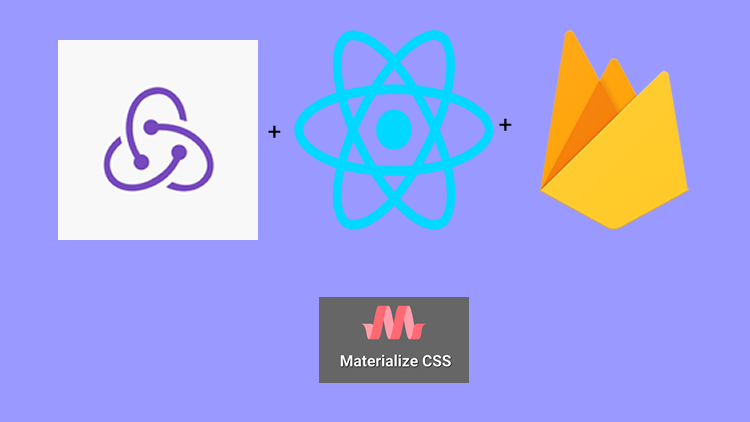 # Repository ### React https://github.com/facebook/react ### Material-ui https://github.com/mui-org/material-ui ### My Github Address https://github.com/pckurdu ### This Project Github Address https://github.com/pckurdu/Build-A-Blog-Site-With-React-Redux-Firebase-And-MaterializeCSS-Part-8- # What Will I Learn? - You will learn how to perform firebase auth operations using the redux. - You will learn the callback functions of signInWithEmailAndPassword (). - You will learn to access redux action in react component. - You will learn to process the reducer operations and store data to the props in the react component. - You will learn to update the state's unknown object with the setState () function # Requirements - text editor (I used visual studio code editor) - Basic javascript information - Basic react information # Difficulty - Basic # Tutorial Contents In this tutorial we will perform sign in and sign out operations using `redux`. We will create a action for the user to login. In action, we will create the necessary control environment and communicate with the `reducer`.When exporting the reducer to the store, we will provide the login control from the firebase with the action. As a result of these transactions we will have information that the user is logged in or not but first we need to activate the component in which the user can enter their information. We created the signIn component in the previous tutorials. In this tutorial we will learn how to access the user-entered inputs. After getting the user's login information, we will communicate with reducer and action in the signIn component. We will examine which values are coming to the signIn component and we will complete our code according to the information. We will perform the sign out process after performing the login process. For the sign out process, we will define another action and in this action we will perform the firebase exit with the firebase function. We will do the action control with the reducer and forward the sign out information to the store. This tutorial is divided into headings as follows: - Create Login Action - Activate SignIn Component - Access Actions From SignIn Component - Access Reducer and Store From SignIn Component - Control of Login Processes Let’ start and enjoy. ### 1-Create Login Action We created the projectActions.js file to add data to firestore. Since we will perform authentication, we create another file. Under the `actions` folder, we will create the `authActions.js` file. We will define a function in this file and use this function as action on the reducer side. Since this function will check the user information, it should take the input information with a parameter. #### In authActions.js ``` //An action called logIn export const logIn=(information)=>{ return (dispatch,getState,{getFirebase})=>{ //We're accessing the firebase const firebase = getFirebase(); //Email and Password method to check the login. firebase.auth().signInWithEmailAndPassword( information.email, information.password ) } } ``` <br> We defined `getFirebase()` in `index.js`.Using `getFirebase()`, we can access the firebase and use the functions in it. The `auth ()` function in the firebase contains functions to perform authentication operations. We'll use the `signInWithEmailAndPassword()` function because we're activating the Email / Password login method. 