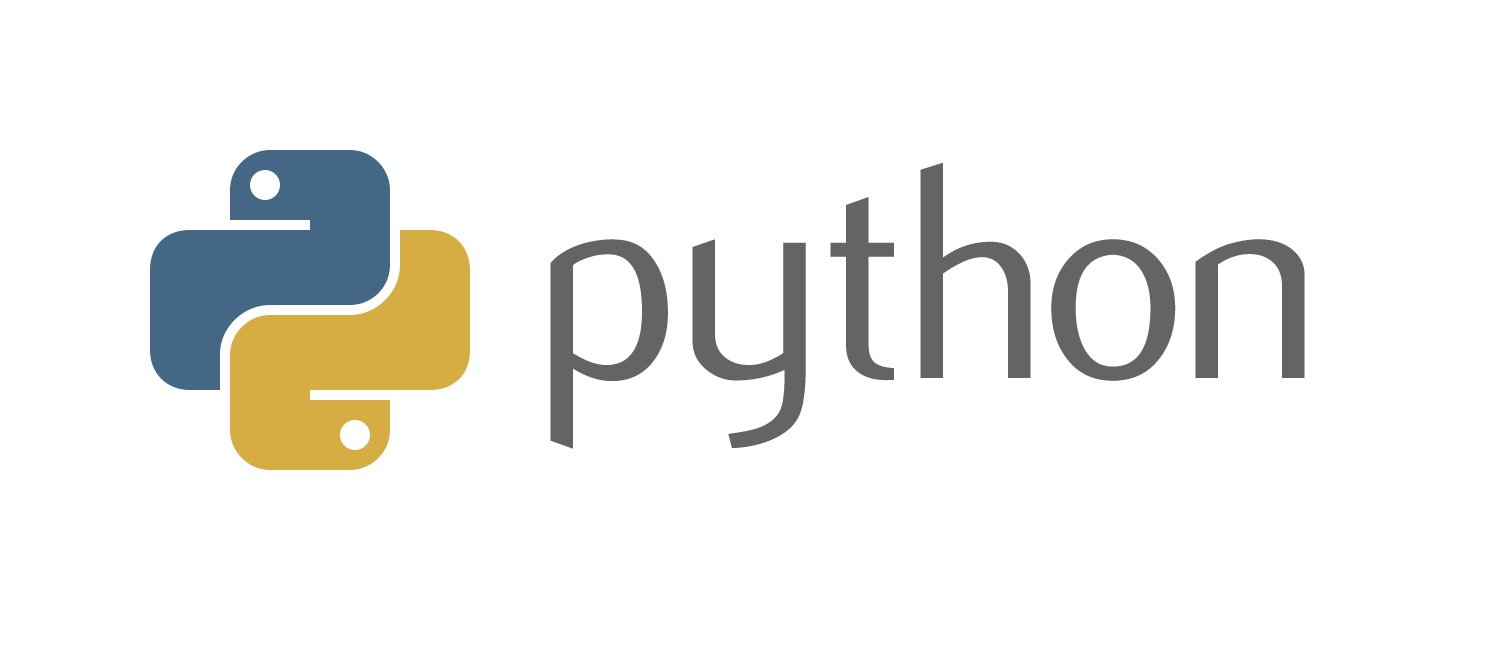 (图源:[bing.com](bing.com)) # 函数也是对象 之所以可以这样操作的原因是因为Python中一切皆是对象,举例说,我们可以定义个简单的函数并打印相关信息: ``` def add(x, y): return x + y print(add) print(type(add)) print(id(add)) ``` 上述代码将输出类似如下信息: ><function add at 0xb6b8d618> <class 'function'> 3065566744 # 函数作为参数 有了上述分析,我们就可以大胆的将函数作为参数传入其它函数中了,下边我们实现了简单的加法(add)、减法函数(sub)。同时我们定义了一个函数计算(calc),这个函数接受一个函数以及其它两个参数。 ``` def add(x, y): return x + y def sub(x, y): return x - y def calc(func, a, b): return func(a, b) print(calc(add, 1, 2)) print(calc(sub, 1, 2)) ``` 可见,我们可以通过调用calc函数,并指定用于进行计算的函数(add或者sub)来计算对应的数值。上述代码输入结果如下: >3 -1 # 函数参数的可变参数 上述例子中,我们直接在calc函数定义中指定了参数,并传递给其中的函数调用(add或sub),但是如果我们没法确定其内被调用的函数有多少个参数,或者有些参数是关键字参数,可怎么办呢? 答案是很简单的,我们只需将其传入到calc函数即可。 ``` def add(x, y): return x + y def add_more(x, y, z): return x + y + z def sub(x, y): return x - y def calc(func, *args, **kwargs): return func(*args, **kwargs) print(calc(add, 1, 2)) print(calc(sub, 1, 2)) print(calc(add_more, 1, 2, 3)) print(calc(add_more, x=1, y=2, z=3)) ``` 其中: ``` def calc(func, *args, **kwargs): return func(*args, **kwargs) ``` 第一句代表将位置参数打包给args(tuple类型),将关键字参数打包给kwargs(dict)类型。第二句函数调用括号内则代表将对应的数据解包。 将上述代码改进一下: ``` def add(x, y): return x + y def add_more(x, y, z): return x + y + z def sub(x, y): return x - y def calc(func, *args, **kwargs): print(args) print(kwargs) return func(*args, **kwargs) print(calc(add, 1, 2)) print() print(calc(sub, 1, 2)) print() print(calc(add_more, 1, 2, 3)) print() print(calc(add_more, x=1, y=2, z=3)) ``` 结果如下: >(1, 2) {} 3 > >(1, 2) {} -1 > >(1, 2, 3) {} 6 > >() {'x': 1, 'y': 2, 'z': 3} 6 # Python 内置函数 在Python内置函数中,有几个函数使用了函数作为参数,比如: * map() `map(function, iterable, ...)` * filter() `filter(function, iterable)` * reduce() `reduce(function, iterable[, initializer])` 本文就不做深入介绍了。
post_id | 44,448,297 |
---|---|
author | oflyhigh |
permlink | 4ckfhr-python |
category | python |
json_metadata | "{"links": ["bing.com"], "format": "markdown", "app": "steemit/0.1", "image": ["https://steemitimages.com/DQmXQzDMbiUMFVUWX8A9qodarzSPfjvgJH9NufV46enHncb/image.png"], "tags": ["python", "programming", "cn-programming", "learning", "cn"]}" |
