Nutbox 오픈 소스 툴킷은 다양한 Web 3.0 시나리오에 맞는 높은 유연성과 확장성을 제공합니다. Nutbox DAO는 DAO에 워드프레스 플러그인을 설치하는 것만큼이나 쉬운 자체 서비스를 구축할 수 있습니다. 대부분 스마트 컨트랙트의 형태로 구축된 툴킷과 서브프레임 프레임워크의 팔레트입니다. DAO는 자체 서비스를 쉽게 시작할 수 있도록 사용자를 설치하고 구성할 수 있습니다. 대표적인 툴킷으로는 NFT, Proof of Brain, Multi-round auction, 웹 2.0 통합, 공공재가 있습니다.
스테이킹 경제는 스테이킹을 통해 재정적으로 실현 가능한 DAO를 부트스트랩하는 패턴입니다. DAO 및 cToken에 대한 장기적인 가치를 창출하기 위해 커뮤니티 구축 툴킷과 같은 DAO서비스들 관점에서 DAO는 더 많은 가치들을 커뮤니티와 웹3.0생태계에 제공해야합니다.
--- It's a series of Redux. Hope you go through everything as Redux is not easy concept to understand if you are beginner and i am still beginner too but i have written this series to share my knowledge. Why Redux? I wrote the concept of Redux and why it has created by Redux team. Introducing MVC architecture MVC MVC is a design pattern. The client sends the request to the controller > update the model according to the action > pass it to the view When an action comes in, the controller receives and then update the data held by the model. After that, the changes are reflected in the view. However, as the application gets bigger and bigger, its hard to figure out which model and view are intertwined. The reason why we apply Flux over MVC? Facebook had reported the cause of the alarm bug in the message app with two-way data flow. So to fix it, introduced Flux with one-way data flow. What is Flux? Flex is kind of abstract concept. To overcome MVC limitation, Facebook created an application architecture. What is Redux? It's a JS library that implement the flux architecture created by Facebook. A state management system for cross components or app-wide state. Usage : Efficient data flow and state management between components One thing to keep in mind In order to update values immutably, your code must copy the existing objects as Redux expects that all state updates are immutably. dispatch an action and that action trigger a reducer Core redux concept * Redux uses a "one-way data flow" app structure When something happens in the app: The UI dispatches an action. The store runs the reducers, and the state is updated based on what occurred. The store notifies the UI that the state has changed. The UI re-renders based on the new state. Redux Terms and Concepts Action An action is a plan JS object with type filed. It always has a type. The rest is payload. const addTodoAction = { type: 'todos/todoAdded', payload: 'buy milk' // additional info } Action creators A function that creates and return an action object Reducers A reducer is a function that receives the current state and an action object. To decide how to update the state and return the new state: function counterReducer(state = initialState, action) {// Check to see if the reducer cares about this actionif (action.type === 'counter/increment') {// If so, make a copy of `state`return {...state,// and update the copy with the new valuevalue: state.value + 1}} store The current Redux state lives in store. The store is created by passing in a reducer. dispatch The only way to update the state in redux store is call "store.dispatch()" and pass in an action object store.dispatch({type: 'counter/increment'}) A example story You are at Domino Pizza shop! Hmm yummy! Customers are about to order a pizza with different toppings. All teams from management, chef, front cashier are need to know about that order data. Those data is stored in a central repository so it can be updated easily. { Type : "ORDER_PIZZA", payload : { Name: "Marie", Amount: "$30" } } 3 Action types : Put an order, Make a pizza or Cancel the order Redux cycle(Pizza store) Action Creator -> Action -> dispatch -> Reducers -> State Customer order -> Pizza -> Machine -> Departments -> All order status data. Redux Cycle create a store import { createStore } from "redux"; you can have only one store to manage the data. 2. dispatch the action Action is like what we want to do. Dispatch method send actions to the store. Actions (objects) MUST have TYPE property - what kind of action. Don't mutate the state - redux built on Immutability (copy) store.dispatch({ type: "ADD_TODO", text: toDo }); 2. Handle a reducer & connect it Update the new state. Reducer is like a function that used to update store and have 2 arguments which are (state, action). state - old state/state before update action - What happened/ what update Here's what a simple Reducer looks like: function reducer(state, action) { //return new state } To handle several action cases, we typically use switch statement below. By using a switch statement, you can handle different action types within your Reducer. 