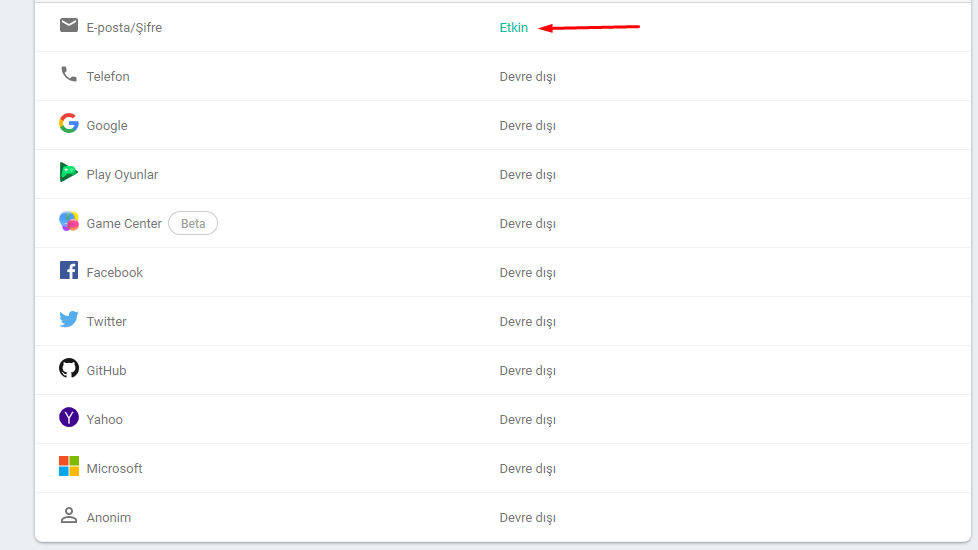 <br> `signInWithEmailAndPassword ()` returns whether the user is logged on by email or password. If the operation is successful, we can catch it with the `then()` function. If the login process is wrong or if the error occurred during the connection to the firebase, we will be notified by the `catch()` function. #### In authActions.js ``` //Email and Password method to check the login. firebase.auth().signInWithEmailAndPassword( information.email, information.password //if login is successful ).then(()=>{ dispatch({type:'LOGIN_SUCCESS'}); //if login fails }).catch((err)=>{ dispatch({type:'LOGIN_ERROR',err}); }); ``` <br> As we complete the logIn action, we can switch to the reducer control. We have created the `authReducer.js` file before. In this reducer, we will check the type of action and keep a variable with the help of error. #### In authReducer.js ``` //default data is used if no state data is assigned. const initState={ //error variable authError:null } ``` <br> We will do according to the action type of the state and authError variable. ``` //create reducer with state and action parameters const authReducer=(state=initState,action)=>{ switch (action.type){ case 'LOGIN_ERROR': console.log('login error'); //Importing authError in state return{ ...state, authError:'login failed' } case 'LOGIN_SUCCESS': console.log('login success'); return{ ...state, authError:null } default: return state; } } ``` <br> The reducer does not know which action is running and controls all actions. We can use action according to the type of action. Now the reducer knows the result of the firebase signIn process. Using the components reducer, we can learn the result of the signIn process and do the operations accordingly. Then let's prepare to use this reducer in the signIn component. ### 2- Activate SignIn Component We have previously designed this page. 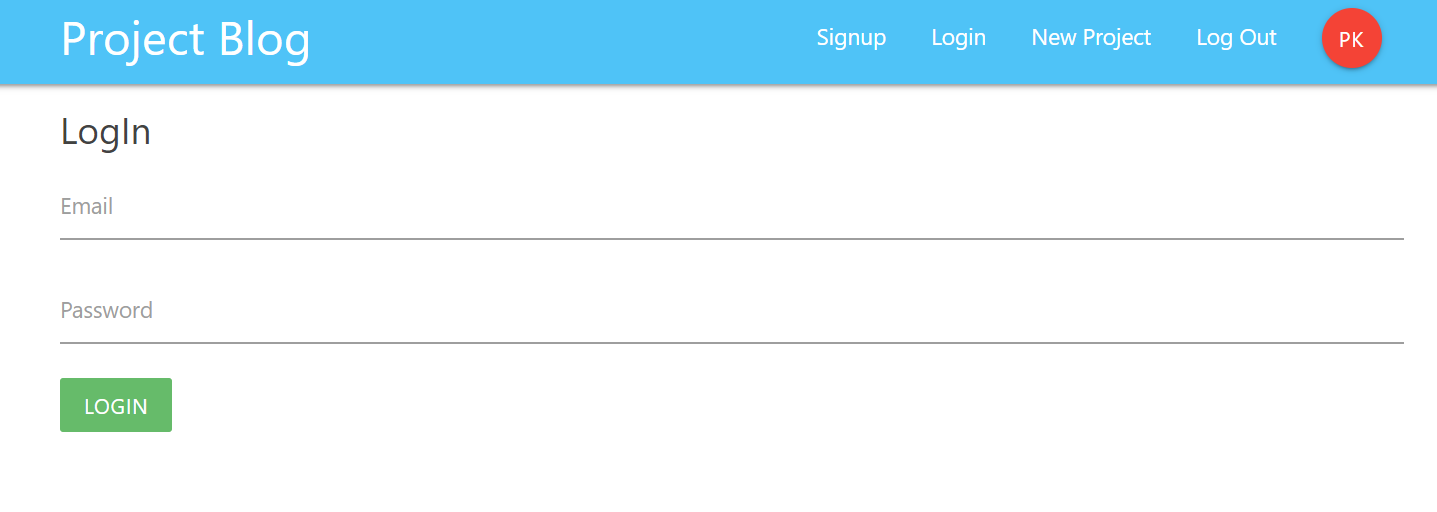 <br> Let's enter the values entered into the input and push the button into the state of this component. For these operations, we must first define the `state`.This state must be created to keep the state `email` and `password`. ### In SignIn.js ``` class SignIn extends Component { //for inputs state = { email: '', password: '' } ``` <br> As input values change, we must update these state values. For this we must trigger the input `onChange` event and define a function to run when triggered. ``` //will work when the input is changed. handleChange = (e) => { this.setState({ [e.target.id]: e.target.value }) } … {/* for email */} <div className="input-field"> <label htmlFor="email">Email</label> <input type="email" id='email' onChange={this.handleChange} /> </div> {/* for password */} <div className="input-field"> <label htmlFor="password">Password</label> <input type="password" id='password' onChange={this.handleChange} /> </div> ``` <br> We used the `setState()` function to perform the state update. On this page we have defined two inputs and we used `e.target.value` to detect which input has changed. Thus the values in the inputs will be equal to the state. When the submit event of the form is triggered, it will be retaining the final state of the state inputs. Let's create a function to trigger when the submit event occurs. ``` //will work when the form is submit. handleSubmit = (e) => { e.preventDefault(); console.log(this.state); } … <form className="white" onSubmit={this.handleSubmit}> ``` <br> So we can access the input values. 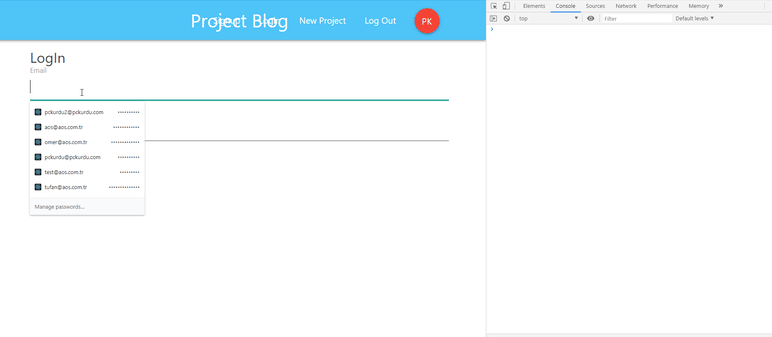 <br> The input information appears on the console screen.  <br> Now signIn component reducer is ready for use. ### 3- Access Actions From SignIn Component Since we are going to connect to the reducer and store within the signIn component, we must use the `connect` module. We must access the `logIn action` we created at the moment of connection. So we can run this action and control the firebase auth. #### In SignIn.js ``` //To access store and reducer import {connect} from 'react-redux'; //To access action import {logIn} from '../../store/actions/authActions'; ``` <br> Let's create a function to access action from component. We should use this function for the second parameter of the connect function. The first parameter of the connect module accesses the store and we access the action with the second parameter. ``` //Function to access action const mapDispatchToProps=(dispatch)=>{ return{ signIn:(info)=>dispatch(logIn(info)) } } export default connect(null,mapDispatchToProps)(SignIn) ``` <br> The generated `sigIn` represents the logIn function in action. With this function we can access this signIn with component props. Then when we submit it, we can run this action with the information in the state. ``` //will work when the form is submit. handleSubmit = (e) => { e.preventDefault(); //action function this.props.signIn(this.state); } ``` <br> So the logIn function in action will work but the component does not know the result of the transaction. ### 4- Access Reducer and Store From SignIn Component We have defined a variable called `authError` so that we can know the result of the process. We were keeping this variable in store. Then let's access the store in the signIn component and edit the page according to the authError variable. ``` //Function to access store const mapStateToProps=(state)=>{ return { authError:state.auth.authError } } export default connect(mapStateToProps,mapDispatchToProps)(SignIn) ``` <br> With the help of such a function, we have received the authError data from the store on the props of the page. ``` render() { const {authError}=this.props; return ( ``` <br> We can now give a warning if we are making an incorrect entry at the bottom of the page. ``` {/* for submit */} <div className="input-field"> <button className="btn green lighten-1 z-depth-0">Login</button> <div className="red-text center"> {/* If there is data in authError, it means that it has received an error. */} {authError ? <p>{authError}</p>: null} </div> </div> ``` <br> We can control the operations as we finished coding. ### 5- Control of Login Processes 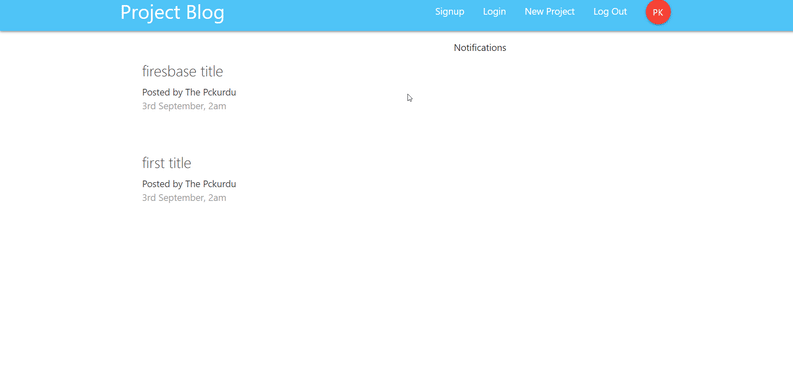 <br> If you enter the wrong user name or password on the login screen, the `authError` value will appear on the screen. 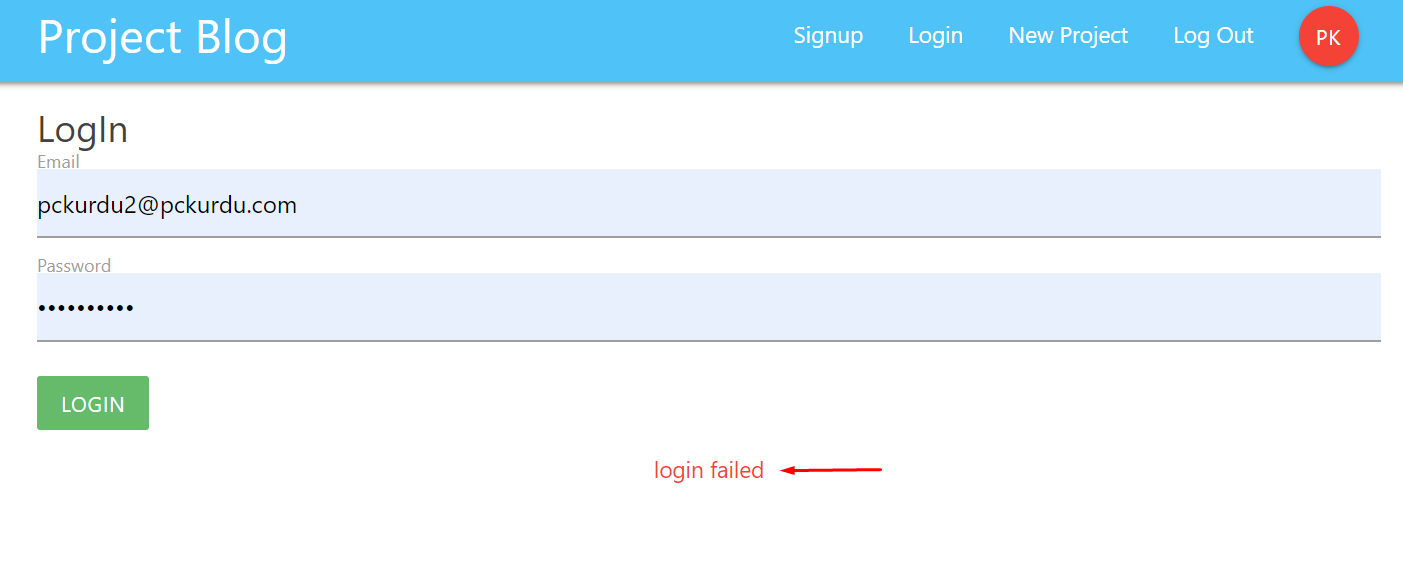 <br> We will not include user information in the firebase value in the store that we wrote to the console. If you type the user name and password correctly, the user information will be filled in the firebase value. 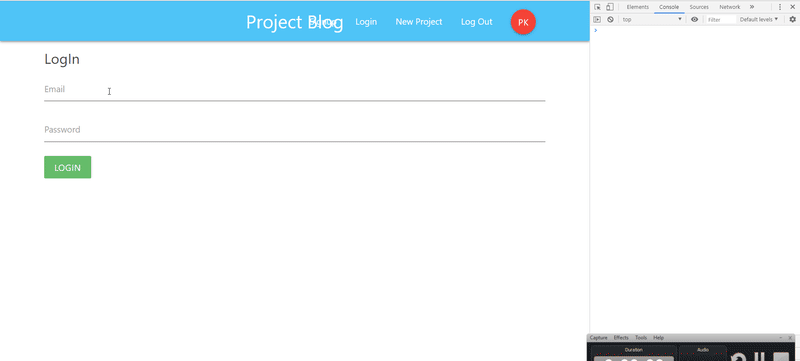 <br> and 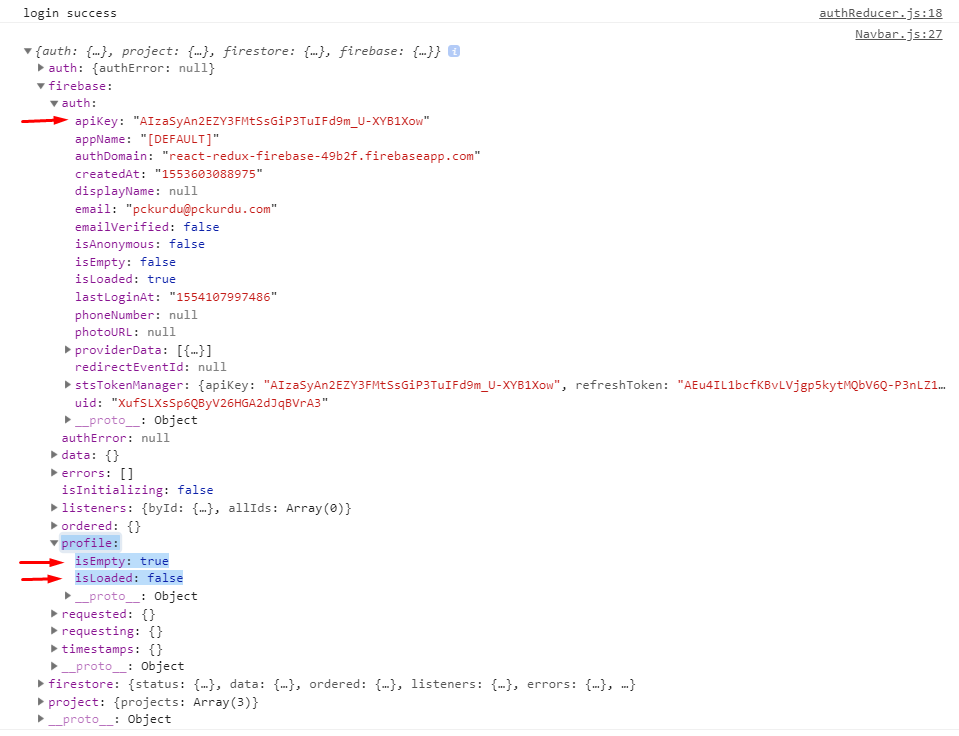 <br> # Curriculum https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-1 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-2 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-3 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-4 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-5 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-6 https://steemit.com/utopian-io/@pckurdu/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-7 # Proof of Work Done https://github.com/pckurdu/Build-A-Blog-Site-With-React-Redux-Firebase-And-MaterializeCSS-Part-8-