created | 2018-04-20 02:45:09 |
last_update | 2018-04-20 02:45:09 |
depth | 0 |
children | 8 |
net_rshares | 19,817,018,110,948 |
last_payout | 2018-04-27 02:45:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 94.208 SBD |
curator_payout_value | 15.867 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 2,053 |
author_reputation | 1,148,153,621,496,884 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
pharesim | 0 | 81,708,774,945 | 0.2% | ||
abit | 0 | 152,024,075,024 | 100% | ||
adm | 0 | 8,069,705,669,542 | 50% | ||
blockchainbilly | 0 | 19,574,710,993 | 50% | ||
deanliu | 0 | 1,101,347,671,045 | 100% | ||
ace108 | 0 | 261,749,688,924 | 25% | ||
laoyao | 0 | 35,190,252,528 | 100% | ||
somebody | 0 | 1,258,750,546,322 | 100% | ||
midnightoil | 0 | 111,627,230,611 | 100% | ||
xiaohui | 0 | 696,758,323,045 | 100% | ||
oflyhigh | 0 | 2,206,718,132,317 | 100% | ||
xiaokongcom | 0 | 10,299,776,017 | 100% | ||
yulan | 0 | 14,854,713,580 | 100% | ||
chinadaily | 0 | 222,232,685,535 | 100% | ||
helene | 0 | 648,459,068,301 | 100% | ||
ffcrossculture | 0 | 34,846,325,844 | 100% | ||
ethansteem | 0 | 198,640,659,150 | 100% | ||
englishtchrivy | 0 | 56,749,560,520 | 26% | ||
profitgenerator | 0 | 397,272,965 | 100% | ||
damarth | 0 | 80,398,937,432 | 3% | ||
jianghao | 0 | 231,676,028 | 10% | ||
mrtv2 | 0 | 53,442,385,401 | 100% | ||
steemtruth | 0 | 7,274,516,932 | 10% | ||
redes | 0 | 1,417,583,942,821 | 29% | ||
lalala | 0 | 75,575,123,866 | 100% | ||
devilwsy | 0 | 2,228,922,407 | 100% | ||
janiceting | 0 | 2,214,345,198 | 100% | ||
abraomarcos | 0 | 2,189,974,813 | 100% | ||
lydiachan | 0 | 20,926,068,712 | 100% | ||
newhope | 0 | 1,895,940,501,852 | 24% | ||
dragon40 | 0 | 2,010,516,022 | 10% | ||
blackbunny | 0 | 80,416,322,225 | 100% | ||
bxt | 0 | 156,142,716,298 | 100% | ||
lingfei | 0 | 51,680,072,574 | 100% | ||
yyyy | 0 | 453,028,674 | 100% | ||