4. subscribe store.subscribe(paintTodos); You never mutate the state. but the only way to modify is to dispatch the action. Keep it mind that return new state objects, instead of mutating the previous state. never do like state.push(action.text), Do this by creating a new array return […state, {text: action.text}] The reason why we use filter() is that we should never ever mutate the array. filter() method creates a new array with all elements. --- React-redux The folder structure reducer, actionsand store Using React-redux, it provides Provider & Connect(). /actions -> Contains files to related to action creators /components -> Files related to components /reducers -> Files related to reducers /index.js -> set up both react and redux. Reducer is a pure function that changes the state. Whenever dispatch() method run, state re-updated. To handle these several reducers, we use combineReducers() of Redux package. To create a store, we use createStore() method. Store provide state down to child component() with Provider. subscribe() and dispatch() methods will be there to notice the changes. const render = () => { ReactDOM.render( document.getElementById('root') ) }; --- Redux toolkit Getting Started | Redux Toolkit The Redux Toolkit package is intended to be the standard way to write Redux logic.redux-toolkit.js.org --- Redux Middleware redux-saga & redux-thunk It was the most difficult part to understand for me. --- Redux with TypeScript Usage with TypeScript | React Redux React Redux itself is currently written in plain JavaScript. However, it works well with static type systems such as…react-redux.js.org --- Frequently asked questions The differences between Context API and Redux 1. Why Redux over React Context API? Although they have similar purpose, React Context has some potential disadvantages like complex setup with bunch of nested JSX Code and performance. One of Redux team members said its still not ready for all Flux-like state propagation(change frequently). React context is not optimized for high-frequency state changes. React-Redux uses the Context api, so you always are using both together. Redux is for state management, Context is for propagating values down the render tree. Not the same thing. --- Conclusion I highly recommend you to watch this to summary everything Reference Redux Fundamentals, Part 2: Concepts and Data Flow | Redux In Part 1: Redux Overview, we talked about what Redux is, why you might want to use it, and listed the other Redux…redux.js.org Understanding Redux: The World's Easiest Guide to Beginning Redux This is a comprehensive (but simplified) guide for absolute Redux beginners, or any who wants to re-evaluate their…www.freecodecamp.org Please subscribe my channel iDevBrandon Welcome to my vlog! I am Brandon. I'd share my life journey how i grow up as a developer & investorwww.youtube.com
<center><img src='https://www.weforum.org/weforum.jpg' alt='STEEM POSTS' /></center> ##### <p>Despite over 10,000 mental health apps on the market and a growing need, concerns about safety, efficacy, quality, and privacy continue to impede progress in this space</p><p>· A new resource,<i> </i><a href="https://www.weforum.org/whitepapers/global-governance-toolkit-for-digital-mental-health"><i>Global Governance Toolkit for Digital Mental Health</i></a>, provides help for governments, regulators and independent assurance bodies to address growing ethical concerns about the use of technology in mental and behavioural health</p><p>· Mental health and disruptive technology will be a key issue at the <a href="https://www.weforum.org/events/global-technology-governance-summit-2021/">Global Technology Governance Summit</a>, 6-7 April</p> ##### <br><center><a href='https://dlike.io/post/@martinedward/new-resource-for-protecting-personal-data-for-mental-health-apps'>Shared On DLIKE</a><hr><br><a href='https://dlike.io/'><img src='https://dlike.io/images/dlike-logo.jpg'></a></center>
# Brightly Interactive Light Toolkit for Creatives --- ## Screenshots <center><img alt="1.mp4" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2021-03-31/73a3567a-1-thumb.jpg"/></center> --- ## Hunter's comment Hi Dear Hunter, This is modular, interactive light toolkit and project-ready fixture collection made for everyone from casual light lovers to industry pros i am hunting today. 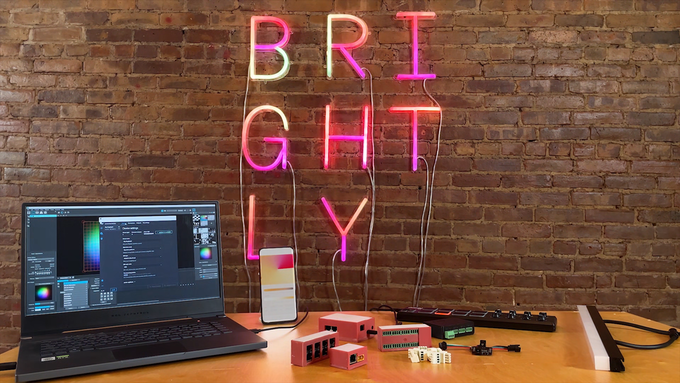 Creative work is now a matter of joke. You can make your own preferred lighting at your choice. Its top listed project of kickstarter. You can check this out. --- ## Link https://www.kickstarter.com/projects/glowbrightly/brightly-interactive-light-toolkit-for-creatives --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@engrsayful/brightly-interactive-light-toolkit-for-creatives) </center>
VSL Toolkit Pro Review Video clip has actually been substantial in the past numerous years, and also it is just getting bigger as well as more important in 2019 and beyond. There are all kinds of various kinds of video clips out there yet primary income for great deals of leading marketing experts and brands are Video Sales Letters (VSLs). Exactly how To Develop A Straightforward Video Sales Letter Script That Sells VSL Toolkit Pro can help your organisation boost the variety of leads or sales you obtain virtually overnight. In the direct mail days, "sales letters" were the supreme marketing tool to land clients fast. As technology has actually created, things have actually transformed, and also currently video sales letters have, in several ways, changed the traditional "sales letter." You can use a video sales letter (VSL) to market a product or a service, and this can be a great tool to bring in chilly website traffic that does not also realize they have a requirement for your service or product. The factor is that a video sales letter aims to initially assist the viewer recognize they have pain factors, and after that recommend them a service. The hardest part of the video clip sales letter creation process is scriptwriting. As soon as you have the