post_id | 72,377,983 |
---|---|
author | pckurdu |
permlink | build-a-blog-site-with-react-redux-firebase-and-materializecss-part-8 |
category | utopian-io |
json_metadata | {"tags":["utopian-io","tutorials","react","redux","firebase"],"image":["https:\/\/cdn.steemitimages.com\/DQmRpmFiQxCwe4jswNSCADhUgLnyH8Ce249cszhzrYXLGvC\/react-redux.fw.png","https:\/\/cdn.steemitimages.com\/DQmeB5KpSezxEZbVkk9sYhqJa36GsrDqd2MMVhrmkT8j3NW\/react1.png","https:\/\/cdn.steemitimages.com\/DQmcga18bspMstXDegGHAjjfNrujrHUsCPifyJVZM45DEGk\/react2.png","https:\/\/cdn.steemitimages.com\/DQmRqc4HLmpJTvafy2grM4SBmEiPNdMSX4K3Za1DHReXJ8J\/react1.gif","https:\/\/cdn.steemitimages.com\/DQmV56ZMd8eeXoVagvMxw9d7iVHFcebtf1fyDws9UKo1HFk\/react3.png","https:\/\/cdn.steemitimages.com\/DQmbYnDPmjwnfJjbnFHQWb5Y1fErF5oU4Wjm2zBiTZJXUw5\/react2.gif","https:\/\/cdn.steemitimages.com\/DQmXancfNoFjDfHw4WDFn2c4e2VUYQqNh5uFtRcF6YUodBG\/react4.png","https:\/\/cdn.steemitimages.com\/DQmdgofGTshiUKj6xBGbMM52dTGR7Z1uWcuaDa1PqVMU4Gf\/react3.gif","https:\/\/cdn.steemitimages.com\/DQmbWZQkN72sPN6oGGXufUEFmGUq7dexqqX2UA1t5AP7PNR\/react5.png"],"links":["https:\/\/github.com\/facebook\/react","https:\/\/github.com\/mui-org\/material-ui","https:\/\/github.com\/pckurdu","https:\/\/github.com\/pckurdu\/Build-A-Blog-Site-With-React-Redux-Firebase-And-MaterializeCSS-Part-8-","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-1","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-2","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-3","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-4","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-5","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-6","https:\/\/steemit.com\/utopian-io\/@pckurdu\/build-a-blog-site-with-react-redux-firebase-and-materializecss-part-7"],"app":"steemit\/0.1","format":"markdown"} |
created | 2019-04-01 13:01:18 |
last_update | 2019-04-01 13:01:18 |
depth | 0 |
children | 5 |
net_rshares | 49,126,574,373,328 |
last_payout | 2019-04-08 13:01:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 27.704 SBD |
curator_payout_value | 8.779 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 12,216 |
author_reputation | 23,382,389,405,576 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
tombstone | 0 | 3,216,696,584,478 | 13.22% | ||
rufans | 0 | 21,466,363,957 | 100% | ||
abh12345 | 0 | 9,263,135,964 | 1.65% | ||
techslut | 0 | 83,862,061,048 | 20% | ||
minersean | 0 | 8,103,730,017 | 75% | ||
erikaflynn | 0 | 13,751,961,004 | 35% | ||
miniature-tiger | 0 | 101,058,663,075 | 50% | ||
jakipatryk | 0 | 14,736,151,742 | 50% | ||
jga | 0 | 2,222,729,265 | 16.52% | ||
helo | 0 | 76,911,019,766 | 31.47% | ||
walnut1 | 0 | 23,761,989,613 | 16.52% | ||
lorenzor | 0 | 928,537,166 | 8.26% | ||
suesa | 0 | 90,673,476,214 | 25% | ||
tensor | 0 | 43,271,229,696 | 100% | ||
codingdefined | 0 | 44,041,263,533 | 31.47% | ||
tsoldovieri | 0 | 1,175,575,391 | 8.26% | ||
tykee | 0 | 10,593,829,572 | 16.52% | ||
iamphysical | 0 | 15,864,484,427 | 90% | ||
felixrodriguez | 0 | 543,972,668 | 5.78% | ||
azulear | 0 | 494,589,041 | 100% | ||
rehan12 | 0 | 10,366,719,052 | 3.3% | ||
silviu93 | 0 | 4,304,097,599 | 16.52% | ||
jadabug | 0 | 1,749,909,989 | 1% | ||
dakeshi | 0 | 1,146,648,902 | 16.52% | ||
crokkon | 0 | 64,238,364,256 | 50% | ||
eastmael | 0 | 6,408,883,799 | 33.05% | ||
espoem | 0 | 57,903,241,162 | 28.64% | ||
mcfarhat | 0 | 24,500,886,002 | 12.58% | ||
vishalsingh4997 | 0 | 130,714,032 | 16.52% | ||