austinsandersco | 0 | 758,068,096 | 70% | ||
kingofdew | 0 | 42,235,039,188 | 100% | ||
emcvay | 0 | 176,863,321 | 10% | ||
mandagoi | 0 | 2,703,049,113 | 21% | ||
wylo | 0 | 613,964,238 | 100% | ||
susanlo | 0 | 66,006,534,641 | 100% | ||
jkkim | 0 | 60,586,510 | 10% | ||
ebejammin | 0 | 5,309,638,896 | 100% | ||
nanosesame | 0 | 37,353,382,445 | 30% | ||
cryptohustler | 0 | 17,353,587,594 | 100% | ||
exec | 0 | 82,041,413,373 | 100% | ||
eval | 0 | 792,987,799 | 100% | ||
speeding | 0 | 3,706,109,707 | 100% | ||
walkinharmony | 0 | 15,264,025,972 | 50% | ||
asterix87 | 0 | 13,320,929,693 | 100% | ||
canbethisone | 0 | 6,482,108,878 | 50% | ||
abetterworld | 0 | 3,159,250,145 | 100% | ||
raili | 0 | 9,776,076,641 | 100% | ||
that1consultant | 0 | 302,353,392 | 100% | ||
sanzo | 0 | 285,909,900 | 100% | ||
davaowhenyo | 0 | 614,503,785 | 100% | ||
allenshayzar | 0 | 614,503,785 | 100% | ||
raku | 0 | 610,056,865 | 100% | ||
resteeming | 0 | 614,860,000 | 100% | ||
ravenousappetite | 0 | 614,503,440 | 100% | ||
aabb | 0 | 10,690,902,322 | 100% | ||
catwomanteresa | 0 | 41,581,675,865 | 40% | ||
auntigormint | 0 | 537,652,272 | 100% | ||
mrliga | 0 | 17,236,910,921 | 100% | ||
twinkledrop | 0 | 83,241,741,277 | 100% | ||
sweethoney | 0 | 253,884,794 | 100% | ||
liangfengyouren | 0 | 1,010,511,804 | 50% | ||
idx | 0 | 13,965,126,359 | 100% | ||
jiangchen | 0 | 9,788,826,771 | 100% | ||
lancy | 0 | 4,501,349,078 | 92% | ||
cn-reader | 0 | 63,453,963,832 | 52% | ||
bearpaw | 0 | 2,528,182,040 | 100% | ||
freedom-fighter | 0 | 614,503,785 | 100% | ||
eduter | 0 | 3,103,667,655 | 100% | ||
technologynepal | 0 | 613,122,478 | 100% | ||
davidke20 | 0 | 2,004,466,583 | 15% | ||
rosatravels | 0 | 39,872,702,197 | 20% | ||
dgorbunov | 0 | 553,202,119 | 100% | ||
khalilad | 0 | 606,090,200 | 100% | ||
ms8988 | 0 | 602,448,519 | 100% | ||
stakuza | 0 | 513,835,827 | 100% | ||
ikonik | 0 | 393,531,737 | 100% | ||
vfxness | 0 | 66,551,129 | 100% | ||
lemminon | 0 | 615,320,000 | 100% | ||
bobdos | 0 | 3,594,023,523 | 7% | ||