script, it's very easy. All you have to do is read words, have some slides to present as well as videotape your display utilizing a screen-recording software application. This article will stroll you via creating a script that sells your services or product to cool customers who have no idea who you or your business are. Initially, start with an attention-grabbing statement. Beginning with a sentence that will get hold of the audience's focus as well as get them to focus on you. Commonly, this could be a vibrant case or assurance, and also you'll wish to call out your target audience. Describe the VSL Toolkit Pro trouble and why it's a huge one. In this sentence, you'll want to explain to your particular niche the trouble they have as well as why it's something they can not neglect. Discuss why the problem is even worse than they assume. This is where you can push the trouble much more as well as discuss the consequences of having this issue. You'll intend to explain how this trouble doesn't simply influence your visitor, however likewise the people they care about. This is a great method to make them recognize that this problem is even worse than they assumed. Make the problem struck them on an emotional level. You'll wish to discuss to them what's mosting likely to take place if they don't repair this problem now. At this moment in the VSL, the customer is totally aware they have an issue and now has the desire for a service. Introduce the option. This is when you reveal the customer what you've created to resolve their issue. Construct integrity with your customer. Now, the audience might be thrilled to find out about the option you have, but you need to discuss why you as well as your firm are qualified. Show them evidence. The following part of your manuscript is when you demonstrate and show to the VSL Toolkit Pro viewer that your remedy actually functions. I locate that the best method to do this is to show them testimonials and also case studies of your success stories. You can utilize statistics, screenshots of results or whatever you require to display what your firm has actually completed. Introducing VSL Toolkit Pro Supplier: Max Rylski Item: VSL Toolkit Pro Introduce Date: 2020-May-21 Release Time: 11:00 EDT Front-End Price: $37 Sales Web Page: https://www.socialleadfreak.com/vsl-toolkit-pro-review/ Particular niche: General What Is VSL Toolkit Pro? "VSLs" are like a text sales letter, but stretched out right into Video clip Style. Often with images and also other illustrations to make the factor much better. The reason why many leading online marketers make use of VSLs is they transform as well as they are much easier to develop than more "expensive" kinds of video clips like explainer, computer animations, and so on. I will suggest you the new tool to produce your own videos. It streamlines the whole sales video clip production procedure as quick as well as straightforward to use as possible. It's called VSL Toolkit Pro. VSL Toolkit Pro enables you to easily produce wonderful looking sales videos and VSLs. You simply have to copy & paste your text. With the Drag-n-Drop interface, you can additionally drag images, transparent PNGs and also videos inside the VSL. There're advanced features not found in other "VSL manufacturers" like the ability to place 3D animations and even eco-friendly display sales individuals into your VSLs. PRO You can create an excellent looking VSL actually in a couple of mins - just copy-n-paste your sales script into placeholders. You can produce a lot more complex sales videos with: pictures, videos, icons, clear PNGs, 2D-n-3D animations, as well as also green display sales individuals. You can Drag-n-Drop WHATEVER you see on the screen, you can easily move around, resize, turn, etc. You can develop all kinds of video types (not just VSLs) - Dish videos, instructional videos, video clip ads, slide shows, as well as extra. How Does VSL Toolkit Pro Work? It's very easy for you to create a Full VSL In 3 Easy Actions. Adhering to the 3 actions above, you can literally create a full sales video in a few minutes. Step 1: Select Scene (or empty canvas) You choose from lots of VSL design scenes like: message in middle, message with heading, headline & bullets. etc. Action 2: Copy-n-paste your message You open your video clip sales manuscript text file, as well as copy-n-paste your sales copy right into placeholders. Action 3: Put voiceover or music You drag-n-drop your pre-recorded voice over or background music. That's it! The video is currently ready to provide. Final thought "It's A Large amount. Should I Invest Today?" Not just are you obtaining access to VSL Toolkit Pro for the very best cost ever provided, but likewise You're investing totally without risk. VSL Toolkit Pro consists of a 30-day Refund Guarantee Plan. When you choose VSL Toolkit Pro, your satisfaction is assured. If you are not entirely satisfied with it for any kind of factor within the initial thirty day, you're qualified to a full reimbursement-- no question asked. You've got absolutely nothing to shed! What Are You Waiting on? Try It today and get The Complying with Perk Now! See More: https://sites.google.com/site/cotereview/vsl-toolkit-pro-review-should-i-grab-it