elear | 0 | 5,296,745,356 | 33.05% | ||
zoneboy | 0 | 22,183,607,234 | 100% | ||
carloserp-2000 | 0 | 33,576,430,633 | 100% | ||
carlos84 | 0 | 894,413,125 | 16.52% | ||
che-shyr | 0 | 1,003,186,140 | 50% | ||
utopian-io | 0 | 43,558,445,438,161 | 33.05% | ||
imisstheoldkanye | 0 | 1,365,307,394 | 1% | ||
jaff8 | 0 | 70,505,147,147 | 31.47% | ||
amestyj | 0 | 707,383,998 | 16.52% | ||
iqbaladan | 0 | 5,634,861,720 | 33.05% | ||
mcyusuf | 0 | 1,992,308,586 | 16.52% | ||
alexs1320 | 0 | 44,836,628,218 | 25% | ||
gentleshaid | 0 | 29,240,207,717 | 33.05% | ||
steemitag | 0 | 3,393,909,402 | 10% | ||
cpufronz | 0 | 1,015,840,625 | 50% | ||
ivymalifred | 0 | 289,848,781 | 8.26% | ||
aussieninja | 0 | 5,107,935,454 | 16.52% | ||
ennyta | 0 | 113,302,670 | 8.26% | ||
amosbastian | 0 | 107,684,684,071 | 31.47% | ||
eliaschess333 | 0 | 1,589,124,416 | 8.26% | ||
harry-heightz | 0 | 13,185,426,119 | 33.05% | ||
scienceangel | 0 | 62,873,273,912 | 50% | ||
portugalcoin | 0 | 16,534,298,465 | 15% | ||
vanarchist | 0 | 2,821,404,592 | 100% | ||
sargoon | 0 | 1,079,790,182 | 16.52% | ||
tobias-g | 0 | 143,336,749,852 | 38% | ||
osazuisdela | 0 | 192,152,407 | 15% | ||
miguelangel2801 | 0 | 88,578,751 | 8.26% | ||
didic | 0 | 30,449,380,456 | 25% | ||
emiliomoron | 0 | 620,311,578 | 8.26% | ||
nenya | 0 | 1,677,731,056 | 79% | ||
ulisesfl17 | 0 | 1,857,152,530 | 100% | ||
arac | 0 | 980,270,119 | 100% | ||
endopediatria | 0 | 72,631,585 | 3.3% | ||
fego | 0 | 26,153,857,364 | 31.47% | ||
properfraction | 0 | 2,626,731,277 | 100% | ||
tomastonyperez | 0 | 1,889,084,159 | 8.26% | ||
elvigia | 0 | 1,659,442,884 | 8.26% | ||
jubreal | 0 | 3,241,237,806 | 33.05% | ||
ezravandi | 0 | 3,264,553,071 | 1% | ||
yu-stem | 0 | 10,810,622,604 | 25% | ||
luiscd8a | 0 | 1,658,198,048 | 80% | ||
eniolw | 0 | 8,417,923,571 | 100% | ||
geadriana | 0 | 77,048,364 | 2.47% | ||
josedelacruz | 0 | 784,446,379 | 8.26% | ||
joseangelvs | 0 | 236,476,203 | 16.52% | ||
viannis | 0 | 221,695,714 | 8.26% | ||
rollthedice | 0 | 3,575,626,803 | 33.05% | ||
flores39 | 0 | 393,478,923 | 100% | ||
loukosporbola | 0 | 4,162,223,984 | 27% | ||
erickyoussif | 0 | 372,108,408 | 16.52% | ||
yeee | 0 | 344,383,998 | 100% | ||
beetlevc | 0 | 1,300,836,487 | 2% | ||
isabelll | 0 | 345,245,816 | 100% | ||
michael44 | 0 | 343,879,325 | 100% | ||
seb3364 | 0 | 343,729,567 | 100% | ||
rii | 0 | 343,548,613 | 100% | ||
sjennifer | 0 | 342,977,556 | 100% | ||
m3ik3 | 0 | 343,125,670 | 100% | ||
apt-get | 0 | 344,665,443 | 100% | ||
kleinheim | 0 | 343,329,140 | 100% | ||
anaestrada12 | 0 | 3,221,826,156 | 16.52% | ||
kraggan | 0 | 342,365,548 | 100% | ||
joelsegovia | 0 | 584,310,366 | 8.26% | ||
lumix | 0 | 341,246,285 | 100% | ||
jesusfl17 | 0 | 396,377,680 | 100% | ||
bflanagin | 0 | 2,738,468,011 | 16.52% | ||
ubaldonet | 0 | 3,206,912,727 | 65% | ||
phelrenew | 0 | 541,790,198 | 100% | ||
madel | 0 | 343,405,323 | 100% | ||
house-targaryen | 0 | 342,826,889 | 100% | ||
dalz | 0 | 4,369,085,185 | 13.22% | ||
ulockblock | 0 | 42,308,858,962 | 12.71% | ||
amart29 | 0 | 273,601,738 | 3.3% | ||
jk6276 | 0 | 5,021,285,906 | 16.52% | ||
fronoguclo | 0 | 525,808,019 | 100% | ||
clusonsopi | 0 | 533,970,511 | 100% | ||
enycproxde | 0 | 534,107,117 | 100% | ||
disfarmthreelxe | 0 | 550,506,215 | 100% | ||
kornhafvakim | 0 | 537,064,956 | 100% | ||
heimapowcu | 0 | 519,242,150 | 100% | ||
tradhornspefring | 0 | 542,054,090 | 100% | ||
savannah6em | 0 | 541,608,941 | 100% | ||
emma0tx09 | 0 | 550,852,703 | 100% | ||
brianna3s08q | 0 | 552,740,834 | 100% | ||