heyeshuang | 0 | 645,908,872 | 100% | ||
razor80 | 0 | 587,563,371 | 100% | ||
fastiduos | 0 | 615,320,000 | 100% | ||
winniex | 0 | 3,508,326,951 | 10% | ||
rebecca80 | 0 | 313,882,503 | 100% | ||
weavingwords | 0 | 57,058,949,479 | 100% | ||
nitro.live | 0 | 3,136,359,099 | 100% | ||
chaerin | 0 | 429,359,775 | 100% | ||
cnbuddy | 0 | 3,230,372,395 | 0.1% | ||
chann | 0 | 2,533,062,842 | 10% | ||
yammyamm | 0 | 290,833,854 | 100% | ||
zilin | 0 | 573,137,451 | 100% | ||
lebin | 0 | 31,871,077,952 | 30% | ||
coindzs | 0 | 130,786,275 | 100% | ||
saury | 0 | 303,723,844 | 100% | ||
patrickzhou | 0 | 13,583,052,759 | 100% | ||
ewq | 0 | 745,289,769 | 3% | ||
roundbeargames | 0 | 10,979,111,071 | 10% | ||
maiyude | 0 | 10,955,338,495 | 10% | ||
superoo7 | 0 | 4,735,384,385 | 24% | ||
yumisee | 0 | 330,420,351 | 20% | ||
joeliew | 0 | 280,485,961 | 6% | ||
benytg | 0 | 1,848,719,134 | 100% | ||
vamos-amigo | 0 | 1,690,853,546 | 40% | ||
mycat | 0 | 153,383,045 | 12% | ||
breezieblack | 0 | 258,100,107 | 100% | ||
iipoh06 | 0 | 68,629,099 | 10% | ||
historylover | 0 | 410,954,629 | 100% | ||
annabellenoelle | 0 | 1,171,234,063 | 20% | ||
btccurrency1 | 0 | 55,103,324 | 100% | ||
ethanlee | 0 | 10,442,237,463 | 100% | ||
kasperr | 0 | 106,088,453 | 100% | ||
fishbb | 0 | 847,139,835 | 5% | ||
fanso | 0 | 1,178,555,353 | 100% | ||
cryptoknight27 | 0 | 615,076,421 | 100% | ||
abss | 0 | 92,666,914 | 11% | ||
hepeng.chn | 0 | 147,372,377 | 100% | ||
ashley4u | 0 | 0 | 0% | ||
kumerbakul | 0 | 56,229,670 | 9% | ||
xpp | 0 | 614,007,415 | 100% | ||
paulobassman | 0 | 3,068,305,551 | 100% | ||
jiahua | 0 | 592,619,760 | 100% | ||
xiaoyuanwmm | 0 | 308,117,657 | 100% | ||
dangtmaximun | 0 | 399,281,964 | 100% | ||
steemsla | 0 | 50,337,464 | 100% | ||
astros | 0 | 1,860,632,751 | 100% | ||
fanvideo | 0 | 126,028,931 | 100% | ||
lovelu3650 | 0 | 217,131,139 | 100% | ||
bastianrt | 0 | 318,025,265 | 100% | ||
samirphuyal | 0 | 424,843,197 | 100% |
the content of programming is awesome. excellent context.