Ultimate Video Toolkit Review The core idea behind Ultimate Video Toolkit is to allow any person to produce After Effects high quality videos, by dragging as well as going down a few files, and by duplicating as well as pasting their text into placeholders. With Ultimate Video Toolkit people can produce practically ANY sort of video, without a pricey software application like After Effects, without previous video clip modifying understanding, and also actually within just a few mins. The Ultimate Overview to Easily Make Educational Videos (Part 5) Tip 5. Edit the video Most people feel like they need to be a professional video clip Ultimate Video Toolkit editor to make a good looking video. Yet you don't require pricey editing and enhancing tools or a lot of knowledge to get started. It takes just a few steps as well as some easy video modifying suggestions. To start, remove blunders by choosing them with the playhead, then clicking cut. To trim extra video from the ends or your recording, drag the end of the clip in. Editing Thorough|Camtasia|TechSmith When you complete modifying the video clip, include your audio narration. With your narration on the timeline, you can make use of clip rate as well as extend framework to sync the audio as well as video clip in your job. Surge Relocate & & Prolong Structure|Camtasia|TechSmith Below are a few instances of simple edits: If you require more time to explain a principle divided the clip and also use extend structure to basically ice up the video clip. To quicken a boring part of your recording, add clip rate, then drag the handles to speed it up. Or to concentrate your customer's interest, usage computer animations to zoom in on the vital parts. Step 6. Add a video clip introductory A video introduction leads your visitors into your content, however don't obtain also insane. Keep your introductory easy as well as to the point. Ultimate Video Toolkit wish to get to the meat of your material. They uncommitted about anything apart from what you assured to instruct them. An excellent intro plainly outlines the topic as well as quickly discusses what the audiences can anticipate to learn. To produce your very own video intro, include some area at the start of your video. Hold the change key on your key-board and also drag the playhead to the right. After that, open your media bin and also choose the Library tab. From the "Movement Graphics-- Intro Clips" folder, drag the intro you like onto the timeline. Camtasia comes equipped with a couple of integrated video introductory templates, yet you can get an entire catalog of pre-made video clip possessions, consisting of introductory templates from TechSmith Possessions. To customize your introductory clip, pick it on the timeline and then edit the text as well as forms in the residential or commercial properties panel. Enter message, pick a font, and alter any colors or various other setups for the shapes as well as message. After you have your video clip all created, now is a fun time to include some songs to your video. While it's not required, songs can make a great video clip that much better. For a how-to or video clip lesson, attempt to select something positive as well as favorable. You desire your customers to feel excellent as they're finding out. Step 7. Produce and also share Finally, think of where your video clip will certainly live. There are numerous video holding alternatives to choose from nowadays. You can share your video to an on the internet video system like YouTube, Vimeo, or Screencast, or you can pick to save the video as a neighborhood file. You can likewise share straight to Ultimate Video Toolkit. We've found that most of our individuals prefer to save finished videos on YouTube, especially for external videos. There are several fantastic factors to put your education as well as finding out videos on YouTube. We additionally have a cost-free guide, if you intend to learn our precise pointers as well as tricks on just how to make a YouTube video. Before sending your video clip out right into the globe, I advise sharing your video clip with a couple of people to obtain some video clip comments. This helps guarantee your message is clear as well as your video completes your goals. Ultimate Video Toolkit Review & Introduction Developer: Max Rylski Item: Ultimate Video Toolkit Date Of Release: 2020-Jan-27 Time Of Release: 11:00 EST FE Cost: $37 Sales Page: https://www.socialleadfreak.com/ultimate-video-toolkit-review/ Specific niche: Software program Ultimate Video Toolkit Testimonial - Features & Conveniences Pre-Made Storyboard Templates Consisted Of - Develop Multi-Scene Videos SUPER QUICKLY To make it SUPER EASY for you to create videos, I'm consisting of full multi-scene ready-to-go videos. All the web content is currently arranged nicely, and all you need to do is copy and also paste your message and also photos. You can essentially have a complete video created in under 5 minutes with these themes. 150+ Editable Scenes Included - Mix & Match Scenes To Create Your Very Own Storyboard Sequences There are over 150 specific scenes. Each scene is nicely formatted with message, images, animations, changes, and so on. You can take these scenes, and organize them in any order you want. Producing your own multi-scene videos. TRUE Drag & Decrease Editor Consisted Of - What ever you see on the screen, you can drag & drop anywhere Placement text where ever you desire, position images where ever before you desire, move anything you want anywhere on screen. Create your very own one-of-a-kind formats. Mix & Suit Aspects From Various Layouts - Incorporate genuine video videos, with animated text, with explainer components, with 3D computer animations, etc. 1,000+ Premium Video Clip & Graphics Properties Consisted Of With Ultimate Video Toolkit you can add your very own images, video clips and logos inside the design templates. That means you can create video clips for ANY specific niche subject. And to obtain you began I'm including 100's of assets like images, backgrounds, components, and more. Drag & Go down these assets right into the design templates OR import them right into any other video clip editor you already have. Cutout Individuals Photos These images have actually been professionally removed of their backgrounds, so they're extremely versatile. You can include them on top of any kind of background. HD Video Clips To get you began developing videos, I'm consisting of a collection of hand picked HD video. These videos are available in all type of various groups like: city, fitness, laptop, phone, crowd, nature, and so on. Ultimate Video Toolkit Develops Virtually Any type of Type Of Video clip, For Any Kind Of Particular niche Organisation Discount Videos Produce smooth, contemporary as well as clean looking organisation video clips. Include your own pictures (or video) right into placeholders, then duplicate & paste your message. It's that easy. 3D Animations Want to REALLY order your visitors focus? Include these eye-popping 3D animated scenes to your videos! Recipe Videos Transform your plain text dishes right into beautiful VIDEO CLIP recipes. Explainer Videos Develop prominent explainer design video clips with computer animated things as well as text. Travel Videos Show off your travel videos in vogue with these traveling design templates. Item Discount Videos Let people find out about your special sale with these item price cut video clips. Can be made use of for ANY sort of item, in any niche. Just change the placeholder photos with your very own. Fast Cutting Videos Want something upbeat? These quick cutting videos promptly get hold of interest, and also present your message in a fun, upbeat method. Stylish Video clips Want a slower, much more sophisticated video? We have themes for that too. These are excellent for style, elegance items, or anything else "elegant". 3D Laptop Computer Slideshows Put your videos inside a 3D computer animated laptop computer. Produce awesome looking 3D laptop computer slideshows. It's something distinct that looks cool and makes sure to order interest. Action Videos Transform any photos (or video) right into fast cutting action video clips. They feature motion picture shifts that whip, spin, and also zoom between scenes. Giving them that "action" feel. Occasion Promo Videos This is one more clean as well as streamlined template that can be used to advertise an occasion. OR can be utilized for any other coupon kind video clip (not just occasions). Physical fitness Videos These templates use STRONG shades, as well as fast motion to develop upbeat videos. Great for physical fitness and also sports. Picture Slider Videos Existing your pictures in style with these "image sliders". You can place the images anywhere you desire on the screen-- just drag as well as decrease. Fancy Logo Design Discloses Need a logo design stinger for your video introductory or outro? These elegant logo exposes are sure to thrill. You can add them in addition to any kind of shade background, any type of photo, and even on top of video clips! Easy Logo Exposes If you require an even more clean and also simple logo design stinger, these templates are for you. They function wonderful with social media sites symbols also, not just logos. So if you intend to advertise your social channel web link, these are fantastic for that. Conclusion "It's A Large amount. Should I Spend Today?" Not only are you obtaining access to Ultimate Video Toolkit for the very best price ever before offered, however likewise You're spending totally without danger. Ultimate Video Toolkit consists of a 30-day Cash Back Guarantee Plan. When you pick Ultimate Video Toolkit, your complete satisfaction is assured. If you are not totally satisfied with it for any type of factor within the very first thirty day, you're qualified to a full reimbursement-- no doubt asked. You've got nothing to lose! What Are You Awaiting? Try It today and obtain The Following Benefit Now! See More: https://www.pearltrees.com/tritapii/item284671141
# COACHING TOOLKIT FOR KAYAKING app designed for rowing enthusiasts --- ## Screenshots <center><img alt="styl.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-11-03/a30b7458-styl.jpg"/></center> | <center><img alt="kayak.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-11-03/4d4be93f-kayak.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-11-03/4d4be93f-kayak.jpg">View Image</a></center> | <center><img alt="dragon.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-11-03/88708ee5-dragon.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-11-03/88708ee5-dragon.jpg">View Image</a></center> | | - | - | | --- ## Hunter's comment ¨COACHING¨¨TOOLKIT¨ It is an app designed for enthusiasts or rowing athletes, ideal for different boats Ideal to improve the methods of rowing used in ¨boats¨, ¨kayaks¨ or ¨canoas¨ The app records all the important information of what happens while the athlete is exercising, ideal to maintain control and know the limits of the athlete. https://youtu.be/1sWrgnv_5nY Source: https://www.youtube.com/channel/UC9uUXejt7HNmYaNCR4hM-Ew/videos --- ## Link https://physiological-avatar.azurewebsites.net/?page=36 --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@jlufer/coaching-toolkit-for-kayaking-app-designed-for-rowing-enthusiasts) </center>
Hi. These days, I spent a lot of time doing a sort of combing. I migrated all the core modules to the ES6 folder in order to adapt to more project needs. :) Make over **120+** components to wear again and again! **Uix Kit is not a framework, just a UI toolkit based on some common libraries for building beautiful responsive website.** Uix Kit isn't a reusable component structure, mostly custom CSS and JavaScript based. Definitely interesting, and if you're developing mostly web content and not applications this is particularly useful. It can be used separately, or merge components and grid systems using bootstrap. Support JS, HTML and SASS component library automatically packaged. Automatically convert ES6 JS to ES5 using Babel in this scaffold. Project URL: https://github.com/xizon/uix-kit Preview: https://xizon.github.io/uix-kit/examples/ GitHub pages can only serve static content, and there is no way to run PHP or get Ajax request on the pages. You need to visit the link below to see some special demos https://uiux.cc/uix-kit
# Fotophire Edit crop photos or remove unwanted objects easily --- ## Screenshots <center><img alt="Wondershare-Fotophire-Review.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-06-06/b73fae85-Wondershare-Fotophire-Review.jpg"/></center> --- ## Hunter's comment An easy to use toolkit by wondershare, you can edit photos, erase some parts of it and even cut and crop. You can remove backgrounds or replace them with other images. There are over 200 effects you can apply and make your pictures look better. It works on both Windows and MacOS. --- ## Link https://photo.wondershare.com/photo-editing-toolkit.html --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@aamirijaz/fotophire-edit-crop-photos-or-remove-unwanted-objects-easily) </center>
<p><strong>Introduction of Crimp Tools:</strong></p> <p><a href="https://www.crimpingtools.com/collections/cable-tool"><strong>Crimp tools</strong></a> are used to terminate useless wires. There are many advantages of using crimping tools over wire wrapping and soldering. Such as, if crimping tools is made very professionally without any faults and are made air tight, then oxygen and moisture do not reach the metal and do not corrode the metal. Since no alloys are used, the joints that are made are mechanically very strong. Only wire wrap can be used with small cross-sectional area wires, whereas for both large and small cross-sectional area wires, crimped connections are used.</p> <p>https://cdn.steemitimages.com/DQmXmadq3GPYBgiCz1NiJwVZNELNTMRcq2LjdR4rcLczkUL/crimp%20tools.png</p> <p><strong>Making</strong>:</p> <p>As crimping is very useful, the steps involved in it are, firstly, the terminal is made to fit in the crimping tool and then the wire is made to go inside the terminal. Lastly, the crimping tool's handles are squeezed, reshaping and compressing the terminal till it is welded onto the wire. The connection that is obtained eventually may appear to be loose at the terminal edges but it is made so because these prevent the wires with outer strands from any cuts. If made properly, the crimp's middle is cold formed.</p> <p><strong>Types</strong>:</p> <p>There are a wide variety of crimping tools which are designed for specific sizes and types of terminals. Some of them include Klein modular plug, Klein journeyman crimper. There are two main types or classes of crimped terminals which are barrel connectors and open barrel terminals, the former has a cylindrical opening for wire. Crimped tools are very beneficial.</p> <p><strong>Introduction of Electric Strapping Tools:</strong></p> <p>Strapping is also named as banding and bundling. It is the process of attaching a strap to a thing or an item to fasten, combine etc. Strapping tools (machinery) are also named as bundlers which are useful for bigger volume applications. The major applications of strapping include binding items together, reinforcing boxes or crates, attaching items and securing loads.</p> <p><strong>Orientation</strong>:</p> <p>They are available in vertical and horizontal orientations. The most common one is the vertical orientation. They are also available in top seal, side seal and bottom seal orientation, which shows where the joint has been done and where it is in the bundle. They are even available in automatic and semi-automatic varieties. The former one can be used with conveyor systems.</p> <p><strong>Types and their Working</strong>:</p> <p>Automatic strapping machine has the availability of hands free, which is very good in securing of items. In the semi-automatic strapping machine, there is one operator which handles the seal, cut and tension of polypropylene strapping on any box of any size. Fully automatic and semi-automatic strapping machine are both included in the packaging line production. These <a href="https://www.crimpingtools.com/collections/strap-tools"><strong>electric strapping tools</strong></a> improve the efficiency in a factory or warehouse plus they even manage to help you give a neat and secured packaged product which is really presentable. Since the straps are adjustable, if a box is comparatively softer than other boxes, then there is very less chance of it getting damaged. It is widely used in attaching items to skids, crates, pallets, flatbed and flatcars semi trailers etc.</p> <p> </p> <p> </p> <p> </p>
# Encore Share and synchronize data --- ## Screenshots <center><img alt="4.JPG" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/d442a671-4.JPG"/></center> | <center><img alt="3.JPG" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/0a20cc64-3.JPG"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/0a20cc64-3.JPG">View Image</a></center> | <center><img alt="2.JPG" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/ca944f69-2.JPG"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/ca944f69-2.JPG">View Image</a></center> | <center><img alt="1.JPG" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/2ba4fa41-1.JPG"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-05-04/2ba4fa41-1.JPG">View Image</a></center> | | - | - | - | | --- ## Hunter's comment An Artificial Intelligence based database that is used for management of data from any source. No matter if it is run in any of the marketplaces, online shop or any mobile application. So it offers a toolkit to manage the entire technology. --- ## Link https://encore.io --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@enisshkurti/encore-share-and-synchronize-data) </center>
# prodMMD product manager toolkit --- ## Screenshots <center><img alt="ProdMMD_PublicDash.png" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-03-05/0cf2dd44-ProdMMD_PublicDash.png"/></center> --- ## Hunter's comment > Software your clients appreciate, your company stakeholders desire, and your product team loves. this might be useful for people making dApps on the steem blockchain, you could reward people for doing a variety of functions on your projects and have management of chain about it, even get people to report onchain of progress? :) --- ## Link https://prodmmd.com --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@teamhumble/prodmmd-product-manager-toolkit) </center>
[엔비디아 개발자 다운로드 센터] https://developer.nvidia.com/accelerated-computing-toolkit 위 링크에 들어가 쿠다 툴킷을 받고 우분투에 설치합니다. 우분투 버전 18.04, 쿠다 버전 9.2 및 신규 패치(Patch 1 - Released May 16, 2018) 적용입니다. 받은 툴킷에 그래픽 드라이버 및 openGL 라이브러리 등등이 포함입니다. 편하게 설치 진행 하시면 됩니다. 아! 설치전에 필수로 gcc 와 g++을 설치합니다. $ sudo apt-get install gcc g++ 먼저 기존에 설치되어 있는 드라이버가 있다면... $ sudo apt-get purge nvidia* $ sudo apt-get autoremove $ sudo apt-get autoclean $ sudo rm -rf /usr/local/cuda* 위와 같이 진행하구요. 만약 우분투를 GUI 로 사용하시고 계신다면 실행하세요. $ sudo service lightdm stop $ sudo service mdm stop $ sudo service gdm stop 이제 다운받은 것을 실행합니다. 그래픽 드라이버 포함이기 때문에 설치관련 질문확인하세요. $sudo sh cuda_당신의.버전.입니다_linux.run 이제 설치과 완료 되었다면 ~/.bashrc 에 환경 변수를 두가지 추가 해줍니다. $ sudo export PATH=$PATH:/usr/local/cuda-8.0 $ sudo export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:/usr/local/cuda-8.0/lib64 위 설치 과정을 무사히 완료했다면 아래의 명령어로 확인합니다. $ nvcc -V 당신의... 어쩌구... 쿠다 툴... $ nvidia-smi 모든 것이 완료했습니다! 만약에! 원인 모를 충돌이 일어난다면... 아래의 링크를 통해 한번 꼭 확인 하시기 바랍니다! [우분투(ubuntu) watchdog bug soft lockup 문제 발생시 임시 해결법] https://pilgoo.tistory.com/350
$297 Linkgage + Botsend Combo PRO Lifetime Subscription Promo It is a rare chance to have both Linkgage+Botsend lifetime membership Pro or Business package. The Linkgage provider Offers this combo for PRO package is $297 and Business package is $497. You have only 10 days to buy it and get the lifetime account. Promo link: https://couponrim.com/297-linkgage-botsend-combo-pro-lifetime-subscription-promo/ 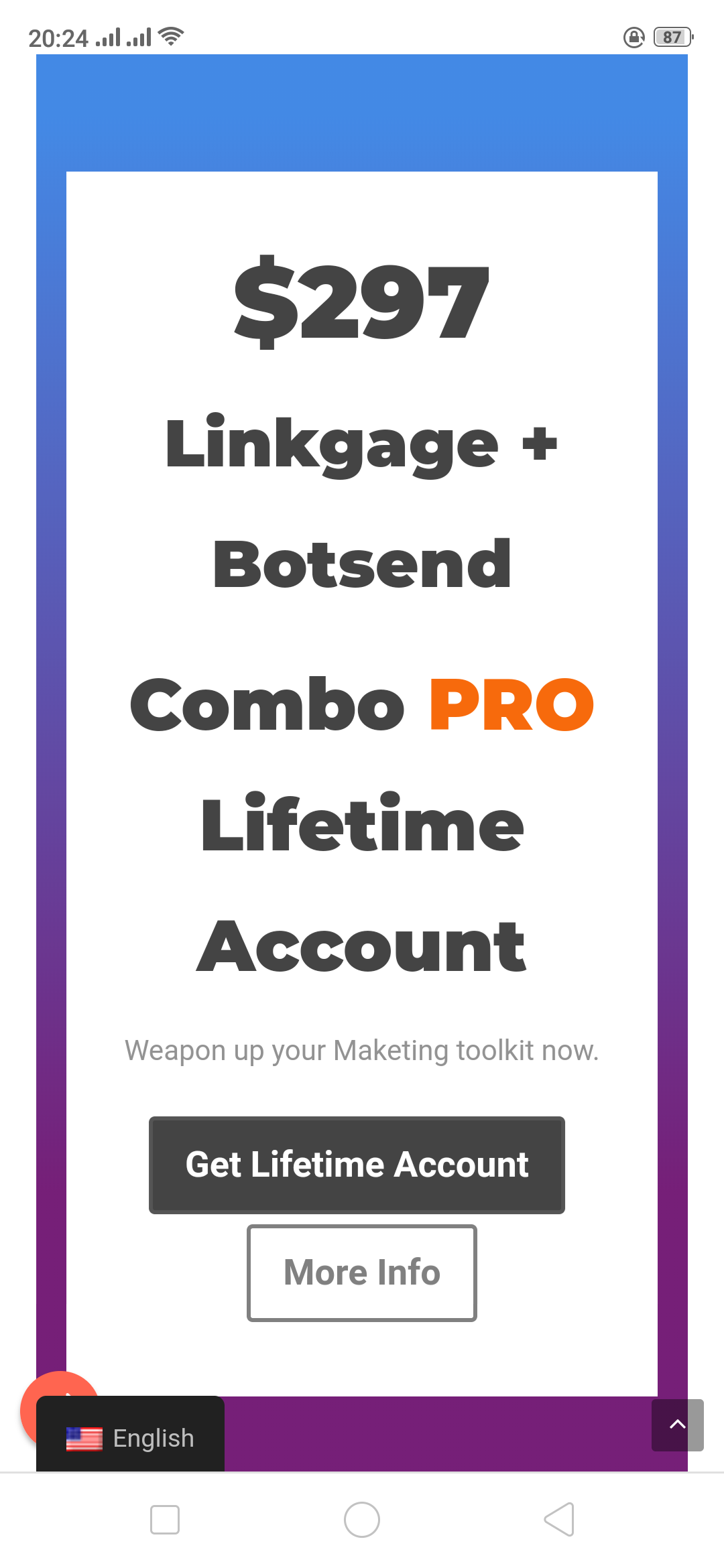
# 2 hector The connected weather station toolkit --- ## Screenshots <center><img alt="3770006418013_p0-900x569.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/d5390bc4-3770006418013_p0-900x569.jpg"/></center> | <center><img alt="Hector-table.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/002549be-Hector-table.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/002549be-Hector-table.jpg">View Image</a></center> | <center><img alt="téléchargement.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/052f4253-téléchargement.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/052f4253-téléchargement.jpg">View Image</a></center> | <center><img alt="0552ba52658f5f697aa762a51e335ce7_original.jpg" src="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/6dd5e117-0552ba52658f5f697aa762a51e335ce7_original.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/steemhunt/production/steemhunt/2019-01-21/6dd5e117-0552ba52658f5f697aa762a51e335ce7_original.jpg">View Image</a></center> | | - | - | - | | --- ## Hunter's comment Hector serves as a thermometer and hygrometer. Its mobile application, available on Android and Apple offers a multitude of small functions. The Hector cube can memorize how its environment evolves and alert you if there is too much change. Its historical function makes it possible to consult the humidity rate as well as the temperature variations over the last 20 days. Its real advantage lies in its versatility. On the company's website, it is as much associated with the monitoring of the weather, the fridge connected or the humidity of luggage during a trip. However, in the case of weather data, it is necessary to wait 24 hours to receive forecasts. What discourage fans of data in real time. --- ## Link https://www.misterhector.com/en/produit/10244/ --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@kouba01/2-hector-the-connected-weather-station-toolkit) </center>
# Pro Tech Toolkit Pro Tech Toolkit + Magnetic Project Mat Bundle --- ## Screenshots <center><img alt="IMG_20181129_000459_615.jpg" src="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/41dbd902-IMG_20181129_000459_615.jpg"/></center> | <center><img alt="IMG_20181129_000447_637.jpg" src="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/90bca347-IMG_20181129_000447_637.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/90bca347-IMG_20181129_000447_637.jpg">View Image</a></center> | <center><img alt="IMG_20181129_000435_116.jpg" src="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/513f2e77-IMG_20181129_000435_116.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/513f2e77-IMG_20181129_000435_116.jpg">View Image</a></center> | <center><img alt="IMG_20181129_000424_162.jpg" src="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/d94188a5-IMG_20181129_000424_162.jpg"/><br><a href="https://s3-us-west-2.amazonaws.com/huntimages/production/steemhunt/2018-11-29/d94188a5-IMG_20181129_000424_162.jpg">View Image</a></center> | | - | - | - | | --- ## Hunter's comment You can repair almost everything with this tech toolkit whether it's Apple product or a gaming console. This bundle includes most useful tools for repairing every tech product. Pro Tech Toolkit is magnetic therefore you won't lose small details of your gadgets. In my opinion this toolkit can be ideal for tech repair centers. --- ## Link https://www.ifixit.com/Store/Tools/Pro-Tech-Toolkit-Magnetic-Project-Mat-Bundle/IF145-444-1 --- ## Contributors Hunter: @alan369 --- <center><br/><br/> This is posted on Steemhunt - A place where you can dig products and earn STEEM. [View on Steemhunt.com](https://steemhunt.com/@alan369/pro-tech-toolkit-pro-tech-toolkit-magnetic-project-mat-bundle) </center>
Hi team, I am trying to give my votes for a proxy on genereos, so I set my voting power lower on eosportal to give more votes to the proxy. The problem, is i don't really understand this, and when i click on the proxy list it sends an error. Where can I learn the basics of what i can do with my eos? Also, not sure how to create an identity in the toolkit. Is it needed?
The Complete Empath Toolkit PDF Download - https://tinyurl.com/yc8d4oty When thinking of starting a company, folks think about goods that sell quick, like food. Even if there are a lot of foods corporations available, a whole new meals industry is what people usually imagine the industry is hungry for. However, if you look at the recent trend in terms of people's way of life, you'll realize that an enormous portion of free time of men and women, specially older people, is invested browsing the world wide web. Even though they may be seeking new meals spots to examine many of the time, they're spending a lot of their time on-line giving their cravings for food for info structured merchandise. Info dependent products appear in different forms -- e books, video clips, Music, podcasts, cellphone and pc software programs, computer software, and the like. People are not just purchasing the latest track from the most used groups and songstresses, or the latest motion picture introduced in virtual form. The truth is, people take a look at a range of facts structured merchandise on the web, since the majority with their requires for job or play is often resolved by the products.
eosDAC vừa giới thiệu về Công Cụ Thành Viên eosDAC demo với các tính năng mới. So với phiên bản hiện tại trên members.eosdac.io thì bản demo này sẽ thêm chức năng Bầu Người Giám Hộ, Quản Lý CPU và Băng Thông (Bandwidth), Mua/Bán Ram, Sổ Địa Chỉ và nhiều tính năng khác chờ bạn khám phá. Video khám phá các tính năng https://www.youtube.com/watch?v=G6t6HPyXp7s Nếu bạn có tài khoản trên mạng thử nghiệm EOS Jungle, bạn có thể thử tương tác với công cụ demo này tại http://members-dev.eosdac.io/ Xem Video dưới đây hướng dẫn về cách tạo tài khoản trên mạng thử nghiệm Jungle https://drive.google.com/open?id=1SB8SL1pWJklTIgD5ihawWs60-xzvOoZ3 Sau đây là tất cả thông tin mà bạn sẽ cần khi test công cụ này và kết nối đến tài khoản mạng thử nghiệm Jungle bằng Scatter. * <b>Domain:</b> dev.cryptolions.io * <b>Port:</b> 38888 * <b>URL công cụ demo:</b> http://members-dev.eosdac.io/ * <b>Testnet Chain Id:</b> 038f4b0fc8ff18a4f0842a8f0564611f6e96e8535901dd45e43ac8691a1c4dca Website: https://eosdac.io Steemit: https://steemit.com/@eosdacvietnam Telegram channel: https://t.me/eosdacvietnam Telegram chat group: https://t.me/eosdacvietnam_chat Twitter: https://twitter.com/eosdacvietnam Medium: https://medium.com/@eosdacvietnam Facebook: https://facebook.com/eosdacvietnam