savannahbs02 | 0 | 535,405,785 | 100% | ||
steemchoose | 0 | 7,802,843,021 | 10.74% | ||
dssdsds | 0 | 1,907,852,545 | 16.52% | ||
jayplayco | 0 | 62,587,656,707 | 16.52% | ||
cryptouno | 0 | 480,890,006 | 5% | ||
pckurdu | 0 | 10,902,832,717 | 100% | ||
lupafilotaxia | 0 | 14,355,640,780 | 100% | ||
fran.frey | 0 | 258,147,806 | 8.26% | ||
alaiza | 0 | 458,560,308 | 100% | ||
mops2e | 0 | 312,169,008 | 22.91% | ||
jrevilla | 0 | 62,913,505 | 16.52% | ||
nataliemg | 0 | 534,396,967 | 100% | ||
swapsteem | 0 | 5,422,324,622 | 16.52% | ||
samuelgr | 0 | 344,964,419 | 100% | ||
allisonot7 | 0 | 536,510,812 | 100% | ||
stem-espanol | 0 | 11,096,653,567 | 16.52% | ||
faithmso0b | 0 | 533,605,850 | 100% | ||
lapp | 0 | 457,352,789 | 100% | ||
steemtpistia | 0 | 460,322,203 | 100% | ||
crassipes | 0 | 460,559,980 | 100% | ||
persventacons | 0 | 543,358,560 | 100% | ||
remarggore | 0 | 551,522,835 | 100% | ||
mattuhedo | 0 | 543,498,479 | 100% | ||
ornorodi1986 | 0 | 551,082,114 | 100% | ||
truetinker | 0 | 343,749,574 | 100% | ||
tokyoduck | 0 | 343,165,491 | 100% | ||
potsdam | 0 | 343,968,418 | 100% | ||
awesome-n | 0 | 343,542,335 | 100% | ||
agrovision | 0 | 458,322,722 | 100% | ||
merlin7 | 0 | 42,760,803,345 | 2.2% | ||
steem-ua | 0 | 601,824,190,650 | 6% | ||
depaldelta | 0 | 341,770,145 | 100% | ||
giulyfarci52 | 0 | 131,915,821 | 8.26% | ||
kylieeytme | 0 | 530,312,865 | 100% | ||
multibeam | 0 | 343,532,648 | 100% | ||
kaczynski | 0 | 173,129,685 | 100% | ||
nijn | 0 | 875,477,101 | 79% | ||
quenty | 0 | 2,352,747,224 | 79% | ||
hdu | 0 | 998,482,665 | 1% | ||
steemexpress | 0 | 1,601,319,008 | 2.81% | ||
alex-hm | 0 | 1,521,039,293 | 50% | ||
bluesniper | 0 | 265,959,557 | 0.1% | ||
mrsbozz | 0 | 670,608,415 | 25% | ||
ascorphat | 0 | 2,129,360,565 | 2.5% | ||
nimloth | 0 | 4,057,587,391 | 79% | ||
rewarding | 0 | 4,960,166,478 | 66.52% | ||
jk6276.mons | 0 | 979,096,523 | 33.05% | ||
hamsa.quality | 0 | 834,323,443 | 1% | ||
jaxson2011 | 0 | 1,261,657,552 | 33.05% | ||
eternalinferno | 0 | 148,955,691 | 33.05% | ||
depalalfa | 0 | 0 | 50% | ||
sengoutim | 0 | 490,243,608 | 100% | ||
itamow | 0 | 490,253,601 | 100% | ||
ofiste | 0 | 490,237,685 | 100% | ||
rambleroid | 0 | 490,240,191 | 100% | ||
eflem | 0 | 490,152,464 | 100% | ||
larindisi | 0 | 490,153,817 | 100% | ||
orert | 0 | 490,152,818 | 100% | ||
toficene | 0 | 490,149,675 | 100% | ||
sportm | 0 | 490,156,259 | 100% | ||
ritimim | 0 | 490,156,019 | 100% | ||
ondire | 0 | 490,153,652 | 100% | ||
oweadi | 0 | 490,151,004 | 100% | ||
ondat | 0 | 490,085,128 | 100% | ||
ucomo | 0 | 490,065,840 | 100% | ||
asedint | 0 | 490,055,639 | 100% | ||
iterithof | 0 | 490,055,450 | 100% | ||
inallets | 0 | 489,825,851 | 100% | ||
teatickic | 0 | 489,498,051 | 100% | ||
esurell | 0 | 489,491,254 | 100% | ||
lemorn | 0 | 489,488,885 | 100% | ||
yavasu | 0 | 489,500,505 | 100% | ||
uldomyo | 0 | 489,485,434 | 100% | ||
darrorir | 0 | 490,061,395 | 100% | ||
iteneneda | 0 | 489,958,114 | 100% | ||
odended | 0 | 489,861,400 | 100% | ||
ofresesh | 0 | 489,737,791 | 100% | ||
sillim | 0 | 489,925,995 | 100% | ||
orat | 0 | 489,878,958 | 100% | ||
erinesine | 0 | 489,787,366 | 100% | ||
inoushi | 0 | 489,446,707 | 100% | ||
noraron | 0 | 489,364,867 | 100% | ||
disase | 0 | 490,044,545 | 100% | ||
perendont | 0 | 490,032,626 | 100% | ||
saitoupli | 0 | 489,437,819 | 100% | ||
saratyom | 0 | 489,430,417 | 100% | ||
reror | 0 | 489,359,485 | 100% | ||
isals | 0 | 489,432,454 | 100% | ||
fofladi | 0 | 489,346,987 | 100% | ||
iplex | 0 | 489,437,235 | 100% | ||
etrilers | 0 | 489,432,940 | 100% | ||
athate | 0 | 489,434,654 | 100% | ||
eytaltul | 0 | 489,508,073 | 100% | ||
etadgitu | 0 | 489,929,378 | 100% | ||
isaterito | 0 | 489,927,036 | 100% | ||
boserr | 0 | 489,928,532 | 100% | ||
abastupo | 0 | 490,117,849 | 100% | ||
bindelam | 0 | 489,723,540 | 100% | ||
owiteal | 0 | 489,698,591 | 100% | ||
utopian.trail | 0 | 13,204,132,830 | 33.05% | ||
tenyears | 0 | 540,650,603 | 100% | ||
dicetime | 0 | 32,844,273,752 | 16.52% |
Thank you for your contribution @pckurdu. - Your tutorials are getting better and better. Excellent work in structuring the tutorial and explaining the code, with several GIFs with the demonstration of their results throughout the contribution. - The section of your curriculum begins to get big in the contribution, could put for example: <b>Curriculum</b>: <a href="#">Part1</a>, <a href="#">Part2</a>... Thank you for your work in developing this tutorial. Looking forward to your upcoming tutorials. Your contribution has been evaluated according to [Utopian policies and guidelines](https://join.utopian.io/guidelines), as well as a predefined set of questions pertaining to the category. To view those questions and the relevant answers related to your post, [click here](https://review.utopian.io/result/8/2-1-1-1-1-3-1-3-). ---- Need help? Chat with us on [Discord](https://discord.gg/uTyJkNm). [[utopian-moderator]](https://join.utopian.io/)
post_id | 72,398,233 |
---|---|
author | portugalcoin |
permlink | re-pckurdu-build-a-blog-site-with-react-redux-firebase-and-materializecss-part-8-20190401t212031255z |
category | utopian-io |
json_metadata | {"tags":["utopian-io"],"users":["pckurdu"],"links":["#","https:\/\/join.utopian.io\/guidelines","https:\/\/review.utopian.io\/result\/8\/2-1-1-1-1-3-1-3-","https:\/\/discord.gg\/uTyJkNm","https:\/\/join.utopian.io\/"],"app":"steemit\/0.1"} |
created | 2019-04-01 21:20:30 |
last_update | 2019-04-01 21:20:30 |
depth | 1 |
children | 1 |
net_rshares | 16,472,160,166,956 |
last_payout | 2019-04-08 21:20:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 9.174 SBD |
curator_payout_value | 2.917 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 958 |
author_reputation | 214,343,891,436,406 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
codingdefined | 0 | 27,606,432,158 | 20.35% | ||
espoem | 0 | 29,254,782,168 | 15% | ||
utopian-io | 0 | 16,011,302,101,567 | 11.17% | ||
jaff8 | 0 | 44,111,498,205 | 20.35% | ||
emrebeyler | 0 | 0 | 0.01% | ||
amosbastian | 0 | 67,382,422,273 | 20.35% | ||
organicgardener | 0 | 10,668,918,729 | 35% | ||
sudefteri | 0 | 5,934,417,600 | 100% | ||
reazuliqbal | 0 | 14,398,363,060 | 8% | ||
amico | 0 | 1,007,844,785 | 0.55% | ||
statsexpert | 0 | 8,976,341,494 | 100% | ||
mightypanda | 0 | 214,011,416,791 | 100% | ||
ulockblock | 0 | 24,489,556,257 | 7.24% | ||
fastandcurious | 0 | 4,090,285,046 | 100% | ||
curbot | 0 | 2,549,680,540 | 100% | ||
linknotfound | 0 | 471,188,319 | 100% | ||
ascorphat | 0 | 2,137,793,146 | 2.5% | ||
monster-inc | 0 | 3,236,100,989 | 100% | ||
holydog | 0 | 250,852,328 | 10% | ||
cleanit | 0 | 280,171,501 | 55% |
Thank you for your review, @portugalcoin! Keep up the good work!
post_id | 72,532,338 |
---|---|
author | utopian-io |
permlink | re-re-pckurdu-build-a-blog-site-with-react-redux-firebase-and-materializecss-part-8-20190401t212031255z-20190404t132034z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.17"} |
created | 2019-04-04 13:20:36 |
last_update | 2019-04-04 13:20:36 |
depth | 2 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-04-11 13:20:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 64 |
author_reputation | 152,913,012,544,965 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
#### Hi @pckurdu! Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation! Your post is eligible for our upvote, thanks to our collaboration with @utopian-io! **Feel free to join our [@steem-ua Discord server](https://discord.gg/KpBNYGz)**
post_id | 72,403,377 |
---|---|
author | steem-ua |
permlink | re-build-a-blog-site-with-react-redux-firebase-and-materializecss-part-8-20190401t234443z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.19"} |
created | 2019-04-01 23:44:45 |
last_update | 2019-04-01 23:44:45 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-04-08 23:44:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 286 |
author_reputation | 23,203,609,903,979 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Congratulations @pckurdu! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) : <table><tr><td>https://steemitimages.com/60x70/http://steemitboard.com/@pckurdu/voted.png?201904012310</td><td>You received more than 3000 upvotes. Your next target is to reach 4000 upvotes.</td></tr> </table> <sub>_You can view [your badges on your Steem Board](https://steemitboard.com/@pckurdu) and compare to others on the [Steem Ranking](http://steemitboard.com/ranking/index.php?name=pckurdu)_</sub> <sub>_If you no longer want to receive notifications, reply to this comment with the word_ `STOP`</sub> ###### [Vote for @Steemitboard as a witness](https://v2.steemconnect.com/sign/account-witness-vote?witness=steemitboard&approve=1) to get one more award and increased upvotes!
post_id | 72,403,558 |
---|---|
author | steemitboard |
permlink | steemitboard-notify-pckurdu-20190401t234759000z |
category | utopian-io |
json_metadata | {"image":["https:\/\/steemitboard.com\/img\/notify.png"]} |
created | 2019-04-01 23:48:00 |
last_update | 2019-04-01 23:48:00 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-04-08 23:48:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 828 |
author_reputation | 38,705,954,145,809 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |
Hey, @pckurdu! **Thanks for contributing on Utopian**. We’re already looking forward to your next contribution! **Get higher incentives and support Utopian.io!** Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via [SteemPlus](https://chrome.google.com/webstore/detail/steemplus/mjbkjgcplmaneajhcbegoffkedeankaj?hl=en) or [Steeditor](https://steeditor.app)). **Want to chat? Join us on Discord https://discord.gg/h52nFrV.** <a href='https://steemconnect.com/sign/account-witness-vote?witness=utopian-io&approve=1'>Vote for Utopian Witness!</a>
post_id | 72,408,142 |
---|---|
author | utopian-io |
permlink | re-build-a-blog-site-with-react-redux-firebase-and-materializecss-part-8-20190402t014609z |
category | utopian-io |
json_metadata | {"app":"beem\/0.20.17"} |
created | 2019-04-02 01:46:09 |
last_update | 2019-04-02 01:46:09 |
depth | 1 |
children | 0 |
net_rshares | 0 |
last_payout | 2019-04-09 01:46:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 SBD |
curator_payout_value | 0.000 SBD |
pending_payout_value | 0.000 SBD |
promoted | 0.000 SBD |
body_length | 589 |
author_reputation | 152,913,012,544,965 |
root_title | "Build A Blog Site With React, Redux Firebase And MaterializeCSS (Part 8)" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 SBD |
percent_steem_dollars | 10,000 |