post_id | 44,448,485 |
---|---|
author | dangtmaximun |
permlink | re-oflyhigh-4ckfhr-python-20180420t024627885z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-20 02:47:03 |
last_update | 2018-04-20 02:47:03 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-27 02:47:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 57 |
author_reputation | 68,478,707,640 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hey, just wanted to let you know I gave you an upvote because I appreciate your content! =D See you around
post_id | 44,455,953 |
---|---|
author | greentomorrow |
permlink | re-oflyhigh-4ckfhr-python-20180420t035805954z |
category | python |
json_metadata | {} |
created | 2018-04-20 03:58:06 |
last_update | 2018-04-20 03:58:06 |
depth | 1 |
children | 1 |
net_rshares | -32,076,498,836 |
last_payout | 2018-04-27 03:58:06 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 107 |
author_reputation | -132,163,372,139 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
oflyhigh | 0 | -30,873,023,158 | -1% | ||
neutralizer | 0 | -1,203,475,678 | -20% |
# Be advised @oflyhigh The comment from @greentomorrow is far from being sincere. It has been identified as being copy/pasted comment spam intended to trick their targets into upvoting them. Please, refrain from doing so. They have been reported to @steemcleaners as well as downvoted and we are giving people a heads-up until they are dealt with. Thank you!
post_id | 44,506,346 |
---|---|
author | neutralizer |
permlink | re-re-oflyhigh-4ckfhr-python-20180420t035805954z-20180420t112828 |
category | python |
json_metadata | {} |
created | 2018-04-20 11:28:30 |
last_update | 2018-04-20 11:28:30 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-27 11:28:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 359 |
author_reputation | 602,559,586,074 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
😥😥😥😥
post_id | 44,460,945 |
---|---|
author | steemit.lover |
permlink | re-oflyhigh-4ckfhr-python-20180420t044554129z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-20 04:46:00 |
last_update | 2018-04-20 04:46:00 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-27 04:46:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 4 |
author_reputation | 1,104,926,356 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
像你学习,每天进步一点点
post_id | 44,461,448 |
---|---|
author | tsinrong |
permlink | re-oflyhigh-4ckfhr-python-20180420t045057755z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-20 04:50:57 |
last_update | 2018-04-20 04:50:57 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-27 04:50:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 12 |
author_reputation | 92,139,201,544 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
可能是玩functional太多了,当我写java和python都会用Lambda Python 3 ``` def increase (n): return lambda x: x + n vals = increase([1]) print(vals(2)) ``` 然后在lambda都能用filter,map和reduce
post_id | 44,529,963 |
---|---|
author | superoo7 |
permlink | re-oflyhigh-4ckfhr-python-20180420t142319180z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-20 14:23:21 |
last_update | 2018-04-20 14:27:57 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-27 14:23:21 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 167 |
author_reputation | 25,572,770,303,018 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
一直很想学python,但是是懒癌患者,略有C#经验和各类C的基础,请教下有没有适合有点变成经验的学习资料(教科书式的一大篇有的没的看不太下去~)
post_id | 44,926,701 |
---|---|
author | shine.wong |
permlink | re-oflyhigh-4ckfhr-python-20180423t023418369z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-23 02:34:24 |
last_update | 2018-04-23 02:34:24 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-04-30 02:34:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 73 |
author_reputation | 1,373,339,077,399 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
最近新出的语言包括其他语言的升级很多都把重点放在了函数式上面,将函数作为一种类型来处理变得越来越流行了。
post_id | 45,308,975 |
---|---|
author | objc0 |
permlink | re-oflyhigh-4ckfhr-python-20180425t083446288z |
category | python |
json_metadata | "{"app": "steemit/0.1", "tags": ["python"]}" |
created | 2018-04-25 08:34:48 |
last_update | 2018-04-25 08:34:48 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2018-05-02 08:34:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 52 |
author_reputation | 3,458,508,830 |
root_title | 每天进步一点点:Python中使用函数作为参数 |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |